mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 11:16:51 +00:00
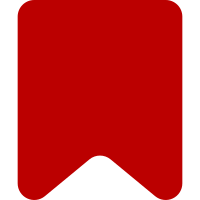
* For the most common case: - replace ve.extendClass with ve.inheritClass (chose slightly different names to detect usage of the old/new one, and I like 'inherit' better). - move it up to below the constructor, see doc block for why. * Cases where more than 2 arguments were passed to ve.extendClass are handled differently depending on the case. In case of a longer inheritance tree, the other arguments could be omitted (like in "ve.ce.FooBar, ve.FooBar, ve.Bar". ve.ce.FooBar only needs to inherit from ve.FooBar, because ve.ce.FooBar inherits from ve.Bar). In the case of where it previously had two mixins with ve.extendClass(), either one becomes inheritClass and one a mixin, both to mixinClass(). No visible changes should come from this commit as the instances still all have the same visible properties in the end. No more or less than before. * Misc.: - Be consistent in calling parent constructors in the same order as the inheritance. - Add missing @extends and @param documentation. - Replace invalid {Integer} type hint with {Number}. - Consistent doc comments order: @class, @abstract, @constructor, @extends, @params. - Fix indentation errors A fairly common mistake was a superfluous space before the identifier on the assignment line directly below the documentation comment. $ ack "^ [^*]" --js modules/ve - Typo "Inhertiance" -> "Inheritance". - Replacing the other confusing comment "Inheritance" (inside the constructor) with "Parent constructor". - Add missing @abstract for ve.ui.Tool. - Corrected ve.FormatDropdownTool to ve.ui.FormatDropdownTool.js - Add function names to all @constructor functions. Now that we have inheritance it is important and useful to have these functions not be anonymous. Example of debug shot: http://cl.ly/image/1j3c160w3D45 Makes the difference between < documentNode; > ve_dm_DocumentNode ... : ve_dm_BranchNode ... : ve_dm_Node ... : ve_dm_Node ... : Object ... without names (current situation): < documentNode; > Object ... : Object ... : Object ... : Object ... : Object ... though before this commit, it really looks like this (flattened since ve.extendClass really did a mixin): < documentNode; > Object ... ... ... Pattern in Sublime (case-sensitive) to find nameless constructor functions: "^ve\..*\.([A-Z])([^\.]+) = function \(" Change-Id: Iab763954fb8cf375900d7a9a92dec1c755d5407e
270 lines
6.5 KiB
JavaScript
270 lines
6.5 KiB
JavaScript
/**
|
|
* VisualEditor data model Node class.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Generic DataModel node.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
* @constructor
|
|
* @extends {ve.Node}
|
|
* @param {String} type Symbolic name of node type
|
|
* @param {Number} [length] Length of content data in document
|
|
* @param {Object} [attributes] Reference to map of attribute key/value pairs
|
|
* @param {Object} [internal] Object with internal data to store for this node
|
|
*/
|
|
ve.dm.Node = function ve_dm_Node( type, length, attributes, internal ) {
|
|
// Parent constructor
|
|
ve.Node.call( this, type );
|
|
|
|
// Properties
|
|
this.length = length || 0;
|
|
this.attributes = attributes || {};
|
|
this.internal = internal || {};
|
|
this.doc = undefined;
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.dm.Node, ve.Node );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Gets a list of allowed child node types.
|
|
*
|
|
* @method
|
|
* @returns {String[]|null} List of node types allowed as children or null if any type is allowed
|
|
*/
|
|
ve.dm.Node.prototype.getChildNodeTypes = function () {
|
|
return ve.dm.nodeFactory.getChildNodeTypes( this.type );
|
|
};
|
|
|
|
/**
|
|
* Gets a list of allowed parent node types.
|
|
*
|
|
* @method
|
|
* @returns {String[]|null} List of node types allowed as parents or null if any type is allowed
|
|
*/
|
|
ve.dm.Node.prototype.getParentNodeTypes = function () {
|
|
return ve.dm.nodeFactory.getParentNodeTypes( this.type );
|
|
};
|
|
|
|
/**
|
|
* Checks if this node can have child nodes.
|
|
*
|
|
* @method
|
|
* @returns {Boolean} Node can have children
|
|
*/
|
|
ve.dm.Node.prototype.canHaveChildren = function () {
|
|
return ve.dm.nodeFactory.canNodeHaveChildren( this.type );
|
|
};
|
|
|
|
/**
|
|
* Checks if this node can have child nodes which can also have child nodes.
|
|
*
|
|
* @method
|
|
* @returns {Boolean} Node can have grandchildren
|
|
*/
|
|
ve.dm.Node.prototype.canHaveGrandchildren = function () {
|
|
return ve.dm.nodeFactory.canNodeHaveGrandchildren( this.type );
|
|
};
|
|
|
|
/**
|
|
* Checks if this node represents a wrapped element in the linear model.
|
|
*
|
|
* @method
|
|
* @returns {Boolean} Node represents a wrapped element
|
|
*/
|
|
ve.dm.Node.prototype.isWrapped = function () {
|
|
return ve.dm.nodeFactory.isNodeWrapped( this.type );
|
|
};
|
|
|
|
/**
|
|
* Checks if this node can contain content.
|
|
*
|
|
* @method
|
|
* @returns {Boolean} Node can contain content
|
|
*/
|
|
ve.dm.Node.prototype.canContainContent = function () {
|
|
return ve.dm.nodeFactory.canNodeContainContent( this.type );
|
|
};
|
|
|
|
/**
|
|
* Checks if this node is content.
|
|
*
|
|
* @method
|
|
* @returns {Boolean} Node is content
|
|
*/
|
|
ve.dm.Node.prototype.isContent = function () {
|
|
return ve.dm.nodeFactory.isNodeContent( this.type );
|
|
};
|
|
|
|
/**
|
|
* Gets the inner length.
|
|
*
|
|
* @method
|
|
* @returns {Number} Length of the node's contents
|
|
*/
|
|
ve.dm.Node.prototype.getLength = function () {
|
|
return this.length;
|
|
};
|
|
|
|
/**
|
|
* Gets the outer length, including any opening/closing elements.
|
|
*
|
|
* @method
|
|
* @returns {Number} Length of the entire node
|
|
*/
|
|
ve.dm.Node.prototype.getOuterLength = function () {
|
|
return this.length + ( this.isWrapped() ? 2 : 0 );
|
|
};
|
|
|
|
/**
|
|
* Gets the range inside the node.
|
|
*
|
|
* @method
|
|
* @returns {ve.Range} Inner node range
|
|
*/
|
|
ve.dm.Node.prototype.getRange = function () {
|
|
var offset = this.getOffset();
|
|
if ( this.isWrapped() ) {
|
|
offset++;
|
|
}
|
|
return new ve.Range( offset, offset + this.length );
|
|
};
|
|
|
|
/**
|
|
* Gets the range outside the node.
|
|
*
|
|
* @method
|
|
* @returns {ve.Range} Outer node range
|
|
*/
|
|
ve.dm.Node.prototype.getOuterRange = function () {
|
|
var offset = this.getOffset();
|
|
return new ve.Range( offset, offset + this.getOuterLength() );
|
|
};
|
|
|
|
/**
|
|
* Sets the inner length.
|
|
*
|
|
* @method
|
|
* @param {Number} length Length of content
|
|
* @throws Invalid content length error if length is less than 0
|
|
* @emits lengthChange (diff)
|
|
* @emits update
|
|
*/
|
|
ve.dm.Node.prototype.setLength = function ( length ) {
|
|
if ( length < 0 ) {
|
|
throw new Error( 'Length cannot be negative' );
|
|
}
|
|
// Compute length adjustment from old length
|
|
var diff = length - this.length;
|
|
// Set new length
|
|
this.length = length;
|
|
// Adjust the parent's length
|
|
if ( this.parent ) {
|
|
this.parent.adjustLength( diff );
|
|
}
|
|
// Emit events
|
|
this.emit( 'lengthChange', diff );
|
|
this.emit( 'update' );
|
|
};
|
|
|
|
/**
|
|
* Adjust the length.
|
|
*
|
|
* @method
|
|
* @param {Number} adjustment Amount to adjust length by
|
|
* @throws Invalid adjustment error if resulting length is less than 0
|
|
* @emits lengthChange (diff)
|
|
* @emits update
|
|
*/
|
|
ve.dm.Node.prototype.adjustLength = function ( adjustment ) {
|
|
this.setLength( this.length + adjustment );
|
|
};
|
|
|
|
/**
|
|
* Gets the offset of this node within the document.
|
|
*
|
|
* If this node has no parent than the result will always be 0.
|
|
*
|
|
* @method
|
|
* @returns {Number} Offset of node
|
|
*/
|
|
ve.dm.Node.prototype.getOffset = function () {
|
|
return this.root === this ? 0 : this.root.getOffsetFromNode( this );
|
|
};
|
|
|
|
/**
|
|
* Gets an element attribute value.
|
|
*
|
|
* @method
|
|
* @returns {Mixed} Value of attribute, or undefined if no such attribute exists
|
|
*/
|
|
ve.dm.Node.prototype.getAttribute = function ( key ) {
|
|
return this.attributes[key];
|
|
};
|
|
|
|
/**
|
|
* Gets a reference to this node's attributes object
|
|
*
|
|
* @method
|
|
* @returns {Object} Attributes object (by reference)
|
|
*/
|
|
ve.dm.Node.prototype.getAttributes = function () {
|
|
return this.attributes;
|
|
};
|
|
|
|
/**
|
|
* Get a clone of the linear model element for this node. The attributes object is deep-copied.
|
|
*
|
|
* @returns {Object} Element object with 'type' and (optionally) 'attributes' fields
|
|
*/
|
|
ve.dm.Node.prototype.getClonedElement = function () {
|
|
var retval = { 'type': this.type };
|
|
if ( !ve.isEmptyObject( this.attributes ) ) {
|
|
retval.attributes = ve.copyObject( this.attributes );
|
|
}
|
|
if ( !ve.isEmptyObject( this.internal ) ) {
|
|
retval.internal = ve.copyObject( this.internal );
|
|
}
|
|
return retval;
|
|
};
|
|
|
|
/**
|
|
* Checks if this node can be merged with another.
|
|
*
|
|
* For two nodes to be mergeable, this node and the given node must either be the same node or:
|
|
* - Have the same type
|
|
* - Have the same depth
|
|
* - Have similar ancestory (each node upstream must have the same type)
|
|
*
|
|
* @method
|
|
* @param {ve.dm.Node} node Node to consider merging with
|
|
* @returns {Boolean} Nodes can be merged
|
|
*/
|
|
ve.dm.Node.prototype.canBeMergedWith = function ( node ) {
|
|
var n1 = this,
|
|
n2 = node;
|
|
// Move up from n1 and n2 simultaneously until we find a common ancestor
|
|
while ( n1 !== n2 ) {
|
|
if (
|
|
// Check if we have reached a root (means there's no common ancestor or unequal depth)
|
|
( n1 === null || n2 === null ) ||
|
|
// Ensure that types match
|
|
n1.getType() !== n2.getType()
|
|
) {
|
|
return false;
|
|
}
|
|
// Move up
|
|
n1 = n1.getParent();
|
|
n2 = n2.getParent();
|
|
}
|
|
return true;
|
|
};
|