mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-16 02:51:50 +00:00
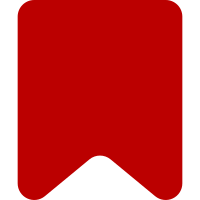
What this changes: * The moment the user selects anything in the parameter search widget, the input is cleared, no matter what happens next. Even in case of an error. We know the input was bad in this case. Let's get rid of it. * The method makes sure it does not even try to add a duplicate parameter. This should be unreachable, but better be safe than sorry. This is split from I5eeb973. I run into this while playing around with different approaches related to hiding deprecated parameters. Typically there should be no way the parameter search widget offers a duplicate. Still I believe it's a good idea to have this extra safety-net. Bug: T272487 Bug: T288827 Change-Id: I04e76d73b4a3f6467d0ccf3ccff5d2f6b4114bd9
127 lines
3.7 KiB
JavaScript
127 lines
3.7 KiB
JavaScript
/*!
|
|
* VisualEditor user interface MWParameterPlaceholderPage class.
|
|
*
|
|
* @copyright 2011-2020 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* MediaWiki transclusion dialog parameter placeholder page.
|
|
*
|
|
* @class
|
|
* @extends OO.ui.PageLayout
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.MWParameterModel} parameter Reference to a placeholder parameter with an empty
|
|
* name, as well as to the template the parameter belongs to
|
|
* @param {string} name Unique symbolic name of page
|
|
* @param {Object} [config] Configuration options
|
|
* @cfg {jQuery} [$overlay] Overlay to render dropdowns in
|
|
*/
|
|
ve.ui.MWParameterPlaceholderPage = function VeUiMWParameterPlaceholderPage( parameter, name, config ) {
|
|
var veConfig = mw.config.get( 'wgVisualEditorConfig' );
|
|
|
|
// Configuration initialization
|
|
config = ve.extendObject( {
|
|
scrollable: false
|
|
}, config );
|
|
|
|
// Parent constructor
|
|
ve.ui.MWParameterPlaceholderPage.super.call( this, name, config );
|
|
|
|
// Properties
|
|
// TODO: the unique `name` seems to be a relic of when BookletLayout held
|
|
// the parameters in separate pages rather than in a StackLayout.
|
|
this.name = name;
|
|
this.parameter = parameter;
|
|
this.template = this.parameter.getTemplate();
|
|
this.addParameterSearch = new ve.ui.MWParameterSearchWidget( this.template, {
|
|
showAll: !!config.expandedParamList
|
|
} )
|
|
.connect( this, {
|
|
choose: 'onParameterChoose',
|
|
showAll: 'onParameterShowAll'
|
|
} );
|
|
|
|
this.addParameterFieldset = new OO.ui.FieldsetLayout( {
|
|
label: ve.msg( 'visualeditor-dialog-transclusion-add-param' ),
|
|
icon: 'parameter',
|
|
classes: [ 've-ui-mwTransclusionDialog-addParameterFieldset' ],
|
|
$content: this.addParameterSearch.$element
|
|
} );
|
|
|
|
this.addParameterFieldset.$element.attr( 'aria-label', ve.msg( 'visualeditor-dialog-transclusion-add-param' ) );
|
|
|
|
// Initialization
|
|
this.$element
|
|
.addClass( 've-ui-mwParameterPlaceholderPage' )
|
|
.append( this.addParameterFieldset.$element );
|
|
|
|
if ( !veConfig.transclusionDialogNewSidebar ) {
|
|
var removeButton = new OO.ui.ButtonWidget( {
|
|
framed: false,
|
|
icon: 'trash',
|
|
title: ve.msg( 'visualeditor-dialog-transclusion-remove-param' ),
|
|
flags: [ 'destructive' ],
|
|
classes: [ 've-ui-mwTransclusionDialog-removeButton' ]
|
|
} )
|
|
.connect( this, { click: 'onRemoveButtonClick' } );
|
|
|
|
this.$element.append( removeButton.$element );
|
|
}
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWParameterPlaceholderPage, OO.ui.PageLayout );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Respond to the parameter search widget showAll event
|
|
*
|
|
* @fires showAll
|
|
*/
|
|
ve.ui.MWParameterPlaceholderPage.prototype.onParameterShowAll = function () {
|
|
this.emit( 'showAll', this.name );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWParameterPlaceholderPage.prototype.setOutlineItem = function () {
|
|
// Parent method
|
|
ve.ui.MWParameterPlaceholderPage.super.prototype.setOutlineItem.apply( this, arguments );
|
|
|
|
if ( this.outlineItem ) {
|
|
this.outlineItem
|
|
.setIcon( 'parameter' )
|
|
.setMovable( false )
|
|
.setRemovable( true )
|
|
.setLevel( 1 )
|
|
.setFlags( [ 'placeholder' ] )
|
|
.setLabel( ve.msg( 'visualeditor-dialog-transclusion-add-param' ) );
|
|
}
|
|
};
|
|
|
|
ve.ui.MWParameterPlaceholderPage.prototype.onParameterChoose = function ( name ) {
|
|
this.addParameterSearch.query.setValue( '' );
|
|
|
|
if ( !name || this.template.hasParameter( name ) ) {
|
|
return;
|
|
}
|
|
|
|
// Note that every parameter is known after it is added
|
|
var knownBefore = this.template.getSpec().isKnownParameterOrAlias( name );
|
|
|
|
this.template.addParameter( new ve.dm.MWParameterModel( this.template, name ) );
|
|
|
|
ve.track( 'activity.transclusion', {
|
|
action: knownBefore ? 'add-known-parameter' : 'add-unknown-parameter'
|
|
} );
|
|
};
|
|
|
|
ve.ui.MWParameterPlaceholderPage.prototype.onRemoveButtonClick = function () {
|
|
this.parameter.remove();
|
|
};
|