mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-25 14:56:20 +00:00
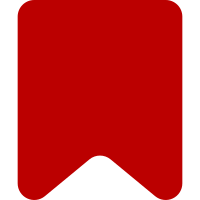
In general, the direction of the MWExtensionInspector textarea should be dependent on the directionality of the node it is editing. The only exceptions are <hiero> and <math> that need to have their textarea LTR always; these two inspectors' directionality definition is overridden in their onOpen() method. Bug: 56779 Change-Id: Iac5c1c3bf2c61b9fa36c9588c1734c91ca4305c4
126 lines
3.1 KiB
JavaScript
126 lines
3.1 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface MWExtensionInspector class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* MediaWiki extension inspector.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
* @extends ve.ui.SurfaceInspector
|
|
*
|
|
* @constructor
|
|
* @param {ve.ui.SurfaceWindowSet} windowSet Window set this inspector is part of
|
|
* @param {Object} [config] Configuration options
|
|
*/
|
|
ve.ui.MWExtensionInspector = function VeUiMWExtensionInspector( windowSet, config ) {
|
|
// Parent constructor
|
|
ve.ui.SurfaceInspector.call( this, windowSet, config );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWExtensionInspector, ve.ui.SurfaceInspector );
|
|
|
|
/* Static properties */
|
|
|
|
ve.ui.MWExtensionInspector.static.nodeView = null;
|
|
|
|
ve.ui.MWExtensionInspector.static.nodeModel = null;
|
|
|
|
ve.ui.MWExtensionInspector.static.removeable = false;
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Handle frame ready events.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.MWExtensionInspector.prototype.initialize = function () {
|
|
// Parent method
|
|
ve.ui.SurfaceInspector.prototype.initialize.call( this );
|
|
|
|
this.input = new OO.ui.TextInputWidget( {
|
|
'$': this.$,
|
|
'overlay': this.surface.$localOverlay,
|
|
'multiline': true
|
|
} );
|
|
this.input.$element.addClass( 've-ui-mwExtensionInspector-input' );
|
|
|
|
// Initialization
|
|
this.$form.append( this.input.$element );
|
|
};
|
|
|
|
|
|
/**
|
|
* Handle the inspector being opened.
|
|
*/
|
|
ve.ui.MWExtensionInspector.prototype.onOpen = function () {
|
|
var extsrc = '';
|
|
|
|
// Parent method
|
|
ve.ui.SurfaceInspector.prototype.onOpen.call( this );
|
|
|
|
this.node = this.surface.getView().getFocusedNode();
|
|
|
|
if ( this.node ) {
|
|
extsrc = this.node.getModel().getAttribute( 'mw' ).body.extsrc;
|
|
}
|
|
|
|
// Direction of the input textarea should correspond to the
|
|
// direction of the surrounding content of the node itself
|
|
// rather than the GUI direction:
|
|
this.input.setRTL( this.node.$element.css( 'direction' ) === 'rtl' );
|
|
|
|
// Wait for animation to complete
|
|
setTimeout( ve.bind( function () {
|
|
// Setup input text
|
|
this.input.setValue( extsrc );
|
|
this.input.$input.focus().select();
|
|
}, this ), 200 );
|
|
};
|
|
|
|
/**
|
|
* Handle the inspector being closed.
|
|
*
|
|
* @param {string} action Action that caused the window to be closed
|
|
*/
|
|
ve.ui.MWExtensionInspector.prototype.onClose = function ( action ) {
|
|
var mwData,
|
|
surfaceModel = this.surface.getModel();
|
|
|
|
// Parent method
|
|
ve.ui.SurfaceInspector.prototype.onClose.call( this, action );
|
|
|
|
if ( this.node instanceof this.constructor.static.nodeView ) {
|
|
mwData = ve.copy( this.node.getModel().getAttribute( 'mw' ) );
|
|
mwData.body.extsrc = this.input.getValue();
|
|
surfaceModel.change(
|
|
ve.dm.Transaction.newFromAttributeChanges(
|
|
surfaceModel.getDocument(), this.node.getOuterRange().start, { 'mw': mwData }
|
|
)
|
|
);
|
|
} else {
|
|
mwData = {
|
|
'name': this.constructor.static.nodeModel.static.extensionName,
|
|
'attrs': {},
|
|
'body': {
|
|
'extsrc': this.input.getValue()
|
|
}
|
|
};
|
|
surfaceModel.getFragment().collapseRangeToEnd().insertContent( [
|
|
{
|
|
'type': this.constructor.static.nodeModel.static.name,
|
|
'attributes': {
|
|
'mw': mwData
|
|
}
|
|
},
|
|
{ 'type': '/' + this.constructor.static.nodeModel.static.name }
|
|
] );
|
|
}
|
|
};
|