mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
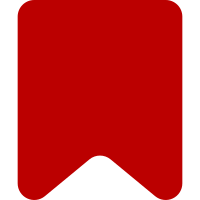
The activation timing was always a bit of a lie even pre-TargetLoader, because the timer only started when the first RL request for VE modules had loaded. But at least the process it covered was consistent, which is no longer true with TargetLoader. Now that we start the request for the HTML together with the RL request, the activation time might include some, all or none of the HTML request depending on how fast the RL request was. This change makes the activation timings more useful by measuring from the moment the user clicks "edit" to the moment the editor is done loading, which is what actually matters. * Moved start of activation timing to VPT init ** For mobile this falls back to when mw.Target#load is called; we'll have to fix that in MobileFrontend later * Moved end of activation timing out of TargetEvents#onSurfaceReady into individual onSurfaceReady handlers ** This is necessary because VPT's onSurfaceReady does quite a lot, and we want to include the time that takes in our measurements Change-Id: Ie44f0b839b39a2b3b22dcd86e20f0d1170cb6069
127 lines
3.1 KiB
JavaScript
127 lines
3.1 KiB
JavaScript
/*!
|
|
* VisualEditor MediaWiki Initialization MobileViewTarget class.
|
|
*
|
|
* @copyright 2011-2015 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
*
|
|
* @class
|
|
* @extends ve.init.mw.Target
|
|
*
|
|
* @constructor
|
|
* @param {jQuery} $container Container to render target into
|
|
* @param {Object} [config] Configuration options
|
|
* @cfg {number} [section] Number of the section target should scroll to
|
|
* @cfg {boolean} [isIos=false] Whether the platform is an iOS device
|
|
*/
|
|
ve.init.mw.MobileViewTarget = function VeInitMwMobileViewTarget( config ) {
|
|
var currentUri = new mw.Uri();
|
|
config = config || {};
|
|
|
|
// Parent constructor
|
|
ve.init.mw.Target.call(
|
|
this, mw.config.get( 'wgRelevantPageName' ), currentUri.query.oldid
|
|
);
|
|
|
|
this.section = config.section;
|
|
this.isIos = !!config.isIos;
|
|
|
|
// Events
|
|
this.connect( this, {
|
|
surfaceReady: 'onSurfaceReady'
|
|
} );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.init.mw.MobileViewTarget, ve.init.mw.Target );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.init.mw.MobileViewTarget.static.toolbarGroups = [
|
|
// Style
|
|
{ include: [ 'bold', 'italic' ] },
|
|
// Link
|
|
{ include: [ 'link' ] },
|
|
// Cite
|
|
{
|
|
header: OO.ui.deferMsg( 'visualeditor-toolbar-cite-label' ),
|
|
indicator: 'down',
|
|
type: 'list',
|
|
icon: 'reference',
|
|
title: OO.ui.deferMsg( 'visualeditor-toolbar-cite-label' ),
|
|
include: [ { group: 'cite' }, 'reference/existing' ]
|
|
}
|
|
];
|
|
|
|
ve.init.mw.MobileViewTarget.static.excludeCommands = [];
|
|
|
|
ve.init.mw.MobileViewTarget.static.name = 'mobile';
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Once surface is ready ready, init UI.
|
|
*/
|
|
ve.init.mw.MobileViewTarget.prototype.onSurfaceReady = function () {
|
|
this.restoreEditSection();
|
|
this.events.trackActivationComplete();
|
|
};
|
|
|
|
/**
|
|
* Create a surface.
|
|
*
|
|
* @method
|
|
* @param {ve.dm.Document} dmDoc Document model
|
|
* @param {Object} [config] Configuration options
|
|
* @returns {ve.ui.MobileSurface}
|
|
*/
|
|
ve.init.mw.MobileViewTarget.prototype.createSurface = function ( dmDoc, config ) {
|
|
return new ve.ui.MobileSurface( dmDoc, config );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.init.mw.MobileViewTarget.prototype.setupToolbar = function ( surface ) {
|
|
// Parent method
|
|
ve.init.mw.Target.prototype.setupToolbar.call( this, surface );
|
|
|
|
// Append the context to the toolbar
|
|
this.toolbar.$element.append( surface.context.$element );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.init.mw.MobileViewTarget.prototype.attachToolbar = function () {
|
|
// Move the toolbar to the overlay header
|
|
this.toolbar.$element.appendTo( '.overlay-header > .toolbar' );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.init.mw.MobileViewTarget.prototype.goToHeading = function ( headingNode ) {
|
|
this.scrollToHeading( headingNode );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.init.mw.MobileViewTarget.prototype.scrollToHeading = function ( headingNode ) {
|
|
var position,
|
|
target = this;
|
|
|
|
setTimeout( function () {
|
|
if ( target.isIos ) {
|
|
position = headingNode.$element.offset().top - target.toolbar.$element.height();
|
|
target.surface.$element.closest( '.overlay-content' ).scrollTop( position );
|
|
} else {
|
|
ve.init.mw.MobileViewTarget.super.prototype.scrollToHeading.call( target, headingNode );
|
|
}
|
|
} );
|
|
};
|