mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
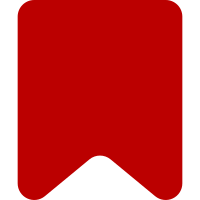
Instead of using @emits in both, use our custom @fires in production (JSDuck 4), and in the future it'll just naturally use the native one. This way we can also index oojs without issues, which seems to have started using @fires already. Change-Id: I7c3b56dd112626d57fa87ab995d205fb782a0149
78 lines
1.8 KiB
JavaScript
78 lines
1.8 KiB
JavaScript
/*!
|
|
* VisualEditor Registry class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Generic data registry.
|
|
*
|
|
* @abstract
|
|
* @mixins OO.EventEmitter
|
|
*
|
|
* @constructor
|
|
*/
|
|
ve.Registry = function VeRegistry() {
|
|
// Mixin constructors
|
|
OO.EventEmitter.call( this );
|
|
|
|
// Properties
|
|
this.registry = {};
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.mixinClass( ve.Registry, OO.EventEmitter );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* @event register
|
|
* @param {string} name
|
|
* @param {Mixed} data
|
|
*/
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Associate one or more symbolic names with some data.
|
|
*
|
|
* Only the base name will be registered, overriding any existing entry with the same base name.
|
|
*
|
|
* @method
|
|
* @param {string|string[]} name Symbolic name or list of symbolic names
|
|
* @param {Mixed} data Data to associate with symbolic name
|
|
* @fires register
|
|
* @throws {Error} Name argument must be a string or array
|
|
*/
|
|
ve.Registry.prototype.register = function ( name, data ) {
|
|
if ( typeof name !== 'string' && !ve.isArray( name ) ) {
|
|
throw new Error( 'Name argument must be a string or array, cannot be a ' + typeof name );
|
|
}
|
|
var i, len;
|
|
if ( ve.isArray( name ) ) {
|
|
for ( i = 0, len = name.length; i < len; i++ ) {
|
|
this.register( name[i], data );
|
|
}
|
|
} else if ( typeof name === 'string' ) {
|
|
this.registry[name] = data;
|
|
this.emit( 'register', name, data );
|
|
} else {
|
|
throw new Error( 'Name must be a string or array of strings, cannot be a ' + typeof name );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Gets data for a given symbolic name.
|
|
*
|
|
* Lookups are done using the base name.
|
|
*
|
|
* @method
|
|
* @param {string} name Symbolic name
|
|
* @returns {Mixed|undefined} Data associated with symbolic name
|
|
*/
|
|
ve.Registry.prototype.lookup = function ( name ) {
|
|
return this.registry[name];
|
|
};
|