mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-28 12:46:45 +00:00
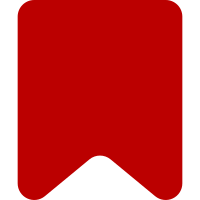
Objectives: * Make drop down tools look more like buttons and less like inputs, since they aren't text input and are buttons * Make context toolbars inside surface widgets render correctly * Show outlines of groups on hover to hint tool relationships * Make neighboring active tools look cleaner Changes: ve.ui.Tool.css * Merge ButtonTool and DropdownTool styles as much as possible * Add styles for DropdownTool active states * Only round the corners of the first and last tool in a group * Soften the borders between consecutive active tools ve.ui.Toolbar.css * Add border to groups on hover ve.ui.Widget.css * Isolate surface widget toolbar styles by using stricter selector ve.ui.Tool.js * Fix incorrect capitalization of class name ve.ui.SurfaceWidget.js * Add classes to toolbar and surface for better style targeting Change-Id: Ib5ae8f705ef1e9c481e5bdf8c8dcef9c1eb22c4d
109 lines
2.3 KiB
JavaScript
109 lines
2.3 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface SurfaceWidget class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Creates an ve.ui.SurfaceWidget object.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
* @extends ve.ui.Widget
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.ElementLinearData} data Content data
|
|
* @param {Object} [config] Config options
|
|
* @cfg {Object[]} [tools] Toolbar configuration
|
|
* @cfg {string[]} [commands] List of supported commands
|
|
*/
|
|
ve.ui.SurfaceWidget = function VeUiSurfaceWidget( data, config ) {
|
|
// Config intialization
|
|
config = config || {};
|
|
|
|
// Parent constructor
|
|
ve.ui.Widget.call( this, config );
|
|
|
|
// Properties
|
|
this.surface = new ve.ui.Surface( data, { '$$': this.$$ } );
|
|
this.toolbar = new ve.ui.SurfaceToolbar( this.surface, { '$$': this.$$ } );
|
|
|
|
// Initialization
|
|
this.surface.$.addClass( 've-ui-surfaceWidget-surface' );
|
|
this.toolbar.$.addClass( 've-ui-surfaceWidget-toolbar' );
|
|
this.$
|
|
.addClass( 've-ui-surfaceWidget' )
|
|
.append( this.toolbar.$, this.surface.$ );
|
|
if ( config.tools ) {
|
|
this.toolbar.addTools( config.tools );
|
|
}
|
|
if ( config.commands ) {
|
|
this.surface.addCommands( config.commands );
|
|
}
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.SurfaceWidget, ve.ui.Widget );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Get surface.
|
|
*
|
|
* @method
|
|
* @returns {ve.ui.Surface} Surface
|
|
*/
|
|
ve.ui.SurfaceWidget.prototype.getSurface = function () {
|
|
return this.surface;
|
|
};
|
|
|
|
/**
|
|
* Get toolbar.
|
|
*
|
|
* @method
|
|
* @returns {ve.ui.Toolbar} Toolbar
|
|
*/
|
|
ve.ui.SurfaceWidget.prototype.getToolbar = function () {
|
|
return this.toolbar;
|
|
};
|
|
|
|
/**
|
|
* Get content data.
|
|
*
|
|
* @method
|
|
* @returns {ve.dm.ElementLinearData} Content data
|
|
*/
|
|
ve.ui.SurfaceWidget.prototype.getContent = function () {
|
|
return this.surface.getModel().getDocument().getData();
|
|
};
|
|
|
|
/**
|
|
* Initialize surface and toolbar.
|
|
*
|
|
* Widget must be attached to DOM before initializing.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.SurfaceWidget.prototype.initialize = function () {
|
|
this.toolbar.initialize();
|
|
this.surface.initialize();
|
|
this.surface.view.documentView.documentNode.$.focus();
|
|
};
|
|
|
|
/**
|
|
* Destroy surface and toolbar.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.SurfaceWidget.prototype.destroy = function () {
|
|
if ( this.surface ) {
|
|
this.surface.destroy();
|
|
}
|
|
if ( this.toolbar ) {
|
|
this.toolbar.destroy();
|
|
}
|
|
this.$.remove();
|
|
};
|