mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-06 06:29:11 +00:00
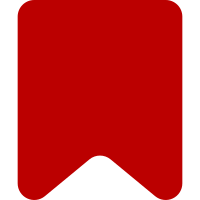
Objectives: * Split ve.Surface into ve.Editor and ve.ui.Surface * Move actions, triggers and commands to ve.ui * Move toolbar wrapping, floating, shadow and actions functionality to configurable options of ve.ui.Toolbar * Make ve.ce.Surface and ve.ui.Surface inherit ve.Element and use this.$$ for iframe friendliness * Make the toolbar separately initialized so it's possible to have a surface without one, as well as control where the toolbar is Some change notes: VisualEditor.php * Added standalone module for mediawiki integrated unit testing ve.ce.Surface.js * Remove requirement to pass in an attached container to construct object * Inherit ve.Element and use this.$$ instead of $ * Make getSelectionRect iframe friendly * Move most of the initialize stuff to a new initialize method to be called after the surface is attached to the DOM ve.init.mw.ViewPageTarget.js * Merge toolbar functions into setup/teardown methods * Add toolbar manually (since it's not added by the surface anymore) ve.init.sa.Target.js * Update new init procedure for editor, surface and toolbar separately * Move toolbar floating stuff to ve.Toolbar Change-Id: If91a9d6e76a8be8d1b5a2566394765a37d29a8a7
75 lines
1.8 KiB
JavaScript
75 lines
1.8 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface UndoButtonTool class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* UserInterface undo button tool.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.ButtonTool
|
|
* @constructor
|
|
* @param {ve.ui.Toolbar} toolbar
|
|
* @param {Object} [config] Config options
|
|
*/
|
|
ve.ui.UndoButtonTool = function VeUiUndoButtonTool( toolbar, config ) {
|
|
// Parent constructor
|
|
ve.ui.ButtonTool.call( this, toolbar, config );
|
|
|
|
// Events
|
|
this.toolbar.getSurface().getModel().connect( this, { 'history': 'onUpdateState' } );
|
|
|
|
// Initialization
|
|
this.setDisabled( true );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.UndoButtonTool, ve.ui.ButtonTool );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.ui.UndoButtonTool.static.name = 'undo';
|
|
|
|
ve.ui.UndoButtonTool.static.icon = 'undo';
|
|
|
|
ve.ui.UndoButtonTool.static.titleMessage = 'visualeditor-historybutton-undo-tooltip';
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Handle the button being clicked.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.UndoButtonTool.prototype.onClick = function () {
|
|
this.toolbar.getSurface().execute( 'history', 'undo' );
|
|
};
|
|
|
|
/**
|
|
* Handle the toolbar state being updated.
|
|
*
|
|
* @method
|
|
* @param {ve.dm.Node[]} nodes List of nodes covered by the current selection
|
|
* @param {ve.dm.AnnotationSet} full Annotations that cover all of the current selection
|
|
* @param {ve.dm.AnnotationSet} partial Annotations that cover some or all of the current selection
|
|
*/
|
|
ve.ui.UndoButtonTool.prototype.onUpdateState = function () {
|
|
this.setDisabled( !this.toolbar.getSurface().getModel().hasPastState() );
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.ui.toolFactory.register( 'undo', ve.ui.UndoButtonTool );
|
|
|
|
ve.ui.commandRegistry.register(
|
|
'undo', 'history', 'undo'
|
|
);
|
|
|
|
ve.ui.triggerRegistry.register(
|
|
'undo', { 'mac': new ve.ui.Trigger( 'cmd+z' ), 'pc': new ve.ui.Trigger( 'ctrl+z' ) }
|
|
);
|
|
|