mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 11:16:51 +00:00
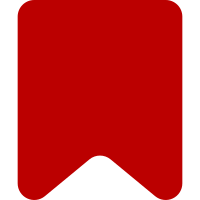
Detecting page status in a similar way as WikiEditor inspector. Disabled accept button now behaves appropriately. Accept button status is now evaluated on enter or submit. Change-Id: Ibfef6ffd87cb9a71e37242d6214d0f8e3af2e2c0
108 lines
2.3 KiB
JavaScript
108 lines
2.3 KiB
JavaScript
/**
|
|
* VisualEditor user interface Inspector class.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Creates an ve.ui.Inspector object.
|
|
*
|
|
* @class
|
|
* @constructor
|
|
* @extends {ve.EventEmitter}
|
|
* @param {ve.ui.Toolbar} toolbar
|
|
* @param {ve.ui.Context} context
|
|
*/
|
|
ve.ui.Inspector = function VeUiInspector( toolbar, context ) {
|
|
// Inheritance
|
|
ve.EventEmitter.call( this );
|
|
|
|
if ( !toolbar || !context ) {
|
|
return;
|
|
}
|
|
|
|
// Properties
|
|
this.toolbar = toolbar;
|
|
this.context = context;
|
|
this.$ = $( '<div class="ve-ui-inspector"></div>', context.inspectorDoc );
|
|
this.$closeButton = $(
|
|
'<div class="ve-ui-inspector-button ve-ui-inspector-closeButton"></div>',
|
|
context.inspectorDoc
|
|
);
|
|
this.$acceptButton = $(
|
|
'<div class="ve-ui-inspector-button ve-ui-inspector-acceptButton"></div>',
|
|
context.inspectorDoc
|
|
);
|
|
this.$form = $( '<form>', context.inspectorDoc );
|
|
|
|
// DOM Changes
|
|
this.$.append( this.$closeButton, this.$acceptButton, this.$form );
|
|
|
|
// Events
|
|
this.$closeButton.on( {
|
|
'click': function () {
|
|
context.closeInspector( false );
|
|
}
|
|
} );
|
|
this.$acceptButton.on( {
|
|
'click': function () {
|
|
if ( $( this ).hasClass( 've-ui-inspector-button-disabled' ) ) {
|
|
return;
|
|
}
|
|
context.closeInspector( true );
|
|
}
|
|
} );
|
|
this.$form.on( {
|
|
'submit': ve.bind( this.onSubmit, this ),
|
|
'keydown': ve.bind( this.onKeyDown, this )
|
|
} );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.Inspector, ve.EventEmitter );
|
|
|
|
/* Methods */
|
|
|
|
ve.ui.Inspector.prototype.onSubmit = function ( e ) {
|
|
e.preventDefault();
|
|
if ( this.$acceptButton.hasClass( 've-ui-inspector-button-disabled' ) ) {
|
|
return;
|
|
}
|
|
this.context.closeInspector( true );
|
|
return false;
|
|
};
|
|
|
|
ve.ui.Inspector.prototype.onKeyDown = function ( e ) {
|
|
// Escape
|
|
if ( e.which === 27 ) {
|
|
this.context.closeInspector( false );
|
|
e.preventDefault();
|
|
return false;
|
|
}
|
|
};
|
|
|
|
ve.ui.Inspector.prototype.open = function () {
|
|
// Prepare to open
|
|
if ( this.prepareOpen ) {
|
|
this.prepareOpen();
|
|
}
|
|
// Show
|
|
this.$.show();
|
|
this.context.closeMenu();
|
|
// Open
|
|
if ( this.onOpen ) {
|
|
this.onOpen();
|
|
}
|
|
this.emit( 'open' );
|
|
};
|
|
|
|
ve.ui.Inspector.prototype.close = function ( accept ) {
|
|
this.$.hide();
|
|
if ( this.onClose ) {
|
|
this.onClose( accept );
|
|
}
|
|
this.emit( 'close' );
|
|
};
|