mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 11:16:51 +00:00
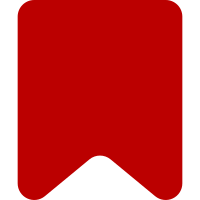
This allows us to put other internal data in there in the future. Also passing it through the Node constructor properly now. * ve.dm.Node ** Rename fringeWhitespace property to internal ** Add internal parameter to constructor ** Remove setFringeWhitespace() * Increase the number of parameters passed through by ve.Factory to 3 * Pass through .internal from linmod to nodeFactory in ve.dm.Document * ve.dm.Converter ** Rename .fringeWhitespace to .internal.whitespace and make it an array ** Store a temporary reference to .internal in domElement.veInternal * Add internal to all node constructors except TextNode Tests: * Update for fringeWhitespace->internal rename * Add third parameter to ve.Factory tests * Add .internal to getNodeTreeSummary Change-Id: If20c0bb78fee3efa55f72e51e7fc261283358de7
124 lines
3.5 KiB
JavaScript
124 lines
3.5 KiB
JavaScript
( function ( QUnit ) {
|
|
|
|
/**
|
|
* Builds a summary of a node tree.
|
|
*
|
|
* Generated summaries contain node types, lengths, outer lengths, attributes and summaries for
|
|
* each child recursively. It's simple and fast to use deepEqual on this.
|
|
*
|
|
* @method
|
|
* @param {ve.Node} node Node tree to summarize
|
|
* @param {Boolean} [shallow] Do not summarize each child recursively
|
|
* @returns {Object} Summary of node tree
|
|
*/
|
|
function getNodeTreeSummary( node, shallow ) {
|
|
var i,
|
|
summary = {
|
|
'getType': node.getType(),
|
|
'getLength': node.getLength(),
|
|
'getOuterLength': node.getOuterLength(),
|
|
'attributes': node.attributes,
|
|
'internal': node.internal
|
|
};
|
|
if ( node.children !== undefined ) {
|
|
summary['children.length'] = node.children.length;
|
|
if ( !shallow ) {
|
|
summary.children = [];
|
|
for ( i = 0; i < node.children.length; i++ ) {
|
|
summary.children.push( getNodeTreeSummary( node.children[i] ) );
|
|
}
|
|
}
|
|
}
|
|
return summary;
|
|
}
|
|
|
|
/**
|
|
* Builds a summary of a node selection.
|
|
*
|
|
* Generated summaries contain length of results as well as node summaries, ranges, indexes, indexes
|
|
* within parent and node ranges for each result. It's simple and fast to use deepEqual on this.
|
|
*
|
|
* @method
|
|
* @param {Object[]} selection Selection to summarize
|
|
* @returns {Object} Summary of selection
|
|
*/
|
|
function getNodeSelectionSummary( selection ) {
|
|
var i,
|
|
summary = {
|
|
'length': selection.length
|
|
};
|
|
if ( selection.length ) {
|
|
summary.results = [];
|
|
for ( i = 0; i < selection.length; i++ ) {
|
|
summary.results.push( {
|
|
'node': getNodeTreeSummary( selection[i].node, true ),
|
|
'range': selection[i].range,
|
|
'index': selection[i].index,
|
|
'indexInNode': selection[i].indexInNode,
|
|
'nodeRange': selection[i].nodeRange,
|
|
'nodeOuterRange': selection[i].nodeOuterRange
|
|
} );
|
|
}
|
|
}
|
|
return summary;
|
|
}
|
|
|
|
/**
|
|
* Builds a summary of an HTML element.
|
|
*
|
|
* Summaries include node name, text, attributes and recursive summaries of children.
|
|
*
|
|
* @method
|
|
* @param {HTMLElement} element Element to summarize
|
|
* @returns {Object} Summary of element
|
|
*/
|
|
function getDomElementSummary( element ) {
|
|
var $element = $( element ),
|
|
summary = {
|
|
'type': element.nodeName.toLowerCase(),
|
|
'text': $element.text(),
|
|
'attributes': {},
|
|
'children': []
|
|
},
|
|
i;
|
|
// Gather attributes
|
|
for ( i = 0; i < element.attributes.length; i++ ) {
|
|
summary.attributes[element.attributes[i].name] = element.attributes[i].value;
|
|
}
|
|
// Summarize children
|
|
for ( i = 0; i < element.children.length; i++ ) {
|
|
summary.children.push( getDomElementSummary( element.children[i] ) );
|
|
}
|
|
return summary;
|
|
}
|
|
|
|
QUnit.assert.equalNodeTree = function ( actual, expected, shallow, message ) {
|
|
if ( typeof shallow === 'string' && arguments.length === 3 ) {
|
|
message = shallow;
|
|
shallow = undefined;
|
|
}
|
|
var actualSummary = getNodeTreeSummary( actual, shallow ),
|
|
expectedSummary = getNodeTreeSummary( expected, shallow );
|
|
QUnit.push(
|
|
QUnit.equiv( actualSummary, expectedSummary ), actualSummary, expectedSummary, message
|
|
);
|
|
};
|
|
|
|
QUnit.assert.equalNodeSelection = function ( actual, expected, message ) {
|
|
var actualSummary = getNodeSelectionSummary( actual ),
|
|
expectedSummary = getNodeSelectionSummary( expected );
|
|
QUnit.push(
|
|
QUnit.equiv( actualSummary, expectedSummary ), actualSummary, expectedSummary, message
|
|
);
|
|
};
|
|
|
|
QUnit.assert.equalDomElement = function ( actual, expected, message ) {
|
|
var actualSummary = getDomElementSummary( actual ),
|
|
expectedSummary = getDomElementSummary( expected );
|
|
QUnit.push(
|
|
QUnit.equiv( actualSummary, expectedSummary ), actualSummary, expectedSummary, message
|
|
);
|
|
};
|
|
|
|
}( QUnit ) );
|