mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 02:23:58 +00:00
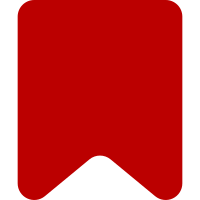
A @method annotation is only necessary when the docblock is not directly followed by a function declaration (in which case JSDuck assumes it documents a property), e.g. when defining an abstract function or referencing a function from another library. I verified that JSDuck generates exactly the same output before and after this change (docs/data-<hash>.js files are identical). Change-Id: I7edf51a8560ab9978b42800ab1026f0b5555c3bf
85 lines
2.3 KiB
JavaScript
85 lines
2.3 KiB
JavaScript
/*!
|
|
* VisualEditor ContentEditable MWGalleryNode class.
|
|
*
|
|
* @copyright 2011-2019 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* ContentEditable MediaWiki gallery node.
|
|
*
|
|
* @class
|
|
* @extends ve.ce.BranchNode
|
|
* @mixins ve.ce.FocusableNode
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.MWGalleryNode} model Model to observe
|
|
* @param {Object} [config] Configuration options
|
|
*/
|
|
ve.ce.MWGalleryNode = function VeCeMWGalleryNode() {
|
|
// Parent constructor
|
|
ve.ce.MWGalleryNode.super.apply( this, arguments );
|
|
|
|
// DOM hierarchy for MWGalleryNode:
|
|
// <ul> this.$element (gallery mw-gallery-{mode})
|
|
// <li> ve.ce.MWGalleryCaptionNode (gallerycaption)
|
|
// <li> ve.ce.MWGalleryImageNode (gallerybox)
|
|
// <li> ve.ce.MWGalleryImageNode (gallerybox)
|
|
// ⋮
|
|
|
|
// Mixin constructors
|
|
ve.ce.FocusableNode.call( this, this.$element );
|
|
|
|
// Events
|
|
this.model.connect( this, { update: 'updateInvisibleIcon' } );
|
|
this.model.connect( this, { update: 'onUpdate' } );
|
|
|
|
// Initialization
|
|
this.onUpdate();
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ce.MWGalleryNode, ve.ce.BranchNode );
|
|
|
|
OO.mixinClass( ve.ce.MWGalleryNode, ve.ce.FocusableNode );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.ce.MWGalleryNode.static.name = 'mwGallery';
|
|
|
|
ve.ce.MWGalleryNode.static.tagName = 'ul';
|
|
|
|
ve.ce.MWGalleryNode.static.iconWhenInvisible = 'imageGallery';
|
|
|
|
ve.ce.MWGalleryNode.static.primaryCommandName = 'gallery';
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Handle model update events.
|
|
*/
|
|
ve.ce.MWGalleryNode.prototype.onUpdate = function () {
|
|
var mwAttrs, defaults, mode, imageWidth, imagePadding;
|
|
|
|
mwAttrs = this.model.getAttribute( 'mw' ).attrs;
|
|
defaults = mw.config.get( 'wgVisualEditorConfig' ).galleryOptions;
|
|
mode = mwAttrs.mode || defaults.mode;
|
|
|
|
this.$element
|
|
.attr( 'class', mwAttrs.class )
|
|
.attr( 'style', mwAttrs.style )
|
|
.addClass( 'gallery mw-gallery-' + mode );
|
|
|
|
if ( mwAttrs.perrow && ( mode === 'traditional' || mode === 'nolines' ) ) {
|
|
// Magic 30 and 8 matches the code in ve.ce.MWGalleryImageNode
|
|
imageWidth = parseInt( mwAttrs.widths || defaults.imageWidth );
|
|
imagePadding = ( mode === 'traditional' ? 30 : 0 );
|
|
this.$element.css( 'max-width', mwAttrs.perrow * ( imageWidth + imagePadding + 8 ) );
|
|
}
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.ce.nodeFactory.register( ve.ce.MWGalleryNode );
|