mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
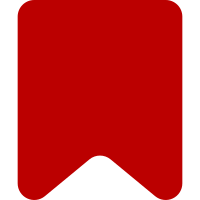
This works just fine, as also previously tested/proven by ve.cloneObject, which uses the same concept of creating an object identical to what invoking the constructor with "new" would do, but without invoking the constructor function (which has side- effects). Except in this case we do invoke the constructor function, but we can't use new in ve.Factory because of the arbitrary number of arguments. Added a test to assert that 3+ arguments and that instanceof work as expected. Change-Id: If0add3da7475886e476900044acda2ba7d01fb11
66 lines
1.6 KiB
JavaScript
66 lines
1.6 KiB
JavaScript
/**
|
|
* VisualEditor Factory tests.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
QUnit.module( 've.Factory' );
|
|
|
|
/* Stubs */
|
|
|
|
ve.FactoryObjectStub = function VeFactoryObjectStub( a, b, c, d ) {
|
|
this.a = a;
|
|
this.b = b;
|
|
this.c = c;
|
|
this.d = d;
|
|
};
|
|
|
|
/* Tests */
|
|
|
|
QUnit.test( 'register', 1, function ( assert ) {
|
|
var factory = new ve.Factory();
|
|
assert.throws(
|
|
function () {
|
|
factory.register( 'factory-object-stub', 'not-a-function' );
|
|
},
|
|
Error,
|
|
'Throws an exception when trying to register a non-function value as a constructor'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'create', 3, function ( assert ) {
|
|
var obj,
|
|
factory = new ve.Factory();
|
|
|
|
assert.throws(
|
|
function () {
|
|
factory.create( 'factory-object-stub', 23, 'foo', { 'bar': 'baz' } );
|
|
},
|
|
Error,
|
|
'Throws an exception when trying to create a object of an unregistered type'
|
|
);
|
|
|
|
factory.register( 'factory-object-stub', ve.FactoryObjectStub );
|
|
|
|
obj = factory.create( 'factory-object-stub', 16, 'foo', { 'baz': 'quux' }, 5 );
|
|
|
|
assert.deepEqual(
|
|
obj,
|
|
new ve.FactoryObjectStub( 16, 'foo', { 'baz': 'quux' }, 5 ),
|
|
'Creates an object of the registered type and passes through arguments'
|
|
);
|
|
|
|
assert.strictEqual(
|
|
obj instanceof ve.FactoryObjectStub,
|
|
true,
|
|
'Creates an object that is an instanceof the registered constructor'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'lookup', 1, function ( assert ) {
|
|
var factory = new ve.Factory();
|
|
factory.register( 'factory-object-stub', ve.FactoryObjectStub );
|
|
assert.strictEqual( factory.lookup( 'factory-object-stub' ), ve.FactoryObjectStub );
|
|
} );
|