mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-24 14:33:59 +00:00
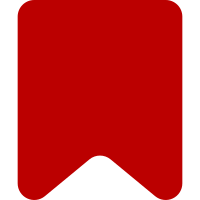
Fleshes out ve.dm.Annotation to a class. Annotations in the linear model will be instances of a subclass of ve.dm.Annotation. Annotations are defined by subclassing ve.dm.Annotation and registering this subclass with ve.dm.AnnotationFactory. ve.dm.AnnotationFactory keeps track of which annotation classes are known, and has code to match an HTML element to an annotation class, for use in the converter. Change-Id: I68802bdb8736ced1f9e04ee49c623944b448141c
100 lines
2.8 KiB
PHP
100 lines
2.8 KiB
PHP
<?php
|
|
/**
|
|
* VisualEditor extension hooks
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
class VisualEditorHooks {
|
|
/** List of skins VisualEditor integration supports */
|
|
protected static $supportedSkins = array( 'vector', 'apex', 'monobook' );
|
|
|
|
/**
|
|
* Adds VisualEditor JS to the output if in the correct namespace.
|
|
*
|
|
* This is attached to the MediaWiki 'BeforePageDisplay' hook.
|
|
*
|
|
* @param $output OutputPage
|
|
* @param $skin Skin
|
|
*/
|
|
public static function onBeforePageDisplay( &$output, &$skin ) {
|
|
global $wgTitle;
|
|
if (
|
|
in_array( $skin->getSkinName(), self::$supportedSkins ) &&
|
|
(
|
|
// Article in the VisualEditor namespace
|
|
$wgTitle->getNamespace() === NS_VISUALEDITOR ||
|
|
// Special page action for an article in the VisualEditor namespace
|
|
$skin->getRelevantTitle()->getNamespace() === NS_VISUALEDITOR
|
|
)
|
|
) {
|
|
$output->addModules( array( 'ext.visualEditor.viewPageTarget' ) );
|
|
}
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Adds extra variables to the page config.
|
|
*
|
|
* This is attached to the MediaWiki 'MakeGlobalVariablesScript' hook.
|
|
*/
|
|
public static function onMakeGlobalVariablesScript( &$vars ) {
|
|
global $wgUser, $wgTitle;
|
|
$vars['wgVisualEditor'] = array(
|
|
'isPageWatched' => $wgUser->isWatched( $wgTitle )
|
|
);
|
|
return true;
|
|
}
|
|
|
|
public static function onResourceLoaderTestModules( array &$testModules, ResourceLoader &$resourceLoader ) {
|
|
$testModules['qunit']['ext.visualEditor.test'] = array(
|
|
'scripts' => array(
|
|
// QUnit plugin
|
|
've.qunit.js',
|
|
// VisualEditor Tests
|
|
've.test.js',
|
|
've.example.js',
|
|
've.Document.test.js',
|
|
've.Node.test.js',
|
|
've.BranchNode.test.js',
|
|
've.LeafNode.test.js',
|
|
've.Factory.test.js',
|
|
// VisualEditor DataModel Tests
|
|
'dm/ve.dm.example.js',
|
|
'dm/ve.dm.NodeFactory.test.js',
|
|
'dm/ve.dm.Node.test.js',
|
|
'dm/ve.dm.Converter.test.js',
|
|
'dm/ve.dm.BranchNode.test.js',
|
|
'dm/ve.dm.LeafNode.test.js',
|
|
'dm/nodes/ve.dm.TextNode.test.js',
|
|
'dm/ve.dm.Document.test.js',
|
|
'dm/ve.dm.DocumentSynchronizer.test.js',
|
|
'dm/ve.dm.Transaction.test.js',
|
|
'dm/ve.dm.TransactionProcessor.test.js',
|
|
'dm/ve.dm.Surface.test.js',
|
|
'dm/ve.dm.SurfaceFragment.test.js',
|
|
'dm/ve.dm.AnnotationFactory.test.js',
|
|
// VisualEditor ContentEditable Tests
|
|
'ce/ve.ce.test.js',
|
|
'ce/ve.ce.Document.test.js',
|
|
'ce/ve.ce.NodeFactory.test.js',
|
|
'ce/ve.ce.Node.test.js',
|
|
'ce/ve.ce.BranchNode.test.js',
|
|
'ce/ve.ce.LeafNode.test.js',
|
|
'ce/nodes/ve.ce.TextNode.test.js',
|
|
),
|
|
'dependencies' => array(
|
|
'ext.visualEditor.core',
|
|
'ext.visualEditor.viewPageTarget',
|
|
),
|
|
'localBasePath' => dirname( __FILE__ ) . '/modules/ve/test',
|
|
'remoteExtPath' => 'VisualEditor/modules/ve/test',
|
|
);
|
|
|
|
return true;
|
|
}
|
|
}
|