mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-28 00:00:49 +00:00
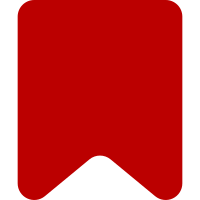
New changes: db0f21d03 ve.ce.Surface: Check dataTransfer.files is non-empty e1cd78933 [BREAKING CHANGE] Move selection handling code to SelectionManager b4b63a463 Move drag/drop handling code to DragDropHandler 540272769 Use the onPaste handler for dropped content d3f9d8485 ClipboardHandler tests: Run tests in series 1232c0420 Tests: Introduce ve.dm.example.annotateText to simplify runs of annotated text 69d7ca482 Apply an annotation to imported (pasted/dropped) text 0e82a6db8 ve.ce.ClipboardHandler: Fix async test running Added files: - src/ce/annotations/ve.ce.ImportedDataAnnotation.js - src/ce/ve.ce.DragDropHandler.js - src/ce/ve.ce.SelectionManager.js - src/dm/annotations/ve.dm.ImportedDataAnnotation.js - tests/ce/ve.ce.DragDropHandler.test.js Local changes: - Implement new selection manager architecture Added files: - src/ce/annotations/ve.ce.ImportedDataAnnotation.js - src/ce/ve.ce.DragDropHandler.js - src/ce/ve.ce.SelectionManager.js - src/dm/annotations/ve.dm.ImportedDataAnnotation.js - tests/ce/ve.ce.DragDropHandler.test.js Bug: T371996 Bug: T377427 Bug: T78696 Change-Id: If8d38246badf919c32915beda7c9a14f16e62a8a
97 lines
3.9 KiB
JavaScript
97 lines
3.9 KiB
JavaScript
/*!
|
|
* VisualEditor MediaWiki-specific ContentEditable Surface tests.
|
|
*
|
|
* @copyright See AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
QUnit.module( 've.ce.Surface (MW)', ve.test.utils.newMwEnvironment() );
|
|
|
|
/* Tests */
|
|
|
|
QUnit.test( 'beforePaste/afterPaste', ( assert ) => {
|
|
const cases = [
|
|
{
|
|
documentHtml: '<p></p>',
|
|
rangeOrSelection: new ve.Range( 1 ),
|
|
pasteHtml: '<span typeof="mw:Entity" id="mwAB">-</span><span typeof="mw:Entity" id="mw-reference-cite">-</span>',
|
|
fromVe: true,
|
|
expectedRangeOrSelection: new ve.Range( 5 ),
|
|
expectedHtml: '<p><span typeof="mw:Entity">-</span><span typeof="mw:Entity" id="mw-reference-cite">-</span></p>',
|
|
msg: 'RESTBase IDs stripped'
|
|
},
|
|
{
|
|
documentHtml: '<p></p>',
|
|
rangeOrSelection: new ve.Range( 1 ),
|
|
pasteHtml: '<span typeof="mw:Entity" id="mwAB">-</span><span typeof="mw:Entity" id="mw-reference-cite">-</span>',
|
|
clipboardHandlerHtml: '<span>-</span><span>-</span>',
|
|
fromVe: true,
|
|
expectedRangeOrSelection: new ve.Range( 5 ),
|
|
expectedHtml: '<p><span typeof="mw:Entity">-</span><span typeof="mw:Entity" id="mw-reference-cite">-</span></p>',
|
|
msg: 'RESTBase IDs still stripped if used when important attributes dropped'
|
|
},
|
|
{
|
|
documentHtml: '<p></p>',
|
|
rangeOrSelection: new ve.Range( 1 ),
|
|
pasteHtml: 'a<sup id="cite_ref-1" class="reference"><a href="./Article#cite_note-1">[1]</a></sup>b',
|
|
expectedRangeOrSelection: new ve.Range( 3 ),
|
|
expectedHtml: '<p>ab</p>',
|
|
msg: 'Read mode references stripped'
|
|
},
|
|
{
|
|
documentHtml: '<p></p>',
|
|
rangeOrSelection: new ve.Range( 1 ),
|
|
pasteHtml: 'a<sup typeof="mw:Extension/ref" data-mw="{}" class="mw-ref reference" about="#mwt1" id="cite_ref-foo-0" rel="dc:references"><a href="./Article#cite_note-foo-0"><span class="mw-reflink-text ve-pasteProtect">[1]</span></a></sup>b',
|
|
expectedRangeOrSelection: new ve.Range( 5 ),
|
|
expectedHtml: '<p>a<sup typeof="mw:Extension/ref" data-mw="{"name":"ref"}" class="mw-ref reference"><a><span class="mw-reflink-text"><span class="cite-bracket">[</span>1<span class="cite-bracket">]</span></span></a></sup>b</p>',
|
|
msg: 'VE references not stripped'
|
|
},
|
|
{
|
|
documentHtml: '<p></p>',
|
|
rangeOrSelection: new ve.Range( 1 ),
|
|
pasteHtml: '<a href="https://example.net/">Lorem</a> <a href="not-a-protocol:Some%20text">ipsum</a> <a href="mailto:example@example.net">dolor</a> <a href="javascript:alert()">sit amet</a>',
|
|
expectedRangeOrSelection: new ve.Range( 27 ),
|
|
// hrefs with invalid protocols get removed by DOMPurify, and these links become spans in
|
|
// ve.dm.LinkAnnotation.static.toDataElement (usually the span is stripped later)
|
|
expectedHtml: '<p>Lorem <span>ipsum</span> dolor <span>sit amet</span></p>',
|
|
config: {
|
|
importRules: {
|
|
external: {
|
|
blacklist: {
|
|
'link/mwExternal': true
|
|
}
|
|
}
|
|
}
|
|
},
|
|
msg: 'External links stripped'
|
|
},
|
|
{
|
|
documentHtml: '<p></p>',
|
|
rangeOrSelection: new ve.Range( 1 ),
|
|
pasteHtml: '<a href="https://example.net/">Lorem</a> <a href="not-a-protocol:Some%20text">ipsum</a> <a href="mailto:example@example.net">dolor</a> <a href="javascript:alert()">sit amet</a>',
|
|
expectedRangeOrSelection: new ve.Range( 27 ),
|
|
// hrefs with invalid protocols get removed by DOMPurify, and these links become spans in
|
|
// ve.dm.LinkAnnotation.static.toDataElement (usually the span is stripped later)
|
|
expectedHtml: '<p><a href="https://example.net/" rel="mw:ExtLink">Lorem</a> <span>ipsum</span> <a href="mailto:example@example.net" rel="mw:ExtLink">dolor</a> <span>sit amet</span></p>',
|
|
config: {
|
|
importRules: {
|
|
external: {
|
|
blacklist: {
|
|
'link/mwExternal': false
|
|
}
|
|
}
|
|
}
|
|
},
|
|
msg: 'External links not stripped, but only some protocols allowed'
|
|
}
|
|
];
|
|
|
|
const done = assert.async();
|
|
( async function () {
|
|
for ( const caseItem of cases ) {
|
|
await ve.test.utils.runSurfacePasteTest( assert, caseItem );
|
|
}
|
|
done();
|
|
}() );
|
|
} );
|