mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-28 04:36:49 +00:00
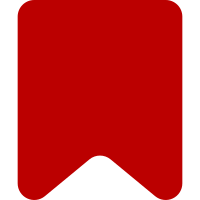
We were populating empty content nodes with zero-length text nodes to make round-trip tests in the test suite work (otherwise blanking a paragraph leaves behind a zero-length text node whereas creating an empty paragraph does not), but the empty nodes are causing problems in CE apparently. * Do not create empty text nodes when constructing a node tree * Be more careful with text-only replacements: ** Don't resize a text node to zero, remove it instead ** There may not be a text node to resize at all, build it in that case ** Switch nodeRange to nodeOuterRange, this was probably broken before Tests: * Change test case for zero-length text node to assert that there is *no* zero-length text node :) * Remove a test case concerning an empty text node from the ve.ce.TextNode suite Change-Id: Ie677457f2f0a7823a517ba3077b844ef52a20fcc
284 lines
6.3 KiB
JavaScript
284 lines
6.3 KiB
JavaScript
/**
|
|
* VisualEditor content editable TextNode tests.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
QUnit.module( 've.ce.TextNode' );
|
|
|
|
/* Tests */
|
|
|
|
QUnit.test( 'getHtml', function ( assert ) {
|
|
var i, len, cases;
|
|
|
|
cases = [
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
'a',
|
|
'b',
|
|
'c',
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': 'abc'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
['a', [ { 'type': 'textStyle/bold' } ]],
|
|
['b', [ { 'type': 'textStyle/bold' } ]],
|
|
['c', [ { 'type': 'textStyle/bold' } ]],
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': '<b>abc</b>'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
['a', [ { 'type': 'textStyle/bold' } ]],
|
|
'b',
|
|
['c', [ { 'type': 'textStyle/italic' } ]],
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': '<b>a</b>b<i>c</i>'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
['a', [
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
['b', [
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
['c', [
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': '<b><i><u>abc</u></i></b>'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
['a', [
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
['b', [
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' }
|
|
]],
|
|
['c', [
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' }
|
|
]],
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': '<b><i><u>abc</u></i></b>'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
['a', [
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
'b',
|
|
['c', [
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' }
|
|
]],
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': '<b><i><u>a</u></i></b>b<u><b><i>c</i></b></u>'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
'a',
|
|
'b',
|
|
'c',
|
|
['d', [
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
['e', [
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' }
|
|
]],
|
|
['f', [
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' }
|
|
]],
|
|
'g',
|
|
'h',
|
|
'i',
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': 'abc<b><i><u>def</u></i></b>ghi'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
'a',
|
|
'b',
|
|
'c',
|
|
['d', [
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
['e', [
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
['f', [
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' }
|
|
]],
|
|
'g',
|
|
'h',
|
|
'i',
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': 'abc<b><i><u>d</u></i></b><i><u>e<b>f</b></u></i>ghi'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
'a',
|
|
'b',
|
|
'c',
|
|
['d', [
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' }
|
|
]],
|
|
['e', [
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
['f', [
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/italic' }
|
|
]],
|
|
'g',
|
|
'h',
|
|
'i',
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': 'abc<i><u><b>d</b>e<b>f</b></u></i>ghi'
|
|
},
|
|
{
|
|
'data': [
|
|
{ 'type': 'paragraph' },
|
|
'a',
|
|
'b',
|
|
'c',
|
|
['d', [
|
|
{ 'type': 'textStyle/italic' },
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' }
|
|
]],
|
|
['e', [
|
|
{ 'type': 'textStyle/bold' },
|
|
{ 'type': 'textStyle/underline' }
|
|
]],
|
|
['f', [
|
|
{ 'type': 'textStyle/underline' },
|
|
{ 'type': 'textStyle/bold' }
|
|
]],
|
|
'g',
|
|
'h',
|
|
'i',
|
|
{ 'type': '/paragraph' }
|
|
],
|
|
'html': 'abc<i><u><b>d</b></u></i><u><b>ef</b></u>ghi'
|
|
},
|
|
{
|
|
// [ ]
|
|
'data': [{ 'type': 'paragraph' },' ',{ 'type': '/paragraph' }],
|
|
'html': ' '
|
|
},
|
|
{
|
|
// [ ][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' '
|
|
},
|
|
{
|
|
// [ ][ ][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' '
|
|
},
|
|
{
|
|
// [ ][ ][ ][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ', ' ', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' '
|
|
},
|
|
{
|
|
// [ ][ ][ ][ ][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ', ' ', ' ', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' '
|
|
},
|
|
{
|
|
// [ ][ ][ ][ ][ ][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ', ' ', ' ', ' ', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' '
|
|
},
|
|
{
|
|
// [ ][A][ ][ ][ ][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', 'A', ' ', ' ', ' ', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' A '
|
|
},
|
|
{
|
|
// [ ][ ][A][ ][ ][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ', 'A', ' ', ' ', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' A '
|
|
},
|
|
{
|
|
// [ ][ ][ ][A][ ][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ', ' ', 'A', ' ', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' A '
|
|
},
|
|
{
|
|
// [ ][ ][ ][ ][A][ ]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ', ' ', ' ', 'A', ' ',{ 'type': '/paragraph' }],
|
|
'html': ' A '
|
|
},
|
|
{
|
|
// [ ][ ][ ][ ][ ][A]
|
|
'data': [{ 'type': 'paragraph' },' ', ' ', ' ', ' ', ' ', 'A',{ 'type': '/paragraph' }],
|
|
'html': ' A'
|
|
}
|
|
];
|
|
expect( cases.length );
|
|
for ( i = 0, len = cases.length; i < len; i++ ) {
|
|
ve.dm.example.preprocessAnnotations( cases[i].data );
|
|
assert.equal(
|
|
( new ve.ce.TextNode(
|
|
( new ve.dm.Document( cases[i].data ) )
|
|
.documentNode.getChildren()[0].getChildren()[0] )
|
|
).getHtml(),
|
|
cases[i].html
|
|
);
|
|
}
|
|
} );
|