mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
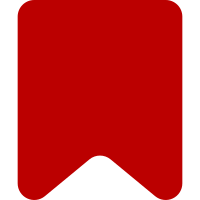
* Document them consistently between secetions Inheritance and Static Properties under their own (new) section Events. * Removed any quotes or brackets around the event name in existing @emit annotations Search: @emit.*['"{}(){}] * For every call to this.emit() anywhere, added @emits. * Fixed all warnings for references to undefined events (introduced as a result of the previous point). Event handler parameter documented based on the emit() call and actual handlers using the event. Usually the latter is more elaborate. * Extend coverage of jQuery as needed (copied from mwcore/maintenance/jsduck/external.js written by me, hereby implicitly and explicitly released under MIT). Specifics * ve.ce.Surface#onContentChange: Fixed type of range from Object to ve.Range. * ve.ce.SurfaceObserver#poll: Fix syntax for code from {} to `backticks`. * ve.ui.Toolbar#onContextChange: Doesn't actually emit "clearState" event. Removed #onClearState in Tool, ButtonTool and DropdownTool. Bug: 45872 Change-Id: Id879aa769b2c72d86a0322e75dddeb868211ce28
109 lines
2.6 KiB
JavaScript
109 lines
2.6 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface Toolbar class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* UserInterface toolbar.
|
|
*
|
|
* @class
|
|
* @extends ve.EventEmitter
|
|
* @constructor
|
|
* @param {jQuery} $container
|
|
* @param {ve.Surface} surface
|
|
* @param {Array} config
|
|
*/
|
|
ve.ui.Toolbar = function VeUiToolbar( $container, surface, config ) {
|
|
// Parent constructor
|
|
ve.EventEmitter.call( this );
|
|
|
|
// Properties
|
|
this.surface = surface;
|
|
this.$ = $container;
|
|
this.$groups = $( '<div>' );
|
|
this.config = config || {};
|
|
|
|
// Events
|
|
this.surface.getModel().on( 'contextChange', ve.bind( this.onContextChange, this ) );
|
|
|
|
// Initialization
|
|
this.$groups.addClass( 've-ui-toolbarGroups' );
|
|
this.$.prepend( this.$groups );
|
|
this.setup();
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.Toolbar, ve.EventEmitter );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* @event updateState
|
|
* @see ve.dm.SurfaceFragment#getAnnotations
|
|
* @param {ve.dm.Node[]} nodes List of nodes covered by the current selection
|
|
* @param {ve.AnnotationSet} full Annotations that cover all of the current selection
|
|
* @param {ve.AnnotationSet} partial Annotations that cover some or all of the current selection
|
|
*/
|
|
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Gets the surface the toolbar controls.
|
|
*
|
|
* @method
|
|
* @returns {ve.Surface} Surface being controlled
|
|
*/
|
|
ve.ui.Toolbar.prototype.getSurface = function () {
|
|
return this.surface;
|
|
};
|
|
|
|
/**
|
|
* Handle context changes on the surface.
|
|
*
|
|
* @method
|
|
* @emits updateState
|
|
*/
|
|
ve.ui.Toolbar.prototype.onContextChange = function () {
|
|
var i, len, leafNodes,
|
|
fragment = this.surface.getModel().getFragment(),
|
|
nodes = [];
|
|
|
|
leafNodes = fragment.getLeafNodes();
|
|
for ( i = 0, len = leafNodes.length; i < len; i++ ) {
|
|
nodes.push( leafNodes[i].node );
|
|
}
|
|
this.emit( 'updateState', nodes, fragment.getAnnotations(), fragment.getAnnotations( true ) );
|
|
};
|
|
|
|
/**
|
|
* Initialize all tools and groups.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Toolbar.prototype.setup = function () {
|
|
var i, j, group, $group, tool;
|
|
for ( i = 0; i < this.config.length; i++ ) {
|
|
group = this.config[i];
|
|
// Create group
|
|
$group = $( '<div class="ve-ui-toolbarGroup"></div>' )
|
|
.addClass( 've-ui-toolbarGroup-' + group.name );
|
|
if ( group.label ) {
|
|
$group.append( $( '<div class="ve-ui-toolbarLabel"></div>' ).html( group.label ) );
|
|
}
|
|
// Add tools
|
|
for ( j = 0; j < group.items.length; j++ ) {
|
|
tool = ve.ui.toolFactory.create( group.items[j], this );
|
|
if ( !tool ) {
|
|
throw new Error( 'Unknown tool: ' + group.items[j] );
|
|
}
|
|
$group.append( tool.$ );
|
|
}
|
|
// Append group
|
|
this.$groups.append( $group );
|
|
}
|
|
};
|