mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 03:08:42 +00:00
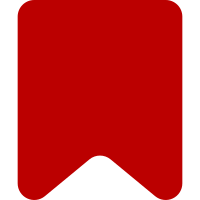
The sanity check converts the linear model back to DOM, then compares this DOM to the original DOM and rejects the deferred if they are not equal. The DOM creation has to be done synchronously (before we unlock the surface), but the actual comparsion can be (and is) done asynchronously. To make the UI flow of the save dialog easier we just keep the save button on the toolbar itself disabled until the sanity check is done. Though this should finish before the user starts editing the document (let alone start saving), we do add a class to the button to indicate a progress cursor. To simulate a slow sanity check, set the setTimeout in startSanityCheck to 5000, load VE, make a change, hover the button, and see it change from disabled + progress-cursor to enabled after 5 seconds. To simulate the sanity check failing, change !== to === in the first "if" in startSanityCheck. Bug: 47521 Bug: 50067 Change-Id: I04f71fe8e00c6257fbc953cc9de3323e24709b0f
116 lines
3.7 KiB
PHP
116 lines
3.7 KiB
PHP
<?php
|
|
/**
|
|
* Resource loader module for certain VisualEditor messages.
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Module for special messages VisualEditor needs to have parsed server side.
|
|
*/
|
|
class VisualEditorMessagesModule extends ResourceLoaderModule {
|
|
|
|
/* Protected Members */
|
|
|
|
protected $origin = self::ORIGIN_USER_SITEWIDE;
|
|
|
|
/* Methods */
|
|
|
|
public function getScript( ResourceLoaderContext $context ) {
|
|
$msgInfo = $this->getMessageInfo();
|
|
$parsedMesssages = array();
|
|
$messages = array();
|
|
foreach ( $msgInfo['args'] as $msgKey => $msgArgs ) {
|
|
$parsedMesssages[ $msgKey ] = call_user_func_array( 'wfMessage', $msgArgs )
|
|
->inLanguage( $context->getLanguage() )
|
|
->parse();
|
|
}
|
|
foreach ( $msgInfo['vals'] as $msgKey => $msgVal ) {
|
|
$messages[ $msgKey ] = $msgVal;
|
|
}
|
|
return
|
|
've.init.platform.addParsedMessages(' . FormatJson::encode( $parsedMesssages ) . ');'.
|
|
've.init.platform.addMessages(' . FormatJson::encode( $messages ) . ');';
|
|
}
|
|
|
|
protected function getMessageInfo() {
|
|
$msgKeys = array();
|
|
|
|
// Messages that just require simple parsing
|
|
$msgArgs = array(
|
|
'minoredit' => array( 'minoredit' ),
|
|
'watchthis' => array( 'watchthis' ),
|
|
'visualeditor-browserwarning' => array( 'visualeditor-browserwarning' ),
|
|
'visualeditor-report-notice' => array( 'visualeditor-report-notice' ),
|
|
'missingsummary' => array( 'missingsummary' ),
|
|
'visualeditor-savedialog-dirtywarning' => array( 'visualeditor-savedialog-dirtywarning' ),
|
|
);
|
|
|
|
// Override message value
|
|
$msgVals = array(
|
|
'visualeditor-feedback-link' => wfMessage( 'visualeditor-feedback-link' )
|
|
->inContentLanguage()
|
|
->text(),
|
|
);
|
|
|
|
// Copyright warning (based on EditPage::getCopyrightWarning)
|
|
global $wgRightsText;
|
|
if ( $wgRightsText ) {
|
|
$copywarnMsg = array( 'copyrightwarning',
|
|
'[[' . wfMessage( 'copyrightpage' )->inContentLanguage()->text() . ']]',
|
|
$wgRightsText );
|
|
} else {
|
|
$copywarnMsg = array( 'copyrightwarning2',
|
|
'[[' . wfMessage( 'copyrightpage' )->inContentLanguage()->text() . ']]' );
|
|
}
|
|
// EditPage supports customisation based on title, we can't support that here
|
|
// since these messages are cached on a site-level. $wgTitle is likely set to null.
|
|
$title = Title::newFromText( 'Dwimmerlaik' );
|
|
wfRunHooks( 'EditPageCopyrightWarning', array( $title, &$copywarnMsg ) );
|
|
|
|
// Keys used in copyright warning
|
|
$msgKeys[] = 'copyrightpage';
|
|
$msgKeys[] = $copywarnMsg[0];
|
|
// Normalise to 'copyrightwarning' so we have a consistent key in the front-end.
|
|
$msgArgs[ 'copyrightwarning' ] = $copywarnMsg;
|
|
|
|
$msgKeys = array_values( array_unique( array_merge(
|
|
$msgKeys,
|
|
array_keys( $msgArgs ),
|
|
array_keys( $msgVals )
|
|
) ) );
|
|
|
|
return array(
|
|
'keys' => $msgKeys,
|
|
'args' => $msgArgs,
|
|
'vals' => $msgVals,
|
|
);
|
|
}
|
|
|
|
public function getMessages() {
|
|
// We don't actually use the client-side message system for these messages.
|
|
// But we're registering them in this standardised method to make use of the
|
|
// getMsgBlobMtime utility to make cache invalidation work out-of-the-box.
|
|
|
|
$msgInfo = $this->getMessageInfo();
|
|
return $msgInfo['keys'];
|
|
}
|
|
|
|
public function getDependencies() {
|
|
return array( 'ext.visualEditor.base' );
|
|
}
|
|
|
|
public function getModifiedTime( ResourceLoaderContext $context ) {
|
|
return max(
|
|
$this->getMsgBlobMtime( $context->getLanguage() ),
|
|
// Also invalidate this module if this file changes (i.e. when messages were
|
|
// added or removed, or when the Javascript invocation in getScript is changes).
|
|
// Use 1 because 0 = now, would invalidate continously
|
|
file_exists( __FILE__ ) ? filemtime( __FILE__ ) : 1
|
|
);
|
|
}
|
|
}
|