mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 11:16:51 +00:00
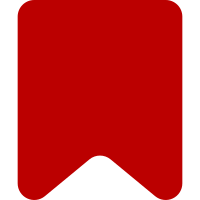
Created an IndexValueStore class which can store any object and return an integer index to its hash map. Linear data is now stored in ve.dm.LinearData instances. Two subclasses for element and meta data contain methods specific to those data types (ElementLinearData and MetaLinearData). The static methods in ve.dm.Document that inspected data at a given offset are now instance methods of ve.dm.ElementLinearData. AnnotationSets (which are no longer OrderedHashSets) have been moved to /dm and also have to be instantiated with a pointer the store. Bug: 46320 Change-Id: I249a5d48726093d1cb3e36351893f4bff85f52e2
72 lines
1.9 KiB
JavaScript
72 lines
1.9 KiB
JavaScript
/*!
|
|
* VisualEditor MetaLinearData class.
|
|
*
|
|
* Class containing meta linear data and an index-value store.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Meta linear data storage
|
|
*
|
|
* @class
|
|
* @extends ve.dm.LinearData
|
|
* @constructor
|
|
* @param {ve.dm.IndexValueStore} store Index-value store
|
|
* @param {Array} [data] Linear data
|
|
*/
|
|
ve.dm.MetaLinearData = function VeDmMetaLinearData( store, data ) {
|
|
ve.dm.LinearData.call( this, store, data );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.dm.MetaLinearData, ve.dm.LinearData );
|
|
|
|
/**
|
|
* Gets linear data from specified index(es).
|
|
*
|
|
* If either index is omitted the array at that point is returned
|
|
*
|
|
* @method
|
|
* @param {number} [offset] Offset to get data from
|
|
* @param {number} [metadataOffset] Index to get data from
|
|
* @returns {Object|Array} Data from index(es), or all data (by reference)
|
|
*/
|
|
ve.dm.MetaLinearData.prototype.getData = function ( offset, metadataOffset ) {
|
|
if ( offset === undefined ) {
|
|
return this.data;
|
|
} else if ( metadataOffset === undefined ) {
|
|
return this.data[offset];
|
|
} else {
|
|
return this.data[offset] === undefined ? undefined : this.data[offset][metadataOffset];
|
|
}
|
|
};
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Gets number of metadata elements at specified offset.
|
|
*
|
|
* @method
|
|
* @param {number} offset Offset to count metadata at
|
|
* @returns {number} Number of metadata elements at specified offset
|
|
*/
|
|
ve.dm.MetaLinearData.prototype.getDataLength = function ( offset ) {
|
|
return this.data[offset] === undefined ? 0 : this.data[offset].length;
|
|
};
|
|
|
|
/**
|
|
* Gets number of metadata elements in the entire object.
|
|
*
|
|
* @method
|
|
* @returns {number} Number of metadata elements in the entire object
|
|
*/
|
|
ve.dm.MetaLinearData.prototype.getTotalDataLength = function () {
|
|
var n = 0, i = this.getLength();
|
|
while ( i-- ) {
|
|
n += this.getDataLength( i );
|
|
}
|
|
return n;
|
|
}; |