mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
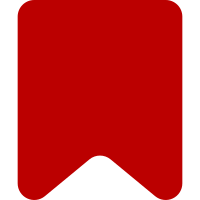
This replaces I8cf9ecd. Significant changes: * The …OutlineContainerWidget doesn't need to know the BookletLayout any more. The only remaining resason to have this dependency was some focus management. This is now done via an event. * Renamed an existing event to match the new one. The two really mean and do the exact same, even if they are triggered from two different places. * Simplified some existing code. * Updated documentation. Bug: T288827 Change-Id: Ifcf2cadabf7fa4b8ecb72e3937003fab3b00d9bb
134 lines
4.1 KiB
JavaScript
134 lines
4.1 KiB
JavaScript
/*!
|
|
* VisualEditor user interface MWTransclusionOutlineContainerWidget class.
|
|
*
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Container for transclusion, may contain a single or multiple templates.
|
|
*
|
|
* @class
|
|
* @extends OO.ui.Widget
|
|
*
|
|
* @constructor
|
|
* @property {Object.<string,ve.ui.MWTransclusionOutlinePartWidget>} partWidgets Map of top-level
|
|
* items currently visible in this container, indexed by part id
|
|
*/
|
|
ve.ui.MWTransclusionOutlineContainerWidget = function VeUiMWTransclusionOutlineContainerWidget() {
|
|
// Parent constructor
|
|
ve.ui.MWTransclusionOutlineContainerWidget.super.call( this );
|
|
|
|
// Initialization
|
|
this.partWidgets = {};
|
|
|
|
this.$element.addClass( 've-ui-mwTransclusionOutlineContainerWidget' );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWTransclusionOutlineContainerWidget, OO.ui.Widget );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* @event filterParameters
|
|
* @param {Object.<string,boolean>} visibility Keyed by unique id of the parameter, e.g. something
|
|
* like "part_1/param1". Note this lists only parameters that are currently in use.
|
|
*/
|
|
|
|
/**
|
|
* @event focusPart
|
|
* @param {string} partId Unique id of the part, e.g. something "part_1" or "part_1/param1".
|
|
*/
|
|
|
|
/**
|
|
* @param {ve.dm.MWTransclusionPartModel|null} removed Removed part
|
|
* @param {ve.dm.MWTransclusionPartModel|null} added Added part
|
|
* @param {number} [newPosition]
|
|
*/
|
|
ve.ui.MWTransclusionOutlineContainerWidget.prototype.onReplacePart = function ( removed, added, newPosition ) {
|
|
if ( removed ) {
|
|
this.removePartWidget( removed );
|
|
}
|
|
// TODO: reselect if active part was in a removed template
|
|
|
|
if ( added ) {
|
|
this.addPartWidget( added, newPosition );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* @param {ve.dm.MWTransclusionModel} transclusionModel
|
|
*/
|
|
ve.ui.MWTransclusionOutlineContainerWidget.prototype.onTransclusionModelChange = function ( transclusionModel ) {
|
|
var newOrder = transclusionModel.getParts();
|
|
|
|
for ( var i = 0; i < newOrder.length; i++ ) {
|
|
var expectedWidget = this.partWidgets[ newOrder[ i ].getId() ],
|
|
$expectedElement = expectedWidget && expectedWidget.$element,
|
|
$currentElement = this.$element.children().eq( i );
|
|
|
|
if ( !$currentElement.is( $expectedElement ) ) {
|
|
// Move each widget to the correct position if it wasn't there before
|
|
$currentElement.before( $expectedElement );
|
|
}
|
|
}
|
|
};
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* @private
|
|
* @param {ve.dm.MWTransclusionPartModel} part
|
|
*/
|
|
ve.ui.MWTransclusionOutlineContainerWidget.prototype.removePartWidget = function ( part ) {
|
|
var partId = part.getId(),
|
|
widget = this.partWidgets[ partId ];
|
|
|
|
if ( widget ) {
|
|
widget.$element.remove();
|
|
delete this.partWidgets[ partId ];
|
|
}
|
|
};
|
|
|
|
/**
|
|
* @private
|
|
* @param {ve.dm.MWTransclusionPartModel} part
|
|
* @param {number} [newPosition]
|
|
*/
|
|
ve.ui.MWTransclusionOutlineContainerWidget.prototype.addPartWidget = function ( part, newPosition ) {
|
|
var widget;
|
|
|
|
if ( part instanceof ve.dm.MWTemplateModel ) {
|
|
widget = new ve.ui.MWTransclusionOutlineTemplateWidget( part );
|
|
// This forwards events from the nested ve.ui.MWTransclusionOutlineTemplateWidget upwards.
|
|
// The array syntax is a way to call `this.emit( 'filterParameters' )`.
|
|
widget.connect( this, { filterParameters: [ 'emit', 'filterParameters' ] } );
|
|
} else if ( part instanceof ve.dm.MWTemplatePlaceholderModel ) {
|
|
widget = new ve.ui.MWTransclusionOutlinePlaceholderWidget( part );
|
|
} else if ( part instanceof ve.dm.MWTransclusionContentModel ) {
|
|
widget = new ve.ui.MWTransclusionOutlineWikitextWidget( part );
|
|
}
|
|
|
|
widget.connect( this, { focusPart: [ 'emit', 'focusPart' ] } );
|
|
|
|
this.partWidgets[ part.getId() ] = widget;
|
|
if ( typeof newPosition === 'number' && newPosition < this.$element.children().length ) {
|
|
this.$element.children().eq( newPosition ).before( widget.$element );
|
|
} else {
|
|
this.$element.append( widget.$element );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Removes all {@see ve.ui.MWTransclusionOutlinePartWidget}, i.e. empties the list.
|
|
*/
|
|
ve.ui.MWTransclusionOutlineContainerWidget.prototype.clear = function () {
|
|
for ( var partId in this.partWidgets ) {
|
|
var widget = this.partWidgets[ partId ];
|
|
widget.disconnect( this );
|
|
widget.$element.remove();
|
|
}
|
|
this.partWidgets = {};
|
|
};
|