mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-28 12:46:45 +00:00
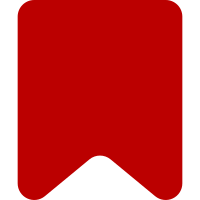
Objectives: * Got rid of mw prefixing in tools, inspectors and dialogs * Simplify tool classes so they can be generically used as items in bars, lists and menus * Add support for a catch-all toolbar group * Simplify tool registration, leaning on tool classes' static name property * Move default commands to command registry * Move default triggers to trigger registry * Get language tool working in standalone Change-Id: Ic97a636f9a193374728629931b6702bee1b3416a
76 lines
1.6 KiB
JavaScript
76 lines
1.6 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface LabeledElement class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Labeled element.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
*
|
|
* @constructor
|
|
* @param {jQuery} $label Label element
|
|
* @param {Object} [config] Config options
|
|
* @cfg {jQuery|string} [label=''] Label text
|
|
*/
|
|
ve.ui.LabeledElement = function VeUiLabeledElement( $label, config ) {
|
|
// Config intialization
|
|
config = config || {};
|
|
|
|
// Properties
|
|
this.$label = $label;
|
|
this.label = null;
|
|
|
|
// Initialization
|
|
this.$label.addClass( 've-ui-labeledElement-label' );
|
|
this.setLabel( config.label );
|
|
};
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Set the label.
|
|
*
|
|
* @method
|
|
* @param {jQuery|string} [value] jQuery HTML node selection or string text value to use for label
|
|
* @chainable
|
|
*/
|
|
ve.ui.LabeledElement.prototype.setLabel = function ( value ) {
|
|
if ( typeof value === 'string' && value.trim() ) {
|
|
this.$label.text( value );
|
|
this.label = value;
|
|
} else if ( value instanceof jQuery ) {
|
|
this.$label.empty().append( value );
|
|
this.label = value;
|
|
} else {
|
|
this.$label.html( ' ' );
|
|
this.label = null;
|
|
}
|
|
return this;
|
|
};
|
|
|
|
/**
|
|
* Get label value as plain text.
|
|
*
|
|
* @return {string} Label text
|
|
*/
|
|
ve.ui.LabeledElement.prototype.getLabelText = function () {
|
|
return this.$label.text();
|
|
};
|
|
|
|
/**
|
|
* Fit the label.
|
|
*
|
|
* @method
|
|
* @chainable
|
|
*/
|
|
ve.ui.LabeledElement.prototype.fitLabel = function () {
|
|
if ( this.$label.autoEllipsis ) {
|
|
this.$label.autoEllipsis( { 'hasSpan': false, 'tooltip': true } );
|
|
}
|
|
return this;
|
|
};
|