mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
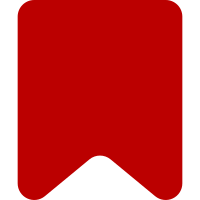
The "mediaClass" property now only serves to capture the original class found on the media so that it can be roundtripped without causing dirty diffs. In the 2.4.0 version of Parsoid's output, that will still be the usual Image/Audio/Video. As of 2.5.0, it will always be File and the mediaClass property can be dropped. Parsoid is currently forward compatible with serializing mw:File, so edited or new media can use that type already. The contextmenu item for media has been updated to make use of the "mediaTag" instead of mediaClass to continue distinguishing media types. That was the only place a grep of mediaClass turned up any use. Bug: T273505 Change-Id: If5dc6b794dacd6973d3b2093e6b385591b91d539
89 lines
2.2 KiB
JavaScript
89 lines
2.2 KiB
JavaScript
/*!
|
|
* VisualEditor MWMediaContextItem class.
|
|
*
|
|
* @copyright 2011-2017 VisualEditor Team and others; see http://ve.mit-license.org
|
|
*/
|
|
|
|
/**
|
|
* Context item for a MWImageNode.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.LinearContextItem
|
|
*
|
|
* @constructor
|
|
* @param {ve.ui.Context} context Context item is in
|
|
* @param {ve.dm.Model} model Model item is related to
|
|
* @param {Object} config Configuration options
|
|
*/
|
|
ve.ui.MWMediaContextItem = function VeUiMWMediaContextItem( context, model ) {
|
|
// Parent constructor
|
|
ve.ui.MWMediaContextItem.super.apply( this, arguments );
|
|
|
|
// Initialization
|
|
this.$element.addClass( 've-ui-mwMediaContextItem' );
|
|
|
|
var mediaTag = model.getAttribute( 'mediaTag' ) || 'img';
|
|
|
|
this.setIcon( {
|
|
img: 'image',
|
|
span: 'imageBroken',
|
|
// TODO: Better icons for audio/video
|
|
audio: 'play',
|
|
video: 'play'
|
|
}[ mediaTag ] );
|
|
|
|
var messagePostfix = ( mediaTag === 'audio' || mediaTag === 'video' ) ? mediaTag : 'image';
|
|
|
|
// The following messages are used here:
|
|
// * visualeditor-media-title-audio
|
|
// * visualeditor-media-title-image
|
|
// * visualeditor-media-title-video
|
|
this.setLabel( ve.msg( 'visualeditor-media-title-' + messagePostfix ) );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWMediaContextItem, ve.ui.LinearContextItem );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.ui.MWMediaContextItem.static.name = 'mwMedia';
|
|
|
|
ve.ui.MWMediaContextItem.static.icon = 'image';
|
|
|
|
ve.ui.MWMediaContextItem.static.label =
|
|
OO.ui.deferMsg( 'visualeditor-media-title-image' );
|
|
|
|
ve.ui.MWMediaContextItem.static.modelClasses = [ ve.dm.MWBlockImageNode, ve.dm.MWInlineImageNode ];
|
|
|
|
ve.ui.MWMediaContextItem.static.commandName = 'media';
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWMediaContextItem.prototype.getDescription = function () {
|
|
return ve.ce.nodeFactory.getDescription( this.model );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWMediaContextItem.prototype.renderBody = function () {
|
|
var title = mw.Title.newFromText( mw.libs.ve.normalizeParsoidResourceName( this.model.getAttribute( 'resource' ) ) );
|
|
this.$body.append(
|
|
$( '<a>' )
|
|
.text( this.getDescription() )
|
|
.attr( {
|
|
href: title.getUrl(),
|
|
target: '_blank',
|
|
rel: 'noopener'
|
|
} )
|
|
);
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.ui.contextItemFactory.register( ve.ui.MWMediaContextItem );
|