mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-24 18:58:42 +00:00
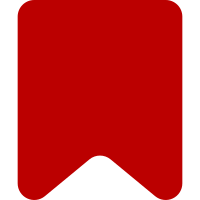
* Document them consistently between secetions Inheritance and Static Properties under their own (new) section Events. * Removed any quotes or brackets around the event name in existing @emit annotations Search: @emit.*['"{}(){}] * For every call to this.emit() anywhere, added @emits. * Fixed all warnings for references to undefined events (introduced as a result of the previous point). Event handler parameter documented based on the emit() call and actual handlers using the event. Usually the latter is more elaborate. * Extend coverage of jQuery as needed (copied from mwcore/maintenance/jsduck/external.js written by me, hereby implicitly and explicitly released under MIT). Specifics * ve.ce.Surface#onContentChange: Fixed type of range from Object to ve.Range. * ve.ce.SurfaceObserver#poll: Fix syntax for code from {} to `backticks`. * ve.ui.Toolbar#onContextChange: Doesn't actually emit "clearState" event. Removed #onClearState in Tool, ButtonTool and DropdownTool. Bug: 45872 Change-Id: Id879aa769b2c72d86a0322e75dddeb868211ce28
73 lines
1.6 KiB
JavaScript
73 lines
1.6 KiB
JavaScript
/*!
|
|
* VisualEditor Registry class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Generic data registry.
|
|
*
|
|
* @abstract
|
|
* @extends ve.EventEmitter
|
|
* @constructor
|
|
*/
|
|
ve.Registry = function VeRegistry() {
|
|
// Parent constructor
|
|
ve.EventEmitter.call( this );
|
|
|
|
// Properties
|
|
this.registry = {};
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.Registry, ve.EventEmitter );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* @event register
|
|
* @param {string} name
|
|
* @param {Mixed} data
|
|
*/
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Associate one or more symbolic names with some data.
|
|
*
|
|
* @method
|
|
* @param {string|string[]} name Symbolic name or list of symbolic names
|
|
* @param {Mixed} data Data to associate with symbolic name
|
|
* @emits register
|
|
* @throws {Error} Name argument must be a string or array
|
|
*/
|
|
ve.Registry.prototype.register = function ( name, data ) {
|
|
if ( typeof name !== 'string' && !ve.isArray( name ) ) {
|
|
throw new Error( 'Name argument must be a string or array, cannot be a ' + typeof name );
|
|
}
|
|
var i, len;
|
|
if ( ve.isArray( name ) ) {
|
|
for ( i = 0, len = name.length; i < len; i++ ) {
|
|
this.register( name[i], data );
|
|
}
|
|
} else if ( typeof name === 'string' ) {
|
|
this.registry[name] = data;
|
|
this.emit( 'register', name, data );
|
|
} else {
|
|
throw new Error( 'Name must be a string or array of strings, cannot be a ' + typeof name );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Gets data for a given symbolic name.
|
|
*
|
|
* @method
|
|
* @param {string} name Symbolic name
|
|
* @returns {Mixed|undefined} Data associated with symbolic name
|
|
*/
|
|
ve.Registry.prototype.lookup = function ( name ) {
|
|
return this.registry[name];
|
|
};
|