mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-12-04 18:58:37 +00:00
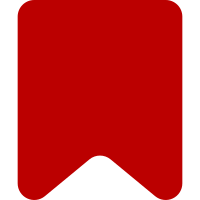
What: Add a hook that runs before a save attempt is made in ApiVisualEditorEdit. The hook receives the same data available in ApiVisualEditorEdit, and implementations of the hook can modify the API response. Why: VE plugins may send additional data when saving an edit, and extensions might want to prevent the save from taking place based on that additional data. See for example the AddLink plugin in Ic8225933c9, where the save is blocked if link suggestions don't exist in the database at save time. Bug: T283109 Change-Id: I6b81ea318f52ec47661086d85b5cc242a3fcd0e4
53 lines
2.1 KiB
PHP
53 lines
2.1 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\VisualEditor;
|
|
|
|
use MediaWiki\Page\ProperPageIdentity;
|
|
use MediaWiki\User\UserIdentity;
|
|
|
|
/**
|
|
* VisualEditorApiVisualEditorEditPreSaveHook
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @copyright 2011-2021 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license MIT
|
|
*/
|
|
|
|
interface VisualEditorApiVisualEditorEditPreSaveHook {
|
|
|
|
/**
|
|
* This hook is executed in calls to ApiVisualEditorEdit using action=save, before the save is attempted.
|
|
*
|
|
* This hook can abort the save attempt by returning false.
|
|
*
|
|
* @param ProperPageIdentity $page The page identity of the title used in the save attempt.
|
|
* @param UserIdentity $user User associated with the save attempt.
|
|
* @param string $wikitext The wikitext used in the save attempt.
|
|
* @param array $params The params passed by the client in the API request. See
|
|
* ApiVisualEditorEdit::getAllowedParams()
|
|
* @param array $pluginData Associative array containing additional data specified by plugins, where the keys of
|
|
* the array are plugin names, and the value are arbitrary data. Plugins are expected to be in a one-to-one
|
|
* correlation with hook handlers and can be specified via the 'plugins' and 'data-*' parameters of the API.
|
|
* @param array &$apiResponse The modifiable API response that VisualEditor will return to the client.
|
|
* There are several keys that are used by VisualEditor and will be overwritten if set here, notable ones include
|
|
* "result" and "edit". See ApiVisualEditorEdit::execute().
|
|
* Note: When returning false, the "message" key must be set to a valid i18n message key, e.g.
|
|
* ```php
|
|
* $apiResponse['message'] = [ 'growthexperiments-addlink-notinstore', $title->getPrefixedText() ];
|
|
* return false;
|
|
* @return void|bool
|
|
* False will abort the save attempt and return an error to the client. If false is returned, the implementer
|
|
* must also set the "message" key in $apiResponse for rendering the error response to the client.
|
|
*/
|
|
public function onVisualEditorApiVisualEditorEditPreSave(
|
|
ProperPageIdentity $page,
|
|
UserIdentity $user,
|
|
string $wikitext,
|
|
array $params,
|
|
array $pluginData,
|
|
array &$apiResponse
|
|
);
|
|
|
|
}
|