mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
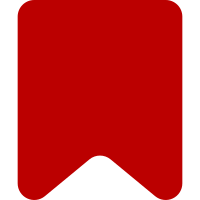
New changes: 56de6f5 Localisation updates from https://translatewiki.net. f8bda64 Widgetise demo menu 6ac48d8 Localisation updates from https://translatewiki.net. 365e131 builderloader: Omit value for boolean "disabled" attribute per HTML5 706e4b3 Prevent double counting of DM nodes in getNodeAndOffset b141a7d Update OOjs UI to v0.1.0-pre (d2451ac748) c5b3921 Localisation updates from https://translatewiki.net. 1606983 Update reference to ConfirmationDialog to use MessageDialog Deletions: * Styles for ve.ui.MWBetaWelcomeDialog - not needed anymore because OO.ui.MessageDialog provides them * Styles for ve.ui.MWGalleryInspector - not needed anymore because ve.ui.MWExtensionInspector provides part of them and the rest are being replaced by programatic sizing Modifications: * ve.ui.MWLinkTargetInputWidget - Added support for validation and href getter * Split message between tool and dialog title for ve.ui.MWEditModeTool and ve.ui.MWWikitextSwitchConfirmDialog General changes: * Updated inheritance. * Added manager param to constructors of dialogs and inspectors. * Updated use of show/hide with toggle. * Added meaningful descriptions of dialog and inspector classes. * Configured dialog and inspector sizes statically. * Configured dialog action buttons statically. * Interfaced with OO.ui.ActionSet to control action buttons. * Moved applyChanges code into getActionProcess methods. * Always using .next in setup/ready process getters and .first in hold/teardown process getters. Change-Id: Ia74732e6e32c0808eee021f0a26225b9e6c3f971
178 lines
4.7 KiB
JavaScript
178 lines
4.7 KiB
JavaScript
/*!
|
|
* VisualEditor user interface MWReferenceListDialog class.
|
|
*
|
|
* @copyright 2011-2014 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Dialog for inserting and editing MediaWiki reference lists.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.NodeDialog
|
|
*
|
|
* @constructor
|
|
* @param {OO.ui.WindowManager} manager Manager of window
|
|
* @param {Object} [config] Configuration options
|
|
*/
|
|
ve.ui.MWReferenceListDialog = function VeUiMWReferenceListDialog( manager, config ) {
|
|
// Parent constructor
|
|
ve.ui.MWReferenceListDialog.super.call( this, manager, config );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWReferenceListDialog, ve.ui.NodeDialog );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.ui.MWReferenceListDialog.static.name = 'referenceList';
|
|
|
|
ve.ui.MWReferenceListDialog.static.title =
|
|
OO.ui.deferMsg( 'visualeditor-dialog-referencelist-title' );
|
|
|
|
ve.ui.MWReferenceListDialog.static.icon = 'references';
|
|
|
|
ve.ui.MWReferenceListDialog.static.modelClasses = [ ve.dm.MWReferenceListNode ];
|
|
|
|
ve.ui.MWReferenceListDialog.static.size = 'medium';
|
|
|
|
ve.ui.MWReferenceListDialog.static.actions = [
|
|
{
|
|
'action': 'apply',
|
|
'label': OO.ui.deferMsg( 'visualeditor-dialog-action-apply' ),
|
|
'flags': 'primary',
|
|
'modes': 'edit'
|
|
},
|
|
{
|
|
'action': 'insert',
|
|
'label': OO.ui.deferMsg( 'visualeditor-dialog-referencelist-insert-button' ),
|
|
'flags': [ 'primary', 'constructive' ],
|
|
'modes': 'insert'
|
|
},
|
|
{
|
|
'label': OO.ui.deferMsg( 'visualeditor-dialog-action-cancel' ),
|
|
'flags': 'safe',
|
|
'modes': [ 'insert', 'edit' ]
|
|
}
|
|
];
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWReferenceListDialog.prototype.getBodyHeight = function () {
|
|
return this.editPanel.$element[0].scrollHeight;
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWReferenceListDialog.prototype.initialize = function () {
|
|
// Parent method
|
|
ve.ui.MWReferenceListDialog.super.prototype.initialize.call( this );
|
|
|
|
// Properties
|
|
this.panels = new OO.ui.StackLayout( { '$': this.$ } );
|
|
this.editPanel = new OO.ui.PanelLayout( {
|
|
'$': this.$, 'scrollable': true, 'padded': true
|
|
} );
|
|
this.optionsFieldset = new OO.ui.FieldsetLayout( {
|
|
'$': this.$
|
|
} );
|
|
|
|
this.groupInput = new OO.ui.TextInputWidget( {
|
|
'$': this.$,
|
|
'placeholder': ve.msg( 'visualeditor-dialog-reference-options-group-placeholder' )
|
|
} );
|
|
this.groupField = new OO.ui.FieldLayout( this.groupInput, {
|
|
'$': this.$,
|
|
'align': 'top',
|
|
'label': ve.msg( 'visualeditor-dialog-reference-options-group-label' )
|
|
} );
|
|
|
|
// Initialization
|
|
this.optionsFieldset.addItems( [ this.groupField ] );
|
|
this.editPanel.$element.append( this.optionsFieldset.$element );
|
|
this.panels.addItems( [ this.editPanel ] );
|
|
this.$body.append( this.panels.$element );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWReferenceListDialog.prototype.getActionProcess = function ( action ) {
|
|
if ( action === 'apply' || action === 'insert' ) {
|
|
return new OO.ui.Process( function () {
|
|
var refGroup, listGroup, oldListGroup, attrChanges, doc,
|
|
surfaceModel = this.getFragment().getSurface();
|
|
|
|
// Save changes
|
|
refGroup = this.groupInput.getValue();
|
|
listGroup = 'mwReference/' + refGroup;
|
|
|
|
if ( this.selectedNode ) {
|
|
// Edit existing model
|
|
doc = surfaceModel.getDocument();
|
|
oldListGroup = this.selectedNode.getAttribute( 'listGroup' );
|
|
|
|
if ( listGroup !== oldListGroup ) {
|
|
attrChanges = {
|
|
listGroup: listGroup,
|
|
refGroup: refGroup
|
|
};
|
|
surfaceModel.change(
|
|
ve.dm.Transaction.newFromAttributeChanges(
|
|
doc, this.selectedNode.getOuterRange().start, attrChanges
|
|
)
|
|
);
|
|
}
|
|
} else {
|
|
// Collapse returns a new fragment, so update this.fragment
|
|
this.fragment = this.getFragment().collapseRangeToEnd().insertContent( [
|
|
{
|
|
'type': 'mwReferenceList',
|
|
'attributes': {
|
|
'listGroup': listGroup,
|
|
'refGroup': refGroup
|
|
}
|
|
},
|
|
{ 'type': '/mwReferenceList' }
|
|
] );
|
|
}
|
|
|
|
this.close( { 'action': action } );
|
|
}, this );
|
|
}
|
|
// Parent method
|
|
return ve.ui.MWReferenceListDialog.super.prototype.getActionProcess.call( this, action );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWReferenceListDialog.prototype.getSetupProcess = function ( data ) {
|
|
return ve.ui.MWReferenceListDialog.super.prototype.getSetupProcess.call( this, data )
|
|
.next( function () {
|
|
var node, refGroup;
|
|
|
|
// Prepopulate from existing node if we're editing a node
|
|
// instead of inserting a new one
|
|
node = this.getFragment().getSelectedNode();
|
|
if ( this.selectedNode instanceof ve.dm.MWReferenceListNode ) {
|
|
refGroup = node.getAttribute( 'refGroup' );
|
|
this.actions.setMode( 'edit' );
|
|
} else {
|
|
refGroup = '';
|
|
this.actions.setMode( 'insert' );
|
|
}
|
|
|
|
this.groupInput.setValue( refGroup );
|
|
}, this );
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.ui.windowFactory.register( ve.ui.MWReferenceListDialog );
|