mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-26 19:56:49 +00:00
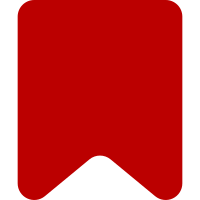
* Tokens are now immutable. The progress of transformations is tracked on chunks instead of tokens. Tokenizer output is cached and can be directly returned without a need for cloning. Transforms are required to clone or newly create tokens they are modifying. * Expansions per chunk are now shared between equivalent frames via a cache stored on the chunk itself. Equivalence of frames is not yet ideal though, as right now a hash tree of *unexpanded* arguments is used. This should be switched to a hash of the fully expanded local parameters instead. * There is now a vastly improved maybeSyncReturn wrapper for async transforms that either forwards processing to the iterative transformTokens if the current transform is still ongoing, or manages a recursive transformation if needed. * Parameters for parser functions are now wrapped in abstract Params and ParserValue objects, which support some handy on-demand *value* expansions. Keys are always expanded. Parser functions are converted to use these interfaces, and now properly expand their values in the correct frame. Making this expansion lazier is certainly possible, but would complicate transformTokens and other token-handling machinery. Need to investigate if it would really be worth it. Dead branch elimination is certainly a bigger win overall. * Complex recursive asynchronous expansions should now be closer to correct for both the iterative (transformTokens) and recursive (maybeSyncReturn after transformTokens has returned) code paths. * Performance degraded slightly. There are no micro-optimizations done yet and the shared expansion cache still has a low hit rate. The progress tracking on chunks is not yet perfect, so there are likely a lot of unneeded re-expansions that can be easily eliminated. There is also more debug tracing right now. Obama currently expands in 54 seconds on my laptop. Change-Id: I4a603f3d3c70ca657ebda9fbb8570269f943d6b6
24 lines
544 B
JavaScript
24 lines
544 B
JavaScript
/**
|
|
*/
|
|
|
|
function BehaviorSwitchHandler( manager, isInclude ) {
|
|
this.manager = manager;
|
|
this.manager.addTransform( this.onBehaviorSwitch.bind( this ), this.rank, 'tag', 'behavior-switch' );
|
|
}
|
|
|
|
BehaviorSwitchHandler.prototype.rank = 2.14;
|
|
|
|
BehaviorSwitchHandler.prototype.onBehaviorSwitch = function ( token, manager, cb ) {
|
|
var env = this.manager.env,
|
|
magic_word = token.attribs[0].v;
|
|
|
|
env.setVariable(magic_word, true);
|
|
|
|
return { };
|
|
};
|
|
|
|
|
|
if (typeof module == "object") {
|
|
module.exports.BehaviorSwitchHandler = BehaviorSwitchHandler;
|
|
}
|