mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 03:08:42 +00:00
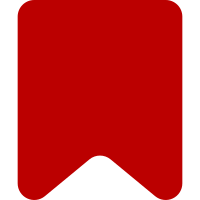
This has no influence on Jenkins but can be used locally to easily run certain tools. Since we already had `.jshintrc` in our repo it was already possible to easily run JSHint from the command-line locally. Taking that as a base the following are new features: * `grunt csslint`: Runs CSSLint on all css files * `grunt qunit`: Runs QUnit (standalone) tests in PhantomJS * `grunt test`: Runs jshint/csslint/qunit * `grunt watch`: Runs the "test" command automatically whenever a file is changed. You can keep this in the background so whenever you save a file in your editor (e.g. Sublime Text) it'll run the tests and if there is a failure, it'll throw a bash error code causing your Terminal application to beep you in whatever way your operating system does so (e.g. for Mac OS X a red badge + jumping icon in the Dock). It will continue to run in the background even after a failure so no need to re-start watch after a failure. * `grunt`: Runs the default task, which is 'test'. Previously to use `jshint .` you had to: * One-time install: * install package -- nodejs npm * npm install -g jshint * Usage: * cd VisualEditor; jshint . Now, for grunt: * One-time install: * install package -- nodejs npm * npm install -g grunt-cli * cd VisualEditor; npm install * Usage: * cd VisualEditor; grunt Change-Id: I7a4fdf4b6bf3f00cef15dc3e2c81eceb595aec7c
61 lines
1.6 KiB
JavaScript
61 lines
1.6 KiB
JavaScript
/*!
|
|
* Grunt file
|
|
*
|
|
* @package VisualEditor
|
|
*/
|
|
|
|
/*jshint node:true */
|
|
module.exports = function ( grunt ) {
|
|
var fs = require( 'fs' ),
|
|
exec = require( 'child_process' ).exec;
|
|
|
|
grunt.loadNpmTasks( 'grunt-contrib-jshint' );
|
|
grunt.loadNpmTasks( 'grunt-contrib-csslint' );
|
|
grunt.loadNpmTasks( 'grunt-contrib-qunit' );
|
|
grunt.loadNpmTasks( 'grunt-contrib-watch' );
|
|
|
|
grunt.initConfig( {
|
|
pkg: grunt.file.readJSON( 'package.json' ),
|
|
jshint: {
|
|
options: JSON.parse( grunt.file.read( '.jshintrc' )
|
|
.replace( /\/\*(?:(?!\*\/)[\s\S])*\*\//g, '' ).replace( /\/\/[^\n\r]*/g, '' ) ),
|
|
all: ['*.js', 'modules/**/*.js']
|
|
},
|
|
csslint: {
|
|
options: {
|
|
csslintrc: '.csslintrc'
|
|
},
|
|
all: ['modules/ve/**/*.css']
|
|
},
|
|
qunit: {
|
|
all: ['modules/ve/test/index-phantomjs-tmp.html']
|
|
},
|
|
watch: {
|
|
files: ['<%= jshint.all %>', '<%= csslint.all %>', '<%= qunit.all %>', '.{jshintrc,jshintignore,csslintrc}'],
|
|
tasks: ['test']
|
|
}
|
|
} );
|
|
|
|
grunt.registerTask( 'pre-qunit', function () {
|
|
var done = this.async();
|
|
grunt.file.setBase( __dirname + '/modules/ve/test' );
|
|
exec( 'php index.php > index-phantomjs-tmp.html', function ( err, stdout, stderr ) {
|
|
if ( err || stderr ) {
|
|
grunt.log.error( err || stderr );
|
|
done( false );
|
|
} else {
|
|
grunt.file.setBase( __dirname );
|
|
done( true );
|
|
}
|
|
} );
|
|
} );
|
|
|
|
grunt.registerTask( 'post-qunit', function () {
|
|
fs.unlinkSync( __dirname + '/modules/ve/test/index-phantomjs-tmp.html' );
|
|
return true;
|
|
} );
|
|
|
|
grunt.registerTask( 'test', ['jshint', 'csslint', 'pre-qunit', 'qunit', 'post-qunit'] );
|
|
grunt.registerTask( 'default', 'test' );
|
|
};
|