mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
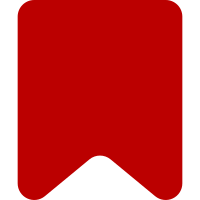
This is one of the blockers for splitting VE up into separate repositories or extending VE with an extension. ve.ui.Frame.js It's critical that we don't emit initialize from ve.ui.Frame until it's completely loaded, especially its styles, because we will begin measuring it straight away. Involved loading the stylesheets using $.ajax and setting base URL of the iframe to the ve.ui styles directory so all the image URLs still worked. This won't work for stylesheets from multiple locations, so we needed a more robust solution. The new solution uses some trickery described in the code documentation, but essentially no longer depends on all stylesheets being located in the same folder. ve.ui.Dialog.js, ve.ui.Inspector.js, ve.ui.Window.js Static methods are now being used to extend a window class to include different stylesheets rather than simple array concatenation. Change-Id: I619238732f975d41305f81f8f818a577a40f49da
145 lines
3.2 KiB
JavaScript
145 lines
3.2 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface Inspector class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* UserInterface inspector.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.Window
|
|
*
|
|
* @constructor
|
|
* @param {ve.Surface} surface
|
|
*/
|
|
ve.ui.Inspector = function VeUiInspector( surface ) {
|
|
// Inheritance
|
|
ve.ui.Window.call( this, surface );
|
|
|
|
// Properties
|
|
this.initialSelection = null;
|
|
|
|
// Initialization
|
|
this.$.addClass( 've-ui-inspector' );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.Inspector, ve.ui.Window );
|
|
|
|
/* Static Properties */
|
|
|
|
/**
|
|
* Pattern to use when matching against annotation type strings.
|
|
*
|
|
* @static
|
|
* @property {RegExp}
|
|
*/
|
|
ve.ui.Inspector.static.typePattern = new RegExp();
|
|
|
|
ve.ui.Inspector.static.titleMessage = 've-ui-inspector-title';
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Handle frame ready events.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Inspector.prototype.initialize = function () {
|
|
// Call parent method
|
|
ve.ui.Window.prototype.initialize.call( this );
|
|
|
|
// Initialization
|
|
this.$form = this.$$( '<form>' );
|
|
this.closeButton = new ve.ui.IconButtonWidget( {
|
|
'$$': this.$$, 'icon': 'previous', 'title': ve.msg( 'visualeditor-inspector-close-tooltip' )
|
|
} );
|
|
this.removeButton = new ve.ui.IconButtonWidget( {
|
|
'$$': this.$$, 'icon': 'remove', 'title': ve.msg( 'visualeditor-inspector-remove-tooltip' )
|
|
} );
|
|
|
|
// Events
|
|
this.$form.on( {
|
|
'submit': ve.bind( this.onFormSubmit, this ),
|
|
'keydown': ve.bind( this.onFormKeyDown, this )
|
|
} );
|
|
this.closeButton.on( 'click', ve.bind( this.onCloseButtonClick, this ) );
|
|
this.removeButton.on( 'click', ve.bind( this.onRemoveButtonClick, this ) );
|
|
|
|
// Initialization
|
|
this.closeButton.$.addClass( 've-ui-inspector-closeButton' );
|
|
this.removeButton.$.addClass( 've-ui-inspector-removeButton' );
|
|
this.$head.prepend( this.closeButton.$ ).append( this.removeButton.$ );
|
|
this.$body.append( this.$form );
|
|
};
|
|
|
|
/**
|
|
* Handle close button click events.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Inspector.prototype.onCloseButtonClick = function () {
|
|
this.close();
|
|
};
|
|
|
|
/**
|
|
* Handle remove button click events.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Inspector.prototype.onRemoveButtonClick = function() {
|
|
this.close( true );
|
|
};
|
|
|
|
/**
|
|
* Handle form submission events.
|
|
*
|
|
* @method
|
|
* @param {jQuery.Event} e Form submit event
|
|
*/
|
|
ve.ui.Inspector.prototype.onFormSubmit = function () {
|
|
this.close();
|
|
return false;
|
|
};
|
|
|
|
/**
|
|
* Handle form keydown events.
|
|
*
|
|
* @method
|
|
* @param {jQuery.Event} e Key down event
|
|
*/
|
|
ve.ui.Inspector.prototype.onFormKeyDown = function ( e ) {
|
|
// Escape
|
|
if ( e.which === 27 ) {
|
|
this.close();
|
|
return false;
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Handle inspector initialize events.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Inspector.prototype.onOpen = function () {
|
|
this.initialSelection = this.surface.getModel().getSelection();
|
|
};
|
|
|
|
/**
|
|
* Get matching annotations within a fragment.
|
|
*
|
|
* @method
|
|
* @param {ve.dm.SurfaceFragment} fragment Fragment to get matching annotations within
|
|
* @returns {ve.dm.AnnotationSet} Matching annotations
|
|
*/
|
|
ve.ui.Inspector.prototype.getMatchingAnnotations = function ( fragment ) {
|
|
return fragment.getAnnotations().getAnnotationsByName( this.constructor.static.typePattern );
|
|
};
|
|
|
|
/* Initialization */
|
|
|
|
ve.ui.Inspector.static.addLocalStylesheets( [ 've.ui.Inspector.css' ] );
|