mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 02:23:58 +00:00
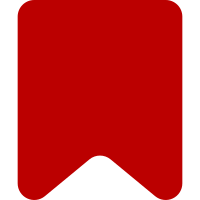
This was done in 3 rather "random" places: 1. Whenever a template is manually added. But rather late, after the template was added, in an event handler that is about focus behavior. It should not continue to manipulate the template that was just added. 2. When the dialog opens with a template preloaded by name, as it is done from the citation menu. 3. When the dialog is about to finish loading. This patch fixes 2 issues: * Get rid of a duplicate call (number 2 and 3) when using the citation menu. * Move number 1 to a place where it's executed much earlier, and only when the user clicks "add template" in a template placeholder. There is no other way to add a template to an existing transclusion, but it's still a more appropriate place I feel. Bug: T311069 Change-Id: I8a65ad703b95ba2092e9ef73493e9903e96b0dd6
89 lines
2.4 KiB
JavaScript
89 lines
2.4 KiB
JavaScript
/*!
|
|
* VisualEditor DataModel MWTransclusionPartModel class.
|
|
*
|
|
* @copyright 2011-2020 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Abstract base class for items in a {@see ve.dm.MWTransclusionModel}. Holds a back-reference to
|
|
* it's parent. Currently used for:
|
|
* - {@see ve.dm.MWTemplateModel} for a single template invocation.
|
|
* - {@see ve.dm.MWTemplatePlaceholderModel} while searching for a template name to be added.
|
|
* - {@see ve.dm.MWTransclusionContentModel} for a raw wikitext snippet.
|
|
*
|
|
* @abstract
|
|
* @class
|
|
* @mixins OO.EventEmitter
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.MWTransclusionModel} transclusion
|
|
*/
|
|
ve.dm.MWTransclusionPartModel = function VeDmMWTransclusionPartModel( transclusion ) {
|
|
// Mixin constructors
|
|
OO.EventEmitter.call( this );
|
|
|
|
// Properties
|
|
this.transclusion = transclusion;
|
|
this.id = this.transclusion.nextUniquePartId();
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.mixinClass( ve.dm.MWTransclusionPartModel, OO.EventEmitter );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* Emitted when anything changed in the content the part represents, e.g. a parameter was added to a
|
|
* template, or a value edited.
|
|
*
|
|
* @event change
|
|
*/
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Get transclusion part is in.
|
|
*
|
|
* @return {ve.dm.MWTransclusionModel} Transclusion
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.getTransclusion = function () {
|
|
return this.transclusion;
|
|
};
|
|
|
|
/**
|
|
* Get a unique part ID within the transclusion.
|
|
*
|
|
* @return {string} Unique ID
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.getId = function () {
|
|
return this.id;
|
|
};
|
|
|
|
/**
|
|
* Remove part from transclusion.
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.remove = function () {
|
|
this.transclusion.removePart( this );
|
|
};
|
|
|
|
/**
|
|
* Create a serialized representation of this part. Contains all information needed to recreate the
|
|
* original wikitext, including extra whitespace. Used in
|
|
* {@see ve.dm.MWTransclusionModel.getPlainObject}. The corresponding deserializer is in
|
|
* {@see ve.dm.MWTransclusionNode.static.getWikitext}.
|
|
*
|
|
* @return {Object|string|undefined} Serialized representation, raw wikitext, or undefined if empty
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.serialize = function () {
|
|
return undefined;
|
|
};
|
|
|
|
/**
|
|
* @return {boolean} True if there is meaningful user input that was not e.g. auto-generated
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.containsValuableData = function () {
|
|
return false;
|
|
};
|