mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
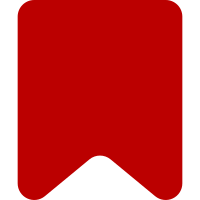
This is done by using the computed property value rather than the literal attribute value when rendering href and src attributes. Helpfully, this provides perfect URL resolution natively in the browser, which means the document's <base> is respected and all that good stuff. For GeneratedContentNodes, we also need to find all DOM elements inside the rendered DOM that have href or src attributes and resolve those. This is done in the new getRenderedDomElements() function, which the existing cleanup steps (remove <link>/<meta>/<style>, clone for correct document) were moved into. In order to make sure that the computed values are always computed correctly, we need to make sure that in cases where HTML strings in data-mw are parsed, they're parsed in the context of the correct document so the correct <base> is applied. We still need to solve this problem for models that actually store and edit an href or src as an attribute. I'll post more about that on bug 48915. Bug: 48915 Change-Id: Iaccb9e3fc05cd151a0f5e632c8d3bd3568735309
98 lines
3.6 KiB
JavaScript
98 lines
3.6 KiB
JavaScript
/*!
|
|
* VisualEditor DataModel InternalList tests.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
QUnit.module( 've.dm.InternalList' );
|
|
|
|
/* Tests */
|
|
|
|
QUnit.test( 'getDocument', 1, function ( assert ) {
|
|
var doc = ve.dm.example.createExampleDocument(),
|
|
internalList = doc.getInternalList();
|
|
assert.deepEqual( internalList.getDocument(), doc, 'Returns original document' );
|
|
} );
|
|
|
|
QUnit.test( 'queueItemHtml/getItemHtmlQueue', 5, function ( assert ) {
|
|
var doc = ve.dm.example.createExampleDocument(),
|
|
internalList = doc.getInternalList();
|
|
assert.deepEqual(
|
|
internalList.queueItemHtml( 'reference', 'foo', 'Bar' ),
|
|
{ 'index': 0, 'isNew': true },
|
|
'First queued item returns index 0 and is new'
|
|
);
|
|
assert.deepEqual(
|
|
internalList.queueItemHtml( 'reference', 'foo', 'Baz' ),
|
|
{ 'index': 0, 'isNew': false },
|
|
'Duplicate key returns index 0 and is not new'
|
|
);
|
|
assert.deepEqual(
|
|
internalList.queueItemHtml( 'reference', 'bar', 'Baz' ),
|
|
{ 'index': 1, 'isNew': true },
|
|
'Second queued item returns index 1 and is new'
|
|
);
|
|
|
|
// Queue up empty data
|
|
internalList.queueItemHtml( 'reference', 'baz', '' );
|
|
assert.deepEqual(
|
|
internalList.queueItemHtml( 'reference', 'baz', 'Quux' ),
|
|
{ 'index': 2, 'isNew': true },
|
|
'Third queued item is new because existing data in queue was empty'
|
|
);
|
|
|
|
assert.deepEqual( internalList.getItemHtmlQueue(), ['Bar', 'Baz', 'Quux'], 'getItemHtmlQueue returns stored HTML items' );
|
|
} );
|
|
|
|
QUnit.test( 'convertToData', 2, function ( assert ) {
|
|
var doc = ve.dm.example.createExampleDocument(),
|
|
internalList = doc.getInternalList(),
|
|
expectedData = [
|
|
{ 'type': 'internalList' },
|
|
{ 'type': 'internalItem' },
|
|
{ 'type': 'paragraph', 'internal': { 'generated': 'wrapper' } },
|
|
'B', 'a', 'r',
|
|
{ 'type': '/paragraph' },
|
|
{ 'type': '/internalItem' },
|
|
{ 'type': 'internalItem' },
|
|
{ 'type': 'paragraph', 'internal': { 'generated': 'wrapper' } },
|
|
'B', 'a', 'z',
|
|
{ 'type': '/paragraph' },
|
|
{ 'type': '/internalItem' },
|
|
{ 'type': '/internalList' }
|
|
];
|
|
|
|
// Mimic convert state setup (as done in ve.dm.Converter#getDataFromDom)
|
|
// TODO: The test should not (directly) reference the global instance
|
|
ve.dm.converter.doc = doc;
|
|
ve.dm.converter.store = doc.getStore();
|
|
ve.dm.converter.internalList = internalList;
|
|
ve.dm.converter.contextStack = [];
|
|
|
|
internalList.queueItemHtml( 'reference', 'foo', 'Bar' );
|
|
internalList.queueItemHtml( 'reference', 'bar', 'Baz' );
|
|
assert.deepEqual( internalList.convertToData( ve.dm.converter, doc ), expectedData, 'Data matches' );
|
|
assert.deepEqual( internalList.getItemHtmlQueue(), [], 'Items html is emptied after conversion' );
|
|
} );
|
|
|
|
QUnit.test( 'clone', 5, function ( assert ) {
|
|
var internalListClone, internalListClone2,
|
|
doc = ve.dm.example.createExampleDocument(),
|
|
doc2 = ve.dm.example.createExampleDocument(),
|
|
internalList = doc.getInternalList();
|
|
|
|
internalList.getNextUniqueNumber(); // =0
|
|
internalListClone = internalList.clone();
|
|
internalList.getNextUniqueNumber(); // =1
|
|
internalListClone2 = internalList.clone( doc2 );
|
|
internalList.getNextUniqueNumber(); // =2
|
|
|
|
assert.equal( internalListClone.getDocument(), internalList.getDocument(), 'Documents match' );
|
|
assert.equal( internalListClone2.getDocument(), doc2, 'Cloning with document parameter' );
|
|
|
|
assert.equal( internalList.getNextUniqueNumber(), 3, 'original internallist has nextUniqueNumber=3' );
|
|
assert.equal( internalListClone.getNextUniqueNumber(), 1, 'first clone has nextUniqueNumber=1' );
|
|
assert.equal( internalListClone2.getNextUniqueNumber(), 2, 'second clone has nextUniqueNumber=2' );
|
|
} );
|