mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
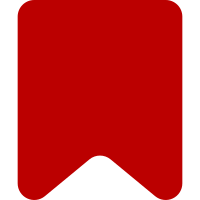
* Made method descriptions imperative: "Do this" rather than "Does this" * Changed use of "this object" to "the object" in method documentation * Added missing documentation * Fixed incorrect documentation * Fixed incorrect debug method names (as in those VeDmClassName tags we add to functions so they make sense when dumped into in the console) * Normalized use of package names throughout * Normalized class descriptions * Removed incorrect @abstract tags * Added missing @method tags * Lots of other minor cleanup Change-Id: I4ea66a2dd107613e2ea3a5f56ff54d675d72957e
140 lines
2.8 KiB
JavaScript
140 lines
2.8 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface ButtonTool class.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* UserInterface button tool.
|
|
*
|
|
* @abstract
|
|
* @class
|
|
* @extends ve.ui.Tool
|
|
* @constructor
|
|
* @param {ve.ui.Toolbar} toolbar
|
|
*/
|
|
ve.ui.ButtonTool = function VeUiButtonTool( toolbar ) {
|
|
// Parent constructor
|
|
ve.ui.Tool.call( this, toolbar );
|
|
|
|
// Properties
|
|
this.active = false;
|
|
this.disabled = false;
|
|
|
|
// Events
|
|
this.$.on( {
|
|
'mousedown': ve.bind( this.onMouseDown, this ),
|
|
'mouseup': ve.bind( this.onMouseUp, this )
|
|
} );
|
|
|
|
// Initialization
|
|
this.$.addClass( 've-ui-buttonTool ve-ui-icon-' + this.constructor.static.name );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.ButtonTool, ve.ui.Tool );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Handle the mouse button being pressed.
|
|
*
|
|
* @method
|
|
* @param {jQuery.Event} e Normalized event
|
|
*/
|
|
ve.ui.ButtonTool.prototype.onMouseDown = function ( e ) {
|
|
if ( e.which === 1 ) {
|
|
e.preventDefault();
|
|
return false;
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Handle the mouse button being released.
|
|
*
|
|
* @method
|
|
* @param {jQuery.Event} e Normalized event
|
|
*/
|
|
ve.ui.ButtonTool.prototype.onMouseUp = function ( e ) {
|
|
if ( e.which === 1 && !this.disabled ) {
|
|
return this.onClick( e );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Handle the button being clicked.
|
|
*
|
|
* This is an abstract method that must be overridden in a concrete subclass.
|
|
*
|
|
* @abstract
|
|
* @method
|
|
*/
|
|
ve.ui.ButtonTool.prototype.onClick = function () {
|
|
throw new Error(
|
|
've.ui.ButtonTool.onClick not implemented in this subclass: ' + this.constructor
|
|
);
|
|
};
|
|
|
|
/**
|
|
* Handle the toolbar state being cleared.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.ButtonTool.prototype.onClearState = function () {
|
|
this.setActive( false );
|
|
};
|
|
|
|
/**
|
|
* Check if the button is active.
|
|
*
|
|
* @method
|
|
* @param {boolean} Button is active
|
|
*/
|
|
ve.ui.ButtonTool.prototype.isActive = function () {
|
|
return this.active;
|
|
};
|
|
|
|
/**
|
|
* Make the button appear active or inactive.
|
|
*
|
|
* @method
|
|
* @param {boolean} state Make button appear active
|
|
*/
|
|
ve.ui.ButtonTool.prototype.setActive = function ( state ) {
|
|
this.active = !!state;
|
|
if ( this.active ) {
|
|
this.$.addClass( 've-ui-buttonTool-active' );
|
|
} else {
|
|
this.$.removeClass( 've-ui-buttonTool-active' );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Check if the button is disabled.
|
|
*
|
|
* @method
|
|
* @param {boolean} Button is disabled
|
|
*/
|
|
ve.ui.ButtonTool.prototype.isDisabled = function () {
|
|
return this.disabled;
|
|
};
|
|
|
|
/**
|
|
* Set the disabled state of the button.
|
|
*
|
|
* This will change the button's appearance and prevent the {onClick} from being called.
|
|
*
|
|
* @method
|
|
* @param {boolean} state Disable button
|
|
*/
|
|
ve.ui.ButtonTool.prototype.setDisabled = function ( state ) {
|
|
this.disabled = !!state;
|
|
if ( this.disabled ) {
|
|
this.$.addClass( 've-ui-buttonTool-disabled' );
|
|
} else {
|
|
this.$.removeClass( 've-ui-buttonTool-disabled' );
|
|
}
|
|
};
|