mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-24 10:48:42 +00:00
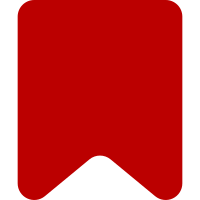
Objectives: * Allow users on-wiki to create tools and dialogs for citation templates of their choosing * Allow editing of citation templates directly, without having to go through the reference dialog * Provide citation template tools within reference editing that use the same titles and icons as the citation tools do, but don't wrap the inserted content in a ref tag Changes: * Reference list was cloning the DOM element it was inserting into its view before the generated content node could finish rendering, so it never ended up showing the finished rendering in the reference list * Documenting hack about use of reference list node's destroy method, and how we are depending on destroy not canceling generated content rendering * Introduced reference model * Added saving/updating method to transclusion model * Added getPartsList method to dm transclusion node, which caches the result and invalidates the cache on update * Added citation dialog, which extends transclusion dialog * Added cite group to toolbars, cite-template in reference dialog toolbar * Factored out getting the node to edit and saving changes procedures in transclusion dialog so they could be extended in citation dialog * Updated uses of autoAdd as per changes in oojs-ui (Ic353f91) * Renamed MWDialogTool file since there was only one tool in it * Expanded TransclusionDialogTool file out since there is now more logic to it * Switched to using ve.dm.MWReferenceModel instead of plain objects in reference search widget Configuration: If you add to MediaWiki:Visualeditor-cite-tool-definition.json the following code you will magically be presented with a delightful array of citation options: [ { "name": "web", "icon": "ref-cite-web", "template": "Cite web" }, { "name": "book", "icon": "ref-cite-book", "template": "Cite book" }, { "name": "news", "icon": "ref-cite-news", "template": "Cite news" }, { "name": "journal", "icon": "ref-cite-journal", "template": "Cite journal" } ] ...or... [ { "name": "any-name", "icon": "any-ooui-icon", "template": "Any template", "title": "Any title text" } ] The title text is derived either from the title property or from the name property by pre-pending the string 'visualeditor-cite-tool-name-' to generate a message key. Titles for 'web', 'book', 'news' and 'journal' are provided. The icon is a normal oo-ui-icon name, and more icons can be added, as usual, by adding a class called .oo-ui-icon-{icon name} to MediaWiki:Common.css. 'ref-cite-web', 'ref-cite-book', 'ref-cite-news' and 'ref-cite-journal' are provided. The template name is simply the name of the template without its namespace prefix. Depends on Ic353f91 in oojs-ui Bug: 50110 Bug: 50768 Change-Id: Id401d973b8d5fe2faec481cc777c17a24fd19dd4
190 lines
5.7 KiB
PHP
190 lines
5.7 KiB
PHP
<?php
|
|
/**
|
|
* Resource loader module providing extra data from the server to VisualEditor.
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @copyright 2011-2014 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
class VisualEditorDataModule extends ResourceLoaderModule {
|
|
|
|
/* Protected Members */
|
|
|
|
protected $origin = self::ORIGIN_USER_SITEWIDE;
|
|
protected $gitInfo;
|
|
protected $gitHeadHash;
|
|
protected $targets = array( 'desktop', 'mobile' );
|
|
|
|
/* Methods */
|
|
|
|
public function __construct () {
|
|
$this->gitInfo = new GitInfo( __DIR__ );
|
|
}
|
|
|
|
public function getScript( ResourceLoaderContext $context ) {
|
|
// Messages
|
|
$msgInfo = $this->getMessageInfo();
|
|
$parsedMessages = array();
|
|
$messages = array();
|
|
foreach ( $msgInfo['args'] as $msgKey => $msgArgs ) {
|
|
$parsedMessages[ $msgKey ] = call_user_func_array( 'wfMessage', $msgArgs )
|
|
->inLanguage( $context->getLanguage() )
|
|
->parse();
|
|
}
|
|
foreach ( $msgInfo['vals'] as $msgKey => $msgVal ) {
|
|
$messages[ $msgKey ] = $msgVal;
|
|
}
|
|
|
|
// Version information
|
|
$language = Language::factory( $context->getLanguage() );
|
|
|
|
$hash = $this->getGitHeadHash();
|
|
$id = $hash ? substr( $this->getGitHeadHash(), 0, 7 ) : false;
|
|
$url = $this->gitInfo->getHeadViewUrl();
|
|
$date = $this->gitInfo->getHeadCommitDate();
|
|
$dateString = $date ? $language->timeanddate( $date, true ) : '';
|
|
|
|
return
|
|
've.init.platform.addParsedMessages(' . FormatJson::encode(
|
|
$parsedMessages,
|
|
ResourceLoader::inDebugMode()
|
|
) . ');'.
|
|
've.init.platform.addMessages(' . FormatJson::encode(
|
|
$messages,
|
|
ResourceLoader::inDebugMode()
|
|
) . ');'.
|
|
// Documented in .docs/external.json
|
|
've.version = ' . FormatJson::encode(
|
|
array(
|
|
'id' => $id,
|
|
'url' => $url,
|
|
'timestamp' => $date,
|
|
'dateString' => $dateString,
|
|
), ResourceLoader::inDebugMode()
|
|
) . ';';
|
|
}
|
|
|
|
protected function getMessageInfo() {
|
|
$msgKeys = array();
|
|
|
|
// Messages that just require simple parsing
|
|
$msgArgs = array(
|
|
'minoredit' => array( 'minoredit' ),
|
|
'missingsummary' => array( 'missingsummary' ),
|
|
'summary' => array( 'summary' ),
|
|
'watchthis' => array( 'watchthis' ),
|
|
'visualeditor-browserwarning' => array( 'visualeditor-browserwarning' ),
|
|
'visualeditor-report-notice' => array( 'visualeditor-report-notice' ),
|
|
'visualeditor-wikitext-warning' => array( 'visualeditor-wikitext-warning' ),
|
|
);
|
|
|
|
// Override message value
|
|
$msgVals = array(
|
|
'visualeditor-feedback-link' => wfMessage( 'visualeditor-feedback-link' )
|
|
->inContentLanguage()
|
|
->text(),
|
|
);
|
|
|
|
// Copyright warning (based on EditPage::getCopyrightWarning)
|
|
global $wgRightsText;
|
|
if ( $wgRightsText ) {
|
|
$copywarnMsg = array( 'copyrightwarning',
|
|
'[[' . wfMessage( 'copyrightpage' )->inContentLanguage()->text() . ']]',
|
|
$wgRightsText );
|
|
} else {
|
|
$copywarnMsg = array( 'copyrightwarning2',
|
|
'[[' . wfMessage( 'copyrightpage' )->inContentLanguage()->text() . ']]' );
|
|
}
|
|
// EditPage supports customisation based on title, we can't support that here
|
|
// since these messages are cached on a site-level. $wgTitle is likely set to null.
|
|
$title = Title::newFromText( 'Dwimmerlaik' );
|
|
wfRunHooks( 'EditPageCopyrightWarning', array( $title, &$copywarnMsg ) );
|
|
|
|
// Keys used in copyright warning
|
|
$msgKeys[] = 'copyrightpage';
|
|
$msgKeys[] = $copywarnMsg[0];
|
|
// Normalise to 'copyrightwarning' so we have a consistent key in the front-end.
|
|
$msgArgs[ 'copyrightwarning' ] = $copywarnMsg;
|
|
|
|
// Citations
|
|
$citationDefinition = json_decode(
|
|
wfMessage( 'visualeditor-cite-tool-definition.json' )->plain()
|
|
);
|
|
$citationTools = array();
|
|
if ( is_array( $citationDefinition ) ) {
|
|
foreach ( $citationDefinition as $tool ) {
|
|
if ( !isset( $tool->title ) ) {
|
|
$tool->title =
|
|
wfMessage( 'visualeditor-cite-tool-name-' . $tool->name )->text();
|
|
$msgKeys[] = $tool->title;
|
|
}
|
|
$citationTools[] = $tool;
|
|
}
|
|
}
|
|
$msgVals['visualeditor-cite-tool-definition.json'] = json_encode( $citationTools );
|
|
|
|
$msgKeys = array_values( array_unique( array_merge(
|
|
$msgKeys,
|
|
array_keys( $msgArgs ),
|
|
array_keys( $msgVals )
|
|
) ) );
|
|
|
|
return array(
|
|
'keys' => $msgKeys,
|
|
'args' => $msgArgs,
|
|
'vals' => $msgVals,
|
|
);
|
|
}
|
|
|
|
public function getMessages() {
|
|
// We don't actually use the client-side message system for these messages.
|
|
// But we're registering them in this standardised method to make use of the
|
|
// getMsgBlobMtime utility to make cache invalidation work out-of-the-box.
|
|
|
|
$msgInfo = $this->getMessageInfo();
|
|
return $msgInfo['keys'];
|
|
}
|
|
|
|
public function getDependencies() {
|
|
return array( 'ext.visualEditor.base' );
|
|
}
|
|
|
|
public function getModifiedTime( ResourceLoaderContext $context ) {
|
|
return max(
|
|
$this->getGitHeadModifiedTime( $context ),
|
|
$this->getMsgBlobMtime( $context->getLanguage() ),
|
|
// Also invalidate this module if this file changes (i.e. when messages were
|
|
// added or removed, or when the Javascript invocation in getScript is changed).
|
|
// Use 1 because 0 = now, would invalidate continously
|
|
file_exists( __FILE__ ) ? filemtime( __FILE__ ) : 1
|
|
);
|
|
}
|
|
|
|
protected function getGitHeadModifiedTime( ResourceLoaderContext $context ) {
|
|
$cache = wfGetCache( CACHE_ANYTHING );
|
|
$key = wfMemcKey( 'resourceloader', 'vedatamodule', 'changeinfo' );
|
|
|
|
$hash = $this->getGitHeadHash();
|
|
|
|
$result = $cache->get( $key );
|
|
if ( is_array( $result ) && $result['hash'] === $hash ) {
|
|
return $result['timestamp'];
|
|
}
|
|
$timestamp = wfTimestamp();
|
|
$cache->set( $key, array(
|
|
'hash' => $hash,
|
|
'timestamp' => $timestamp,
|
|
) );
|
|
return $timestamp;
|
|
}
|
|
|
|
protected function getGitHeadHash() {
|
|
if ( $this->gitHeadHash === null ) {
|
|
$this->gitHeadHash = $this->gitInfo->getHeadSHA1();
|
|
}
|
|
return $this->gitHeadHash;
|
|
}
|
|
}
|