mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
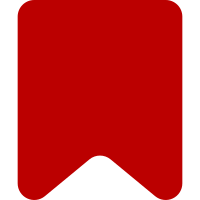
E.g. avoid calling the rather expensive method multiple times in a row, if only 1 of the results is needed. Change-Id: Iff1d2c0892367e927303f6f45d3231e04c045cab
100 lines
2.9 KiB
JavaScript
100 lines
2.9 KiB
JavaScript
/*!
|
|
* VisualEditor MWIncludesContextItem class.
|
|
*
|
|
* @copyright 2011-2020 VisualEditor Team and others; see http://ve.mit-license.org
|
|
*/
|
|
|
|
/**
|
|
* Context item for a MWIncludesContextItem.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.LinearContextItem
|
|
*
|
|
* @constructor
|
|
* @param {ve.ui.Context} context Context item is in
|
|
* @param {ve.dm.Model} model Model item is related to
|
|
* @param {Object} config Configuration options
|
|
*/
|
|
ve.ui.MWIncludesContextItem = function VeUiMWIncludesContextItem() {
|
|
// Parent constructor
|
|
ve.ui.MWIncludesContextItem.super.apply( this, arguments );
|
|
|
|
// Initialization
|
|
this.$element.addClass( 've-ui-mwIncludesContextItem' );
|
|
|
|
this.setLabel( this.getLabelMessage() );
|
|
|
|
this.$actions.remove();
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWIncludesContextItem, ve.ui.LinearContextItem );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.ui.MWIncludesContextItem.static.editable = false;
|
|
|
|
ve.ui.MWIncludesContextItem.static.name = 'mwIncludes';
|
|
|
|
ve.ui.MWIncludesContextItem.static.icon = 'markup';
|
|
|
|
ve.ui.MWIncludesContextItem.static.modelClasses = [
|
|
ve.dm.MWIncludesNode
|
|
];
|
|
|
|
/* Methods */
|
|
|
|
ve.ui.MWIncludesContextItem.prototype.getLabelMessage = function () {
|
|
var key = {
|
|
'mw:Includes/NoInclude': 'visualeditor-includes-noinclude-start',
|
|
'mw:Includes/NoInclude/End': 'visualeditor-includes-noinclude-end',
|
|
'mw:Includes/OnlyInclude': 'visualeditor-includes-onlyinclude-start',
|
|
'mw:Includes/OnlyInclude/End': 'visualeditor-includes-onlyinclude-end',
|
|
'mw:Includes/IncludeOnly': 'visualeditor-includes-includeonly'
|
|
}[ this.model.getAttribute( 'type' ) ];
|
|
// eslint-disable-next-line mediawiki/msg-doc
|
|
return key ? mw.message( key ).text() : '';
|
|
};
|
|
|
|
ve.ui.MWIncludesContextItem.prototype.getDescriptionMessage = function () {
|
|
var key = {
|
|
'mw:Includes/NoInclude': 'visualeditor-includes-noinclude-description',
|
|
'mw:Includes/OnlyInclude': 'visualeditor-includes-onlyinclude-description',
|
|
'mw:Includes/IncludeOnly': 'visualeditor-includes-includeonly-description'
|
|
}[ this.model.getAttribute( 'type' ) ];
|
|
// eslint-disable-next-line mediawiki/msg-doc
|
|
return key ? mw.message( key ).parseDom() : '';
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWIncludesContextItem.prototype.renderBody = function () {
|
|
var wikitext;
|
|
|
|
this.$body.empty();
|
|
|
|
this.$body.append( this.getDescriptionMessage() ).append( mw.msg( 'word-separator' ) );
|
|
|
|
if ( this.model.getAttribute( 'mw' ) ) {
|
|
wikitext = this.model.getAttribute( 'mw' ).src;
|
|
// The opening and closing tags are included, eww
|
|
wikitext = wikitext.replace( /^<includeonly>\s*([\s\S]*)\s*<\/includeonly>$/, '$1' );
|
|
this.$body.append( $( '<pre>' )
|
|
// The following classes are used here:
|
|
// * mw-editfont-monospace
|
|
// * mw-editfont-sans-serif
|
|
// * mw-editfont-serif
|
|
.addClass( 'mw-editfont-' + mw.user.options.get( 'editfont' ) )
|
|
.text( wikitext )
|
|
);
|
|
}
|
|
|
|
this.$body.append( mw.message( 'visualeditor-includes-documentation' ).parseDom() );
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.ui.contextItemFactory.register( ve.ui.MWIncludesContextItem );
|