mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 11:16:51 +00:00
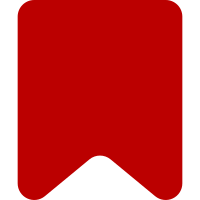
Objective: Simplify the registration and use of triggers Changes: * Renamed ve.Command to ve.Trigger * Renamed command demo to trigger demo * Removed language prefixing of triggers * Generating trigger tooltips rather than hard-coding them in i18n * Added documentation to clarify that only 'mac' and 'pc' are supported platforms, and how the default is chosen * Simplified trigger registry's register command * Updated trigger registrations Change-Id: Ibab6ad5b5c86f24707f064967dc2119a81125392
66 lines
1.8 KiB
JavaScript
66 lines
1.8 KiB
JavaScript
/*!
|
|
* VisualEditor TriggerRegistry class.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Trigger registry.
|
|
*
|
|
* @class
|
|
* @extends ve.Registry
|
|
* @constructor
|
|
*/
|
|
ve.TriggerRegistry = function VeTriggerRegistry() {
|
|
// Parent constructor
|
|
ve.Registry.call( this );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.TriggerRegistry, ve.Registry );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Register a constructor with the factory.
|
|
*
|
|
* The only supported platforms are 'mac' and 'pc'. All platforms not identified as 'mac' will be
|
|
* considered to be 'pc', including 'win', 'linux', 'solaris', etc.
|
|
*
|
|
* @method
|
|
* @param {string|string[]} name Symbolic name or list of symbolic names
|
|
* @param {ve.Trigger|Object} trigger Trigger object, or map of trigger objects keyed by
|
|
* platform name e.g. 'mac' or 'pc'
|
|
*/
|
|
ve.TriggerRegistry.prototype.register = function ( name, trigger ) {
|
|
var platform = ve.init.platform.getSystemPlatform(),
|
|
platformKey = platform === 'mac' ? 'mac' : 'pc';
|
|
|
|
// Validate arguments
|
|
if ( typeof name !== 'string' && !ve.isArray( name ) ) {
|
|
throw new Error( 'name must be a string or array, cannot be a ' + typeof name );
|
|
}
|
|
if ( !( trigger instanceof ve.Trigger ) && !ve.isPlainObject( trigger ) ) {
|
|
throw new Error(
|
|
'trigger must be an instance of ve.Trigger or an object containing instances of ' +
|
|
've.Trigger, cannot be a ' + typeof trigger
|
|
);
|
|
}
|
|
|
|
// Check for platform-specific trigger
|
|
if ( ve.isPlainObject( trigger ) ) {
|
|
// Only register if the current platform is supported
|
|
if ( platformKey in trigger ) {
|
|
ve.Registry.prototype.register.call( this, name, trigger[platformKey] );
|
|
}
|
|
} else {
|
|
ve.Registry.prototype.register.call( this, name, trigger );
|
|
}
|
|
};
|
|
|
|
/* Initialization */
|
|
|
|
ve.triggerRegistry = new ve.TriggerRegistry();
|