mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 11:16:51 +00:00
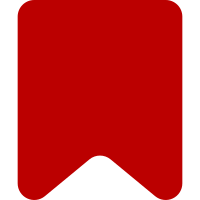
Objectives: * Make the link inspector easier to use * Try to resolve a few bugs (bug 43841, bug 43063, bug 42986) * Stop using jquery.multiSuggest (which didn't really understand annotations) * Better divide MediaWiki specifics from generic implementations Changes: VisualEditor.php, modules/ve/test/index.php, demos/ve/index.php * Updated links to files ve.Registry * Fixed mistake where registry was initialized as an array - this didn't cause any errors because you can add arbitrary properties to an array and use it like any other object ve.Factory * Removed duplicate initialization of registry property * Added entries property, which is an array that's appended to for tracking the order of registrations ve.CommandRegistry * Added mwLink command which opens the mwLink inspector ve.ui.TextInputWidget * Added basic widget class for text inputs ve.ui.TextInputMenuWidget * Added widget that provides a menu of options for a text input widget ve.ui.MWLinkTargetInputWidget * Added MediaWiki specific link target widget ve.ui.MenuWidget * Converted ve.ui.Menu into a widget * Moved the body of onSelect to onMouseUp ve.ui.LinkTargetInputWidget * Added link target widget which adds link annotation functionality to a normal text input ve.ui.InputWidget * Added generic input widget which emits reliable and instant change events and synchronizes a value property with the DOM value ve.ui.Widget * Added base widget class * Widgets can be used in any frame ve.ui.Tool * Fixed line length issues ve.ui.InspectorFactory * Made use of new entries property for factories to select the most recently added inspector if more than one match a given annotation ve.ui.Inspector * Added auto-focus on the first visible input element on open * Moved afterClose event to after re-focus on document on close * Added documentation ve.ui.Frame * Adjusted documentation * Added binding of $$ to the frame context so it can be passed around * Added documentation ve.ui.Context * Added ve.ui.Widget.css to iframes * Updated code as per moving of ve.ui.Menu to ve.ui.MenuWidget * Removed unused positionBelowOverlay method * Added CSS settings to set overlay left and width properties according to context size * Added documentation ve.ui.DropdownTool * Updated code as per moving of ve.ui.Menu to ve.ui.MenuWidget ve.ui.FormatDropdownTool * Added documentation ve.ui.MWLinkButtonTool * Added MediaWiki specific version of ve.ui.LinkButtonTool, which opens the mwLink inspector ve.ui.Widget.css * Added styles for all widgets ve.ui.Tool.css, ve.init.sa.css, ve.init.mw.ViewPageTarget.css, ve.init.mw.ViewPageTarget-apex.css * Updated code as per moving of ve.ui.Menu to ve.ui.MenuWidget ve.ui.Menu.css * Deleted (merged into ve.ui.Widget.css) ve.ui.Menu.css * Deleted suggest styles (no longer used) pending.gif, pending.psd * Added diagonal stripe animation to indicate a pending request to the API ve.ui.MWLinkInspector * Added MediaWiki specific inspector which uses MediaWiki specific annotations and widgets ve.ui.LinkInspector * Removed mw global hint (not needed anymore) * Switched from comparing targets to annotations (since the target text is ambiguous in some situations) * Switched to using input widget, which is configured using a static property * Removed use of jquery.multiSuggest * Moved MediaWiki specifics to their own class (ve.ui.MWLinkInspector) ve.init.mw.ViewPageTarget * Added MediaWiki specific toolbar and command options Change-Id: I859b5871a9d2f17d970c002067c8ff24f3513e9f
77 lines
2.2 KiB
JavaScript
77 lines
2.2 KiB
JavaScript
/*!
|
|
* VisualEditor Factory class.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Generic object factory.
|
|
*
|
|
* @abstract
|
|
* @extends ve.Registry
|
|
* @constructor
|
|
*/
|
|
ve.Factory = function VeFactory() {
|
|
// Parent constructor
|
|
ve.Registry.call( this );
|
|
|
|
// Properties
|
|
this.entries = [];
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.Factory, ve.Registry );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Register a constructor with the factory.
|
|
*
|
|
* @method
|
|
* @param {string|string[]} name Symbolic name or list of symbolic names
|
|
* @param {Function} constructor Constructor to use when creating object
|
|
* @throws {Error} Constructor must be a function
|
|
*/
|
|
ve.Factory.prototype.register = function ( name, constructor ) {
|
|
if ( typeof constructor !== 'function' ) {
|
|
throw new Error( 'constructor must be a function, cannot be a ' + typeof constructor );
|
|
}
|
|
ve.Registry.prototype.register.call( this, name, constructor );
|
|
this.entries.push( name );
|
|
};
|
|
|
|
/**
|
|
* Create an object based on a name.
|
|
*
|
|
* Name is used to look up the constructor to use, while all additional arguments are passed to the
|
|
* constructor directly, so leaving one out will pass an undefined to the constructor.
|
|
*
|
|
* @method
|
|
* @param {string} name Object name.
|
|
* @param {Mixed...} [args] Arguments to pass to the constructor.
|
|
* @returns {Object} The new object.
|
|
* @throws {Error} Unknown object name
|
|
*/
|
|
ve.Factory.prototype.create = function ( name ) {
|
|
var args, obj,
|
|
constructor = this.registry[name];
|
|
|
|
if ( constructor === undefined ) {
|
|
throw new Error( 'No class registered by that name: ' + name );
|
|
}
|
|
|
|
// Convert arguments to array and shift the first argument (name) off
|
|
args = Array.prototype.slice.call( arguments, 1 );
|
|
|
|
// We can't use the "new" operator with .apply directly because apply needs a
|
|
// context. So instead just do what "new" does: create an object that inherits from
|
|
// the constructor's prototype (which also makes it an "instanceof" the constructor),
|
|
// then invoke the constructor with the object as context, and return it (ignoring
|
|
// the constructor's return value).
|
|
obj = ve.createObject( constructor.prototype );
|
|
constructor.apply( obj, args );
|
|
return obj;
|
|
};
|