mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-29 00:30:44 +00:00
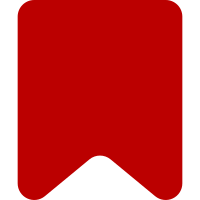
VisualEditor.php: * Make jquery.i18n a dependency of ext.visualEditor.standalone makeStaticLoader.php: * Remove ve.init.platform.addMessages() call with PHP-generated messages * Add fake module for jquery.i18n ** Needed because the module might come from MW core ** Also add special treatment for fallbacks.js and language scripts ve.init.sa.Platform.js: * Remove basic message system, replace with jquery.i18n * Add initialize method that loads messages for current language and fallbacks ve.init.sa.Target.js: * Wait for the platform to initialize before actually doing things * Add .setup() method to allow callers to short-circuit this process ** This is convenient for callers of ve.init.sa.Target in the test suite ve.ce.test.js: * Use existing ve.test.utils function for creating a surface ve.test.utils.js: * Call .setup() on the target so we can get a surface synchronously ve.init.Platform.test.js: * Make these tests async, wait for the platform to initialize * Allow for missing messages to be output either as <foo> (MW) or foo (jquery.i18n) * Get rid of message clearing code, namespace test messages instead Change-Id: Iac7dfd327eadf9b503a61510574d35d748faac92
156 lines
4.1 KiB
JavaScript
156 lines
4.1 KiB
JavaScript
/*!
|
|
* VisualEditor Initialization Platform class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Generic Initialization platform.
|
|
*
|
|
* @abstract
|
|
* @mixins OO.EventEmitter
|
|
*
|
|
* @constructor
|
|
*/
|
|
ve.init.Platform = function VeInitPlatform() {
|
|
// Mixin constructors
|
|
OO.EventEmitter.call( this );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.mixinClass( ve.init.Platform, OO.EventEmitter );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Get a regular expression that matches allowed external link URLs.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @returns {RegExp} Regular expression object
|
|
*/
|
|
ve.init.Platform.prototype.getExternalLinkUrlProtocolsRegExp = function () {
|
|
throw new Error( 've.init.Platform.getExternalLinkUrlProtocolsRegExp must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Get a remotely accessible URL to the modules directory.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @returns {string} Remote modules URL
|
|
*/
|
|
ve.init.Platform.prototype.getModulesUrl = function () {
|
|
throw new Error( 've.init.Platform.getModulesUrl must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Add multiple messages to the localization system.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @param {Object} messages Containing plain message values
|
|
*/
|
|
ve.init.Platform.prototype.addMessages = function () {
|
|
throw new Error( 've.init.Platform.addMessages must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Get a message from the localization system.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @param {string} key Message key
|
|
* @param {Mixed...} [args] List of arguments which will be injected at $1, $2, etc. in the messaage
|
|
* @returns {string} Localized message, or key or '<' + key + '>' if message not found
|
|
*/
|
|
ve.init.Platform.prototype.getMessage = function () {
|
|
throw new Error( 've.init.Platform.getMessage must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Add multiple parsed messages to the localization system.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @param {Object} messages Map of message-key/html pairs
|
|
*/
|
|
ve.init.Platform.prototype.addParsedMessages = function () {
|
|
throw new Error( 've.init.Platform.addParsedMessages must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Get a parsed message as HTML string.
|
|
*
|
|
* Does not support $# replacements.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @param {string} key Message key
|
|
* @returns {string} Parsed localized message as HTML string
|
|
*/
|
|
ve.init.Platform.prototype.getParsedMessage = function () {
|
|
throw new Error( 've.init.Platform.getParsedMessage must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Get client platform string from browser.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @returns {string} Client platform string
|
|
*/
|
|
ve.init.Platform.prototype.getSystemPlatform = function () {
|
|
throw new Error( 've.init.Platform.getSystemPlatform must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Get the user language and any fallback languages.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @returns {string[]} User language strings
|
|
*/
|
|
ve.init.Platform.prototype.getUserLanguages = function () {
|
|
throw new Error( 've.init.Platform.getUserLanguages must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Get a list of URL entry points where media can be found.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @returns {string[]} API URLs
|
|
*/
|
|
ve.init.Platform.prototype.getMediaSources = function () {
|
|
throw new Error( 've.init.Platform.getMediaSources must be overridden in subclass' );
|
|
};
|
|
|
|
/**
|
|
* Initialize the platform. The default implementation is to do nothing and return a resolved
|
|
* promise. Subclasses should override this if they have asynchronous initialization work to do.
|
|
*
|
|
* External callers should not call this. Instead, call #getInitializedPromise.
|
|
*
|
|
* @private
|
|
* @returns {jQuery.Promise} Promise that will be resolved once initialization is done
|
|
*/
|
|
ve.init.Platform.prototype.initialize = function () {
|
|
return $.Deferred().resolve().promise();
|
|
};
|
|
|
|
/**
|
|
* Get a promise to track when the platform has initialized. The platform won't be ready for use
|
|
* until this promise is resolved.
|
|
*
|
|
* @returns {jQuery.Promise} Promise that will be resolved once the platform is ready
|
|
*/
|
|
ve.init.Platform.prototype.getInitializedPromise = function () {
|
|
if ( !this.initialized ) {
|
|
this.initialized = this.initialize();
|
|
}
|
|
return this.initialized;
|
|
};
|