mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-23 22:13:34 +00:00
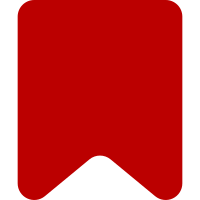
I've verified that no other extension uses this code apart from VE and locally tested that with this patch, VE still works and nothing explodes. There parent patch takes care of making sure the mode is no longer injected and we now have just etags with no hacked in modes because we now use direct mode always. Bug: T341612 Change-Id: Ib1756bf60104467a3a34be9bbb06d8f63537e550
84 lines
1.9 KiB
PHP
84 lines
1.9 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\VisualEditor;
|
|
|
|
use MediaWiki\Config\ServiceOptions;
|
|
use MediaWiki\Permissions\Authority;
|
|
use MediaWiki\Rest\Handler\Helper\PageRestHelperFactory;
|
|
use RequestContext;
|
|
|
|
/**
|
|
* @since 1.40
|
|
*/
|
|
class VisualEditorParsoidClientFactory {
|
|
|
|
/**
|
|
* @internal For use by ServiceWiring.php only or when locating the service
|
|
* @var string
|
|
*/
|
|
public const SERVICE_NAME = 'VisualEditor.ParsoidClientFactory';
|
|
|
|
/** @var bool */
|
|
public const ENABLE_COOKIE_FORWARDING = 'EnableCookieForwarding';
|
|
|
|
/**
|
|
* @internal For used by ServiceWiring.php
|
|
*
|
|
* @var array
|
|
*/
|
|
public const CONSTRUCTOR_OPTIONS = [
|
|
self::ENABLE_COOKIE_FORWARDING,
|
|
self::DEFAULT_PARSOID_CLIENT_SETTING,
|
|
];
|
|
|
|
/** @var string */
|
|
public const DEFAULT_PARSOID_CLIENT_SETTING = 'VisualEditorDefaultParsoidClient';
|
|
|
|
private ServiceOptions $options;
|
|
private PageRestHelperFactory $pageRestHelperFactory;
|
|
|
|
public function __construct(
|
|
ServiceOptions $options,
|
|
PageRestHelperFactory $pageRestHelperFactory
|
|
) {
|
|
$this->options = $options;
|
|
$this->options->assertRequiredOptions( self::CONSTRUCTOR_OPTIONS );
|
|
|
|
$this->pageRestHelperFactory = $pageRestHelperFactory;
|
|
}
|
|
|
|
/**
|
|
* Create a ParsoidClient for accessing Parsoid.
|
|
*
|
|
* @param string|string[]|false $cookiesToForward
|
|
* @param Authority|null $performer
|
|
*
|
|
* @return ParsoidClient
|
|
*/
|
|
public function createParsoidClient(
|
|
/* Kept for compatibility with other extensions */ $cookiesToForward,
|
|
?Authority $performer = null
|
|
): ParsoidClient {
|
|
if ( $performer === null ) {
|
|
$performer = RequestContext::getMain()->getAuthority();
|
|
}
|
|
|
|
return $this->createDirectClient( $performer );
|
|
}
|
|
|
|
/**
|
|
* Create a ParsoidClient for accessing Parsoid.
|
|
*
|
|
* @param Authority $performer
|
|
*
|
|
* @return ParsoidClient
|
|
*/
|
|
private function createDirectClient( Authority $performer ): ParsoidClient {
|
|
return new DirectParsoidClient(
|
|
$this->pageRestHelperFactory,
|
|
$performer
|
|
);
|
|
}
|
|
|
|
}
|