mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
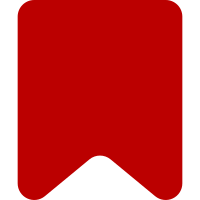
Let's keep the ugly regexp and the comments about why we do this in a single place. This is mostly without behavior changes, with three exceptions: * ve.dm.MWImageModel#attachScalable now passes a title with spaces instead of underscores to the Scalable (this doesn't matter because it's normalized to use spaces later anyway). * ve.dm.MWImageNode#getFilename now returns a title with spaces instead of underscores. This is used in some API queries and when rendering thumbnails for missing files, and this format is actually more correct for both of these. * ve.dm.MWTemplateModel now URI-decodes the template title. This actually fixes a bug where trying to edit a template transclusion whose title contains a '?' would throw an exception about invalid title. Also, clarify that the return value of ve.dm.MWImageModel#getFilename and ve.dm.MWImageNode#getFilename is different :( Change-Id: I8e09015cea82308017ed925ec755b7231518126e
84 lines
2.5 KiB
JavaScript
84 lines
2.5 KiB
JavaScript
/*!
|
|
* VisualEditor DataModel MWCategoryMetaItem class.
|
|
*
|
|
* @copyright 2011-2018 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* DataModel category meta item.
|
|
*
|
|
* @class
|
|
* @extends ve.dm.MetaItem
|
|
* @constructor
|
|
* @param {Object} element Reference to element in meta-linmod
|
|
*/
|
|
ve.dm.MWCategoryMetaItem = function VeDmMWCategoryMetaItem() {
|
|
// Parent constructor
|
|
ve.dm.MWCategoryMetaItem.super.apply( this, arguments );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.dm.MWCategoryMetaItem, ve.dm.MetaItem );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.dm.MWCategoryMetaItem.static.name = 'mwCategory';
|
|
|
|
ve.dm.MWCategoryMetaItem.static.group = 'mwCategory';
|
|
|
|
ve.dm.MWCategoryMetaItem.static.matchTagNames = [ 'link' ];
|
|
|
|
ve.dm.MWCategoryMetaItem.static.matchRdfaTypes = [ 'mw:PageProp/Category' ];
|
|
|
|
ve.dm.MWCategoryMetaItem.static.toDataElement = function ( domElements ) {
|
|
var href = domElements[ 0 ].getAttribute( 'href' ),
|
|
data = ve.parseParsoidResourceName( href ),
|
|
rawTitleAndFragment = data.rawTitle.match( /^(.*?)(?:#(.*))?$/ ),
|
|
titleAndFragment = data.title.match( /^(.*?)(?:#(.*))?$/ );
|
|
return {
|
|
type: this.name,
|
|
attributes: {
|
|
hrefPrefix: data.hrefPrefix,
|
|
category: titleAndFragment[ 1 ],
|
|
origCategory: rawTitleAndFragment[ 1 ],
|
|
sortkey: titleAndFragment[ 2 ] || '',
|
|
origSortkey: rawTitleAndFragment[ 2 ] || ''
|
|
}
|
|
};
|
|
};
|
|
|
|
ve.dm.MWCategoryMetaItem.static.toDomElements = function ( dataElement, doc ) {
|
|
var href,
|
|
domElement = doc.createElement( 'link' ),
|
|
hrefPrefix = dataElement.attributes.hrefPrefix || '',
|
|
category = dataElement.attributes.category || '',
|
|
sortkey = dataElement.attributes.sortkey || '',
|
|
origCategory = dataElement.attributes.origCategory || '',
|
|
origSortkey = dataElement.attributes.origSortkey || '',
|
|
normalizedOrigCategory = ve.decodeURIComponentIntoArticleTitle( origCategory ),
|
|
normalizedOrigSortkey = ve.decodeURIComponentIntoArticleTitle( origSortkey );
|
|
if ( normalizedOrigSortkey === sortkey ) {
|
|
sortkey = origSortkey;
|
|
} else {
|
|
sortkey = encodeURIComponent( sortkey );
|
|
}
|
|
if ( normalizedOrigCategory === category ) {
|
|
category = origCategory;
|
|
} else {
|
|
category = encodeURIComponent( category );
|
|
}
|
|
domElement.setAttribute( 'rel', 'mw:PageProp/Category' );
|
|
href = hrefPrefix + category;
|
|
if ( sortkey !== '' ) {
|
|
href += '#' + sortkey;
|
|
}
|
|
domElement.setAttribute( 'href', href );
|
|
return [ domElement ];
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.dm.modelRegistry.register( ve.dm.MWCategoryMetaItem );
|