mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-10-01 06:06:47 +00:00
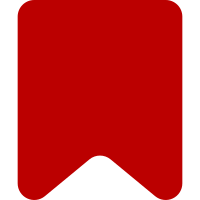
Objectives: * Reduce the number of clicks and mouse maneuvers required to insert media, references or template parameters * Make use of highlighting with mouse movement or arrow key presses, similar to menus, to suggest action when clicked * Improve the way media search results look and feel Changes: ve.ui.SelectWidget.js * Add mouseleave handler to un-highlight when the mouse exits the widget * Document highlight events (already being emitted) ve.ui.SearchWidget.js * Propagate both select and highlight events from results widget * Make arrow keys change highlight instead of selection * Get rid of enter event, make enter key select highlighted item instead * Provide direct access to results widget through getResults method ve.ui.MenuWidget.js * Use the selected item as a starting point if nothing is currently highlighted when adjusting the highlight position ve.ui.Dialog.js * Add footless option to hide the foot element and make the body extend all the way down to the bottom * Remove applyButton, which only some dialogs need, and should be creating themselves, along with other buttons as needed ve.ui.Widget.css * Change highlight and selected colors of option widgets to match other selection colors used elsewhere * Leave selected and highlighted widget looking selected ve.ui.Frame.css * Add background color to combat any color that might have been applied to the frame body in the imported CSS from the parent frame ve.ui.Dialog.css * Add rules for footless mode ve.ui.MWReferenceResultWidget.js, ve.ui.MWParameterResultWidget.js, ve.ui.MWMediaResultWidget.js * Allow highlighting ve.ui.MWParamterSearchWidget.js * Switch from selecting the first item when filtering to highlighting ve-mw/ve.ui.Widget.js * Adjust media result widget styling to better match other elements ve.ui.MWTransclusionDialog.js, ve.ui.MWReferenceListDialog.js, ve.ui.MWReferenceEditDialog.js, ve.ui.MWMetaDialog.js ve.ui.MWMediaEditDialog.js * Add apply button, as per it being removed from parent class ve.ui.MWTransclusionDialog.js, ve.ui.MWReferenceInsertDialog.js, ve.ui.MWMediaInsertDialog.js * Insert parameter/reference/media on select, instead of clicking an insert button * Use 'insert' instead of 'apply' as argument for close method Bug: 50774 Bug: 51143 Change-Id: Ia18e79f1f8df2540f465468edb01f5ce989bf843
102 lines
2.7 KiB
JavaScript
Executable file
102 lines
2.7 KiB
JavaScript
Executable file
/*!
|
|
* VisualEditor UserInterface MWMediaResultWidget class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/*global mw */
|
|
|
|
/**
|
|
* Creates an ve.ui.MWMediaResultWidget object.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.OptionWidget
|
|
*
|
|
* @constructor
|
|
* @param {Mixed} data Item data
|
|
* @param {Object} [config] Config options
|
|
* @cfg {number} [size] Media thumbnail size
|
|
*/
|
|
ve.ui.MWMediaResultWidget = function VeUiMWMediaResultWidget( data, config ) {
|
|
// Configuration intialization
|
|
config = config || {};
|
|
|
|
// Parent constructor
|
|
ve.ui.OptionWidget.call( this, data, config );
|
|
|
|
// Properties
|
|
this.size = config.size || 150;
|
|
this.$thumb = this.buildThumbnail();
|
|
this.$overlay = this.$$( '<div>' );
|
|
|
|
// Initialization
|
|
this.setLabel( new mw.Title( this.data.title ).getNameText() );
|
|
this.$overlay.addClass( 've-ui-mwMediaResultWidget-overlay' );
|
|
this.$
|
|
.addClass( 've-ui-mwMediaResultWidget ve-ui-texture-pending' )
|
|
.css( { 'width': this.size, 'height': this.size } )
|
|
.prepend( this.$thumb, this.$overlay );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.MWMediaResultWidget, ve.ui.OptionWidget );
|
|
|
|
/* Methods */
|
|
|
|
ve.ui.MWMediaResultWidget.prototype.onThumbnailLoad = function () {
|
|
this.$thumb.first().addClass( 've-ui-texture-transparency' );
|
|
this.$
|
|
.addClass( 've-ui-mwMediaResultWidget-done' )
|
|
.removeClass( 've-ui-texture-pending' );
|
|
};
|
|
|
|
ve.ui.MWMediaResultWidget.prototype.onThumbnailError = function () {
|
|
this.$thumb.last()
|
|
.css( 'background-image', '' )
|
|
.addClass( 've-ui-texture-alert' );
|
|
this.$
|
|
.addClass( 've-ui-mwMediaResultWidget-error' )
|
|
.removeClass( 've-ui-texture-pending' );
|
|
};
|
|
|
|
/**
|
|
* Build a thumbnail.
|
|
*
|
|
* @method
|
|
* @returns {jQuery} Thumbnail element
|
|
*/
|
|
ve.ui.MWMediaResultWidget.prototype.buildThumbnail = function () {
|
|
var info = this.data.imageinfo[0],
|
|
image = new Image(),
|
|
$image = this.$$( image ),
|
|
$back = this.$$( '<div>' ),
|
|
$front = this.$$( '<div>' ),
|
|
$thumb = $back.add( $front );
|
|
|
|
// Preload image
|
|
$image
|
|
.load( ve.bind( this.onThumbnailLoad, this ) )
|
|
.error( ve.bind( this.onThumbnailError, this ) );
|
|
image.src = info.thumburl;
|
|
|
|
$thumb.addClass( 've-ui-mwMediaResultWidget-thumbnail' );
|
|
$thumb.last().css( 'background-image', 'url(' + info.thumburl + ')' );
|
|
if ( info.width >= this.size && info.height >= this.size ) {
|
|
$front.addClass( 've-ui-mwMediaResultWidget-crop' );
|
|
$thumb.css( { 'width': '100%', 'height': '100%' } );
|
|
} else {
|
|
$thumb.css( {
|
|
'width': info.thumbwidth,
|
|
'height': info.thumbheight,
|
|
'left': '50%',
|
|
'top': '50%',
|
|
'margin-left': Math.round( -info.thumbwidth / 2 ),
|
|
'margin-top': Math.round( -info.thumbheight / 2 )
|
|
} );
|
|
}
|
|
|
|
return $thumb;
|
|
};
|