mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-30 21:56:49 +00:00
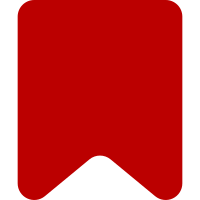
Objectives: * Make it possible to add items to toolbars without having to have all toolbars know about the items in advance * Make it possible to specialize an existing tool and have it be used instead of the base implementation Approach: * Tools are named using a path-style category/id/ext system, making them selectable, the latter component being used to differentiate extended tools from their base classes, but is ignored during selection * Toolbars have ToolGroups, which include or exclude tools by category or category/id, and order them by promoting and demoting selections of tools by category or category/id Future: * Add a way to place available but not yet placed tools in an "overflow" group * Add a mode to ToolGroup to make the tools a multi-column drop-down style list with labels so tools with less obvious icons are easier to identify - and probably use this as the overflow group Change-Id: I7625f861435a99ce3d7a2b1ece9731aaab1776f8
141 lines
2.9 KiB
JavaScript
141 lines
2.9 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface Tool class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* UserInterface tool.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
* @extends ve.ui.Widget
|
|
*
|
|
* @constructor
|
|
* @param {ve.ui.Toolbar} toolbar
|
|
* @param {Object} [config] Config options
|
|
*/
|
|
ve.ui.Tool = function VeUiTool( toolbar, config ) {
|
|
// Parent constructor
|
|
ve.ui.Widget.call( this, config );
|
|
|
|
// Properties
|
|
this.toolbar = toolbar;
|
|
|
|
// Events
|
|
this.toolbar.connect( this, { 'updateState': 'onUpdateState' } );
|
|
ve.ui.triggerRegistry.connect( this, { 'register': 'onTriggerRegistryRegister' } );
|
|
|
|
// Initialization
|
|
this.setTitle();
|
|
this.$.addClass( 've-ui-tool' );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.Tool, ve.ui.Widget );
|
|
|
|
/* Static Properties */
|
|
|
|
/**
|
|
* Symbolic name of tool.
|
|
*
|
|
* @abstract
|
|
* @static
|
|
* @property {string}
|
|
*/
|
|
ve.ui.Tool.static.name = '';
|
|
|
|
/**
|
|
* Message key for tool title.
|
|
*
|
|
* @abstract
|
|
* @static
|
|
* @property {string}
|
|
*/
|
|
ve.ui.Tool.static.titleMessage = null;
|
|
|
|
/**
|
|
* Tool should be automatically added to toolbars.
|
|
*
|
|
* @static
|
|
* @property {boolean}
|
|
*/
|
|
ve.ui.Tool.static.autoAdd = true;
|
|
|
|
/**
|
|
* Check if this tool can be used on a model.
|
|
*
|
|
* @method
|
|
* @static
|
|
* @inheritable
|
|
* @param {ve.dm.Model} model Model to check
|
|
* @returns {boolean} Tool can be used to edit model
|
|
*/
|
|
ve.ui.Tool.static.canEditModel = function () {
|
|
return false;
|
|
};
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Handle trigger registry register events.
|
|
*
|
|
* If a trigger is registered after the tool is loaded, this handler will ensure the tool's title is
|
|
* updated to reflect the available key command for the tool.
|
|
*
|
|
* @param {string} name Symbolic name of trigger
|
|
*/
|
|
ve.ui.Tool.prototype.onTriggerRegistryRegister = function ( name ) {
|
|
if ( name === this.constructor.static.name ) {
|
|
this.setTitle();
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Handle the toolbar state being updated.
|
|
*
|
|
* This is an abstract method that must be overridden in a concrete subclass.
|
|
*
|
|
* @abstract
|
|
* @method
|
|
*/
|
|
ve.ui.Tool.prototype.onUpdateState = function () {
|
|
throw new Error(
|
|
've.ui.Tool.onUpdateState not implemented in this subclass:' + this.constructor
|
|
);
|
|
};
|
|
|
|
/**
|
|
* Sets the tool title attribute in the dom.
|
|
*
|
|
* Combines trigger i18n with tooltip message if trigger exists.
|
|
* Otherwise defaults to titleMessage value.
|
|
*
|
|
* @method
|
|
* @chainable
|
|
*/
|
|
ve.ui.Tool.prototype.setTitle = function () {
|
|
var trigger = ve.ui.triggerRegistry.lookup( this.constructor.static.name ),
|
|
labelMessage = this.constructor.static.titleMessage,
|
|
labelText = labelMessage ? ve.msg( labelMessage ) : '';
|
|
|
|
if ( trigger ) {
|
|
labelText += ' [' + trigger.getMessage() + ']';
|
|
}
|
|
this.$.attr( 'title', labelText );
|
|
return this;
|
|
};
|
|
|
|
/**
|
|
* Destroy tool.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Tool.prototype.destroy = function () {
|
|
this.toolbar.disconnect( this );
|
|
ve.ui.triggerRegistry.disconnect( this );
|
|
this.$.remove();
|
|
};
|