mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-05 22:22:54 +00:00
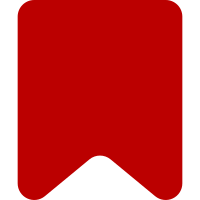
Whenever there is more than 2 spaces (except the extra space on a continued line of an @ tag, or the extra space on a continued line of a list item) it causes a <pre> context. Removed both spurious spaces that caused a <pre> and ones that didn't but looked like it could. When making an ordered or unordered list, the first item needs to be on a new line and in block context (e.g. an empty line before it). Otherwise it is rendered inline as 1. Foo 2. Bar (such as in #rebuildNodes where both the ordered and unordered lists were broken). Change-Id: Id0f154854afbdc8e5a8387da92e6b2cdf0875f69
86 lines
1.8 KiB
JavaScript
86 lines
1.8 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface FlaggableElement class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Flaggable element.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
*
|
|
* @constructor
|
|
* @param {Object} [config] Config options
|
|
* @cfg {string[]} [flags=[]] Styling flags, e.g. 'primary', 'destructive' or 'constructive'
|
|
*/
|
|
ve.ui.FlaggableElement = function VeUiFlaggableElement( config ) {
|
|
// Config initialization
|
|
config = config || {};
|
|
|
|
// Properties
|
|
this.flags = {};
|
|
|
|
// Initialization
|
|
this.setFlags( config.flags );
|
|
};
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Check if a flag is set.
|
|
*
|
|
* @method
|
|
* @param {string} flag Flag name to check
|
|
* @returns {boolean} Has flag
|
|
*/
|
|
ve.ui.FlaggableElement.prototype.hasFlag = function ( flag ) {
|
|
return flag in this.flags;
|
|
};
|
|
|
|
/**
|
|
* Get the names of all flags.
|
|
*
|
|
* @method
|
|
* @returns {string[]} flags Flag names
|
|
*/
|
|
ve.ui.FlaggableElement.prototype.getFlags = function () {
|
|
return ve.getObjectKeys( this.flags );
|
|
};
|
|
|
|
/**
|
|
* Add one or more flags.
|
|
*
|
|
* @method
|
|
* @param {string[]|Object.<string, boolean>} flags List of flags to add, or list of set/remove
|
|
* values, keyed by flag name
|
|
* @chainable
|
|
*/
|
|
ve.ui.FlaggableElement.prototype.setFlags = function ( flags ) {
|
|
var i, len, flag,
|
|
classPrefix = 've-ui-flaggableElement-';
|
|
|
|
if ( ve.isArray( flags ) ) {
|
|
for ( i = 0, len = flags.length; i < len; i++ ) {
|
|
flag = flags[i];
|
|
// Set
|
|
this.flags[flag] = true;
|
|
this.$.addClass( classPrefix + flag );
|
|
}
|
|
} else if ( ve.isPlainObject( flags ) ) {
|
|
for ( flag in flags ) {
|
|
if ( flags[flags] ) {
|
|
// Set
|
|
this.flags[flag] = true;
|
|
this.$.addClass( classPrefix + flag );
|
|
} else {
|
|
// Remove
|
|
delete this.flags[flag];
|
|
this.$.removeClass( classPrefix + flag );
|
|
}
|
|
}
|
|
}
|
|
return this;
|
|
};
|