mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-29 16:44:51 +00:00
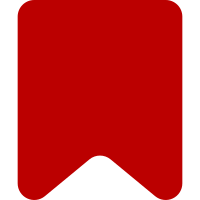
New changes: f039957f3 [BREAKING CHANGE] Use keyed objects for importRules blacklists Local changes: Use extendObject to set importRules This allows us to inherit the ruleset from the parent so we don't have to worry about keeping it up to date. (For example alienTableCell from upstream was missing in MW). Media/Gallery dialogs: Add missing mwTable types. Change-Id: I366a091ff4def66cc25200b3d1b2c23ba6b716f7 Depends-On: I8ff7e8242c8db235a0f9e11e2e52f90d62d368a0
150 lines
4 KiB
JavaScript
150 lines
4 KiB
JavaScript
/*!
|
|
* VisualEditor MediaWiki Initialization CollabTarget class.
|
|
*
|
|
* @copyright 2011-2016 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* MediaWiki mobile article target.
|
|
*
|
|
* @class
|
|
* @extends ve.init.mw.Target
|
|
*
|
|
* @constructor
|
|
* @param {mw.Title} title Page sub-title
|
|
* @param {rebaserUrl} string Rebaser server URL
|
|
* @param {Object} [config] Configuration options
|
|
* @cfg {mw.Title} [importTitle] Title to import
|
|
*/
|
|
ve.init.mw.CollabTarget = function VeInitMwCollabTarget( title, rebaserUrl, config ) {
|
|
config = config || {};
|
|
config.toolbarConfig = $.extend( {
|
|
shadow: true,
|
|
actions: true,
|
|
floatable: true
|
|
}, config.toolbarConfig );
|
|
|
|
this.title = title;
|
|
this.rebaserUrl = rebaserUrl;
|
|
this.importTitle = config.importTitle || null;
|
|
|
|
// Parent constructor
|
|
ve.init.mw.CollabTarget.super.call( this, config );
|
|
|
|
// HACK: Disable history commands until supported (T185706)
|
|
ve.ui.commandRegistry.unregister( 'undo' );
|
|
ve.ui.commandRegistry.unregister( 'redo' );
|
|
|
|
// HACK: Disable references until supported (T194838)
|
|
ve.ui.commandRegistry.unregister( 'reference' );
|
|
ve.ui.commandRegistry.unregister( 'referencesList' );
|
|
ve.ui.commandRegistry.unregister( 'citefromid' );
|
|
|
|
// eslint-disable-next-line no-jquery/no-global-selector
|
|
this.$editableContent = $( '#mw-content-text' );
|
|
|
|
this.toolbarExportButton = new OO.ui.ButtonWidget( {
|
|
label: ve.msg( 'visualeditor-rebase-client-export' ),
|
|
flags: [ 'progressive', 'primary' ]
|
|
} ).connect( this, { click: 'onExportButtonClick' } );
|
|
|
|
// Initialization
|
|
this.$element.addClass( 've-init-mw-articleTarget ve-init-mw-collabTarget' );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.init.mw.CollabTarget, ve.init.mw.Target );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.init.mw.CollabTarget.static.name = 'collab';
|
|
|
|
ve.init.mw.CollabTarget.static.trackingName = 'collab';
|
|
|
|
ve.init.mw.CollabTarget.static.toolbarGroups = ve.copy( ve.init.mw.CollabTarget.static.toolbarGroups );
|
|
ve.init.mw.CollabTarget.static.toolbarGroups.splice( 4, 0, {
|
|
name: 'commentAnnotation',
|
|
include: [ 'commentAnnotation' ]
|
|
} );
|
|
// HACK: Disable references until supported (T194838)
|
|
ve.init.mw.CollabTarget.static.toolbarGroups = ve.init.mw.CollabTarget.static.toolbarGroups.filter( function ( group ) {
|
|
return group.name !== 'reference';
|
|
} );
|
|
|
|
ve.init.mw.CollabTarget.static.importRules = ve.copy( ve.init.mw.CollabTarget.static.importRules );
|
|
ve.init.mw.CollabTarget.static.importRules.external.blacklist[ 'link/mwExternal' ] = false;
|
|
|
|
ve.init.mw.CollabTarget.static.actionGroups = [
|
|
{
|
|
name: 'help',
|
|
include: [ 'help' ]
|
|
},
|
|
{
|
|
name: 'pageMenu',
|
|
type: 'list',
|
|
icon: 'menu',
|
|
indicator: null,
|
|
title: ve.msg( 'visualeditor-pagemenu-tooltip' ),
|
|
include: [ 'changeDirectionality', 'findAndReplace' ]
|
|
},
|
|
{
|
|
name: 'authorList',
|
|
include: [ 'authorList' ]
|
|
}
|
|
];
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Page modifications after editor load.
|
|
*/
|
|
ve.init.mw.CollabTarget.prototype.transformPage = function () {
|
|
};
|
|
|
|
/**
|
|
* Page modifications after editor teardown.
|
|
*/
|
|
ve.init.mw.CollabTarget.prototype.restorePage = function () {
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.init.mw.CollabTarget.prototype.setupToolbar = function () {
|
|
// Parent method
|
|
ve.init.mw.CollabTarget.super.prototype.setupToolbar.apply( this, arguments );
|
|
|
|
this.getToolbar().$actions.append( this.toolbarExportButton.$element );
|
|
};
|
|
|
|
/**
|
|
* Handle click events from the export button
|
|
*/
|
|
ve.init.mw.CollabTarget.prototype.onExportButtonClick = function () {
|
|
var surface = this.getSurface(),
|
|
windowAction = ve.ui.actionFactory.create( 'window', surface );
|
|
windowAction.open( 'mwExportWikitext', { surface: surface } );
|
|
};
|
|
|
|
/**
|
|
* Get the title of the imported document, if there was one
|
|
*
|
|
* @return {mw.Title|null} Title of imported document
|
|
*/
|
|
ve.init.mw.CollabTarget.prototype.getImportTitle = function () {
|
|
return this.importTitle;
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.init.mw.CollabTarget.prototype.getPageName = function () {
|
|
return this.getImportTitle() || this.pageName;
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.init.mw.targetFactory.register( ve.init.mw.CollabTarget );
|