mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-27 20:26:46 +00:00
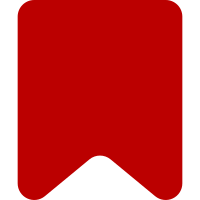
* Added documentation for ve.AnnotationSet * Replaced uses of "// Inheritance" with "// parent Constructor" * Added "// Mixin constructor" where needed * Added missing section comments like "/* Static Methods */" * Cleaned up excessive newlines (matching /\n\n\n/g) * Put unnecessarily multi-line statements on a single line Change-Id: I2c9b47ba296f7dd3c9cc2985581fbcefd6d76325
57 lines
1.4 KiB
JavaScript
57 lines
1.4 KiB
JavaScript
/**
|
|
* VisualEditor AnnotationSet class.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Ordered set of annotations.
|
|
*
|
|
* @constructor
|
|
* @extends {ve.OrderedHashSet}
|
|
* @param {Object[]} annotations Array of annotation objects
|
|
*/
|
|
ve.AnnotationSet = function ( annotations ) {
|
|
// Parent constructor
|
|
ve.OrderedHashSet.call( this, ve.getHash, annotations );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.AnnotationSet, ve.OrderedHashSet );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Gets a clone.
|
|
*
|
|
* @method
|
|
* @returns {ve.AnnotationSet} Copy of annotation set
|
|
*/
|
|
ve.AnnotationSet.prototype.clone = function () {
|
|
return new ve.AnnotationSet( this );
|
|
};
|
|
|
|
/**
|
|
* Gets an annotation set containing only annotations within this set of a given type.
|
|
*
|
|
* @method
|
|
* @param {String|RegExp} type Regular expression or string to compare types with
|
|
* @returns {ve.AnnotationSet} Copy of annotation set
|
|
*/
|
|
ve.AnnotationSet.prototype.getAnnotationsOfType = function ( type ) {
|
|
return this.filter( 'type', type );
|
|
};
|
|
|
|
/**
|
|
* Checks if any annotations in this set are of a given type.
|
|
*
|
|
* @method
|
|
* @param {String|RegExp} type Regular expression or string to compare types with
|
|
* @returns {Boolean} Annotation of given type exists in this set
|
|
*/
|
|
ve.AnnotationSet.prototype.hasAnnotationOfType = function ( type ) {
|
|
return this.containsMatching( 'type', type );
|
|
};
|