mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-11 16:48:43 +00:00
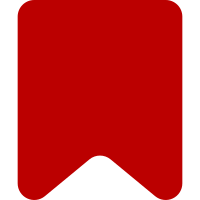
Changes to the use statements done automatically via script Addition of missing use statement done manually Change-Id: Ia08d43b3973a12b8e0628c8552bee1c4b702f249
83 lines
1.7 KiB
PHP
83 lines
1.7 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\VisualEditor;
|
|
|
|
/**
|
|
* VisualEditorHookRunner
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @copyright 2011-2021 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license MIT
|
|
*/
|
|
|
|
use MediaWiki\HookContainer\HookContainer;
|
|
use MediaWiki\Output\OutputPage;
|
|
use MediaWiki\Page\ProperPageIdentity;
|
|
use MediaWiki\User\UserIdentity;
|
|
use Skin;
|
|
|
|
class VisualEditorHookRunner implements
|
|
VisualEditorApiVisualEditorEditPreSaveHook,
|
|
VisualEditorApiVisualEditorEditPostSaveHook,
|
|
VisualEditorBeforeEditorHook
|
|
{
|
|
|
|
private HookContainer $hookContainer;
|
|
|
|
public function __construct( HookContainer $hookContainer ) {
|
|
$this->hookContainer = $hookContainer;
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function onVisualEditorApiVisualEditorEditPreSave(
|
|
ProperPageIdentity $page,
|
|
UserIdentity $user,
|
|
string $wikitext,
|
|
array &$params,
|
|
array $pluginData,
|
|
array &$apiResponse
|
|
) {
|
|
return $this->hookContainer->run( 'VisualEditorApiVisualEditorEditPreSave', [
|
|
$page,
|
|
$user,
|
|
$wikitext,
|
|
&$params,
|
|
$pluginData,
|
|
&$apiResponse
|
|
], [ 'abortable' => true ] );
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function onVisualEditorApiVisualEditorEditPostSave(
|
|
ProperPageIdentity $page,
|
|
UserIdentity $user,
|
|
string $wikitext,
|
|
array $params,
|
|
array $pluginData,
|
|
array $saveResult,
|
|
array &$apiResponse
|
|
): void {
|
|
$this->hookContainer->run( 'VisualEditorApiVisualEditorEditPostSave', [
|
|
$page,
|
|
$user,
|
|
$wikitext,
|
|
$params,
|
|
$pluginData,
|
|
$saveResult,
|
|
&$apiResponse
|
|
], [ 'abortable' => false ] );
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function onVisualEditorBeforeEditor(
|
|
OutputPage $output,
|
|
Skin $skin
|
|
): bool {
|
|
return $this->hookContainer->run( 'VisualEditorBeforeEditor', [
|
|
$output,
|
|
$skin
|
|
], [ 'abortable' => true ] );
|
|
}
|
|
}
|