mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/TitleBlacklist
synced 2024-11-15 02:03:58 +00:00
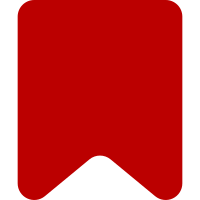
$req can legitimately be null, so we need to add null to the assert as well, so the code doesn't stop immediately. This is a follow-up for I83f9374b2f3236097860f4aecc694326b891905b Change-Id: I85dbc1b4c1452d0671879e6cc02c5411090b1d1d
59 lines
1.9 KiB
PHP
59 lines
1.9 KiB
PHP
<?php
|
|
|
|
use MediaWiki\Auth\AbstractPreAuthenticationProvider;
|
|
use MediaWiki\Auth\AuthenticationRequest;
|
|
use MediaWiki\Auth\AuthManager;
|
|
|
|
class TitleBlacklistPreAuthenticationProvider extends AbstractPreAuthenticationProvider {
|
|
protected $blockAutoAccountCreation;
|
|
|
|
public function __construct( $params = [] ) {
|
|
global $wgTitleBlacklistBlockAutoAccountCreation;
|
|
|
|
$params += [
|
|
'blockAutoAccountCreation' => $wgTitleBlacklistBlockAutoAccountCreation
|
|
];
|
|
|
|
$this->blockAutoAccountCreation = (bool)$params['blockAutoAccountCreation'];
|
|
}
|
|
|
|
public function getAuthenticationRequests( $action, array $options ) {
|
|
$needOverrideOption = false;
|
|
switch ( $action ) {
|
|
case AuthManager::ACTION_CREATE:
|
|
$user = User::newFromName( $options['username'] ) ?: new User();
|
|
$needOverrideOption = TitleBlacklist::userCanOverride( $user, 'new-account' );
|
|
break;
|
|
}
|
|
|
|
return $needOverrideOption ? [ new TitleBlacklistAuthenticationRequest() ] : [];
|
|
}
|
|
|
|
public function testForAccountCreation( $user, $creator, array $reqs ) {
|
|
/** @var TitleBlacklistAuthenticationRequest $req */
|
|
$req = AuthenticationRequest::getRequestByClass( $reqs,
|
|
TitleBlacklistAuthenticationRequest::class );
|
|
// For phan check, to ensure that $req is instance of \TitleBlacklistAuthenticationRequest
|
|
// or null
|
|
if ( $req instanceof TitleBlacklistAuthenticationRequest ) {
|
|
$override = $req->ignoreTitleBlacklist;
|
|
} else {
|
|
$override = false;
|
|
}
|
|
|
|
return TitleBlacklistHooks::testUserName( $user->getName(), $creator, $override, true );
|
|
}
|
|
|
|
public function testUserForCreation( $user, $autocreate, array $options = [] ) {
|
|
$sv = StatusValue::newGood();
|
|
$creator = RequestContext::getMain()->getUser();
|
|
|
|
if ( !$autocreate && empty( $options['creating'] ) || $this->blockAutoAccountCreation ) {
|
|
$sv->merge( TitleBlacklistHooks::testUserName(
|
|
$user->getName(), $creator, true, (bool)$autocreate
|
|
) );
|
|
}
|
|
return $sv;
|
|
}
|
|
}
|