mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/TitleBlacklist
synced 2024-09-23 10:18:34 +00:00
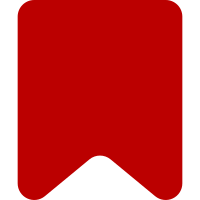
Changes to the use statements done automatically via script Addition of missing use statement done manually Change-Id: I3a7b448f3793f6690bb329a2e5255a558048ed5a
66 lines
2 KiB
PHP
66 lines
2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\TitleBlacklist;
|
|
|
|
use MediaWiki\Auth\AbstractPreAuthenticationProvider;
|
|
use MediaWiki\Auth\AuthenticationRequest;
|
|
use MediaWiki\Auth\AuthManager;
|
|
use MediaWiki\Context\RequestContext;
|
|
use MediaWiki\User\User;
|
|
use StatusValue;
|
|
|
|
class TitleBlacklistPreAuthenticationProvider extends AbstractPreAuthenticationProvider {
|
|
|
|
/** @var bool */
|
|
protected $blockAutoAccountCreation;
|
|
|
|
public function __construct( $params = [] ) {
|
|
global $wgTitleBlacklistBlockAutoAccountCreation;
|
|
|
|
$params += [
|
|
'blockAutoAccountCreation' => $wgTitleBlacklistBlockAutoAccountCreation
|
|
];
|
|
|
|
$this->blockAutoAccountCreation = (bool)$params['blockAutoAccountCreation'];
|
|
}
|
|
|
|
public function getAuthenticationRequests( $action, array $options ) {
|
|
$needOverrideOption = false;
|
|
switch ( $action ) {
|
|
case AuthManager::ACTION_CREATE:
|
|
$user = User::newFromName( $options['username'] ) ?: new User();
|
|
$needOverrideOption = TitleBlacklist::userCanOverride( $user, 'new-account' );
|
|
break;
|
|
}
|
|
|
|
return $needOverrideOption ? [ new TitleBlacklistAuthenticationRequest() ] : [];
|
|
}
|
|
|
|
public function testForAccountCreation( $user, $creator, array $reqs ) {
|
|
/** @var TitleBlacklistAuthenticationRequest $req */
|
|
$req = AuthenticationRequest::getRequestByClass( $reqs,
|
|
TitleBlacklistAuthenticationRequest::class );
|
|
// For phan check, to ensure that $req is instance of \TitleBlacklistAuthenticationRequest
|
|
// or null
|
|
if ( $req instanceof TitleBlacklistAuthenticationRequest ) {
|
|
$override = $req->ignoreTitleBlacklist;
|
|
} else {
|
|
$override = false;
|
|
}
|
|
|
|
return Hooks::testUserName( $user->getName(), $creator, $override, true );
|
|
}
|
|
|
|
public function testUserForCreation( $user, $autocreate, array $options = [] ) {
|
|
$sv = StatusValue::newGood();
|
|
$creator = RequestContext::getMain()->getUser();
|
|
|
|
if ( ( !$autocreate && empty( $options['creating'] ) ) || $this->blockAutoAccountCreation ) {
|
|
$sv->merge( Hooks::testUserName(
|
|
$user->getName(), $creator, true, (bool)$autocreate
|
|
) );
|
|
}
|
|
return $sv;
|
|
}
|
|
}
|