mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Thanks
synced 2024-11-15 10:59:42 +00:00
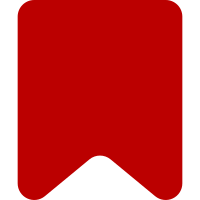
This adds support for thanking for log entries. It gets the log ID from and attribute on the thank link (which does not yet have this; that will come in T187485), and sets the source to be 'log' if it's being called from Special:Log (this can be extended in the future if we want to thank for log entries from elsewhere). Bug: T186921 Change-Id: Ifdb458b873ed4f1e6e64760658d2293aba1e02b3
124 lines
3.4 KiB
JavaScript
124 lines
3.4 KiB
JavaScript
( function ( $, mw, OO ) {
|
|
'use strict';
|
|
|
|
function reloadThankedState() {
|
|
$( 'a.mw-thanks-thank-link' ).each( function ( idx, el ) {
|
|
var $thankLink = $( el );
|
|
if ( mw.thanks.thanked.contains( $thankLink ) ) {
|
|
$thankLink.before( mw.msg( 'thanks-thanked' ) );
|
|
$thankLink.remove();
|
|
}
|
|
} );
|
|
}
|
|
|
|
// $thankLink is the element with the data-revision-id attribute
|
|
// $thankElement is the element to be removed on success
|
|
function sendThanks( $thankLink, $thankElement ) {
|
|
var source, apiParams;
|
|
|
|
if ( $thankLink.data( 'clickDisabled' ) ) {
|
|
// Prevent double clicks while we haven't received a response from API request
|
|
return false;
|
|
}
|
|
$thankLink.data( 'clickDisabled', true );
|
|
|
|
// Determine the thank source (history, diff, or log).
|
|
if ( mw.config.get( 'wgAction' ) === 'history' ) {
|
|
source = 'history';
|
|
} else if ( mw.config.get( 'wgCanonicalSpecialPageName' ) === 'Log' ) {
|
|
source = 'log';
|
|
} else {
|
|
source = 'diff';
|
|
}
|
|
|
|
// Construct the API parameters.
|
|
apiParams = {
|
|
action: 'thank',
|
|
source: source
|
|
};
|
|
if ( $thankLink.data( 'log-id' ) ) {
|
|
apiParams.log = $thankLink.data( 'log-id' );
|
|
} else {
|
|
apiParams.rev = $thankLink.data( 'revision-id' );
|
|
}
|
|
|
|
// Send the API request.
|
|
( new mw.Api() ).postWithToken( 'csrf', apiParams )
|
|
.then(
|
|
// Success
|
|
function () {
|
|
$thankElement.before( mw.message( 'thanks-thanked', mw.user, $thankLink.data( 'recipient-gender' ) ).escaped() );
|
|
$thankElement.remove();
|
|
mw.thanks.thanked.push( $thankLink );
|
|
},
|
|
// Fail
|
|
function ( errorCode ) {
|
|
// If error occured, enable attempting to thank again
|
|
$thankLink.data( 'clickDisabled', false );
|
|
switch ( errorCode ) {
|
|
case 'invalidrevision':
|
|
OO.ui.alert( mw.msg( 'thanks-error-invalidrevision' ) );
|
|
break;
|
|
case 'ratelimited':
|
|
OO.ui.alert( mw.msg( 'thanks-error-ratelimited', mw.user ) );
|
|
break;
|
|
case 'revdeleted':
|
|
OO.ui.alert( mw.msg( 'thanks-error-revdeleted' ) );
|
|
break;
|
|
default:
|
|
OO.ui.alert( mw.msg( 'thanks-error-undefined', errorCode ) );
|
|
}
|
|
}
|
|
);
|
|
}
|
|
|
|
/**
|
|
* Add interactive handlers to all 'thank' links in $content
|
|
*
|
|
* @param {jQuery} $content
|
|
*/
|
|
function addActionToLinks( $content ) {
|
|
var $thankLinks = $content.find( 'a.mw-thanks-thank-link' );
|
|
if ( mw.config.get( 'thanks-confirmation-required' ) ) {
|
|
$thankLinks.each( function () {
|
|
var $thankLink = $( this );
|
|
$thankLink.confirmable( {
|
|
i18n: {
|
|
confirm: mw.msg( 'thanks-confirmation2', mw.user ),
|
|
no: mw.msg( 'cancel' ),
|
|
noTitle: mw.msg( 'thanks-thank-tooltip-no', mw.user ),
|
|
yes: mw.msg( 'thanks-button-thank', mw.user, $thankLink.data( 'recipient-gender' ) ),
|
|
yesTitle: mw.msg( 'thanks-thank-tooltip-yes', mw.user )
|
|
},
|
|
handler: function ( e ) {
|
|
e.preventDefault();
|
|
sendThanks( $thankLink, $thankLink.closest( '.jquery-confirmable-wrapper' ) );
|
|
}
|
|
} );
|
|
} );
|
|
} else {
|
|
$thankLinks.click( function ( e ) {
|
|
var $thankLink = $( this );
|
|
e.preventDefault();
|
|
sendThanks( $thankLink, $thankLink );
|
|
} );
|
|
}
|
|
}
|
|
|
|
if ( $.isReady ) {
|
|
// This condition is required for soft-reloads
|
|
// to also trigger the reloadThankedState
|
|
reloadThankedState();
|
|
} else {
|
|
$( reloadThankedState );
|
|
}
|
|
|
|
$( function () {
|
|
addActionToLinks( $( 'body' ) );
|
|
} );
|
|
|
|
mw.hook( 'wikipage.diff' ).add( function ( $content ) {
|
|
addActionToLinks( $content );
|
|
} );
|
|
}( jQuery, mediaWiki, OO ) );
|