mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/TextExtracts
synced 2024-11-15 11:59:38 +00:00
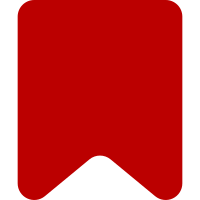
This patch does two things: * Add a testMemCacheHelpers() test for the setCache(), getFromCache(), as well as cacheKey() methods. * Simplify the existing test setup utilizing the PHPUnit4And6Compat layer. I'm also replacing the Config mock with an actual HashConfig, because that's a really trivial object that does not need to be mocked. Change-Id: I7e8a62651a53919d338a7d921f548e941b671670
156 lines
4.3 KiB
PHP
156 lines
4.3 KiB
PHP
<?php
|
|
|
|
namespace TextExtracts\Test;
|
|
|
|
use MediaWikiCoversValidator;
|
|
use TextExtracts\ApiQueryExtracts;
|
|
use Wikimedia\TestingAccessWrapper;
|
|
|
|
/**
|
|
* @covers \TextExtracts\ApiQueryExtracts
|
|
* @group TextExtracts
|
|
*/
|
|
class ApiQueryExtractsTest extends \MediaWikiTestCase {
|
|
use MediaWikiCoversValidator;
|
|
use \PHPUnit4And6Compat;
|
|
|
|
private function newInstance() {
|
|
$config = new \HashConfig( [
|
|
'ParserCacheExpireTime' => \IExpiringStore::TTL_INDEFINITE,
|
|
] );
|
|
|
|
$context = $this->createMock( \IContextSource::class );
|
|
$context->method( 'getConfig' )
|
|
->willReturn( $config );
|
|
|
|
$main = $this->createMock( \ApiMain::class );
|
|
$main->expects( $this->once() )
|
|
->method( 'getContext' )
|
|
->willReturn( $context );
|
|
|
|
$query = $this->createMock( \ApiQuery::class );
|
|
$query->expects( $this->once() )
|
|
->method( 'getMain' )
|
|
->willReturn( $main );
|
|
|
|
return new ApiQueryExtracts( $query, '', $config );
|
|
}
|
|
|
|
public function testMemCacheHelpers() {
|
|
$this->setMwGlobals( 'wgMemc', new \HashBagOStuff() );
|
|
|
|
$title = $this->createMock( \Title::class );
|
|
$title->method( 'getPageLanguage' )
|
|
->willReturn( $this->createMock( \Language::class ) );
|
|
|
|
$page = $this->createMock( \WikiPage::class );
|
|
$page->method( 'getTitle' )
|
|
->willReturn( $title );
|
|
|
|
$text = 'Text to cache';
|
|
|
|
/** @var ApiQueryExtracts $instance */
|
|
$instance = TestingAccessWrapper::newFromObject( $this->newInstance() );
|
|
$this->assertFalse( $instance->getFromCache( $page, false ), 'is not cached yet' );
|
|
$instance->setCache( $page, $text );
|
|
$this->assertSame( $text, $instance->getFromCache( $page, false ) );
|
|
}
|
|
|
|
public function testSelfDocumentation() {
|
|
/** @var ApiQueryExtracts $instance */
|
|
$instance = TestingAccessWrapper::newFromObject( $this->newInstance() );
|
|
|
|
$this->assertInternalType( 'string', $instance->getCacheMode( [] ) );
|
|
$this->assertNotEmpty( $instance->getExamplesMessages() );
|
|
$this->assertInternalType( 'string', $instance->getHelpUrls() );
|
|
|
|
$params = $instance->getAllowedParams();
|
|
$this->assertInternalType( 'array', $params );
|
|
$this->assertArrayHasKey( 'chars', $params );
|
|
$this->assertEquals( $params['chars'][\ApiBase::PARAM_MIN], 1 );
|
|
$this->assertEquals( $params['chars'][\ApiBase::PARAM_MAX], 1200 );
|
|
$this->assertArrayHasKey( 'limit', $params );
|
|
$this->assertEquals( $params['limit'][\ApiBase::PARAM_DFLT], 20 );
|
|
$this->assertEquals( $params['limit'][\ApiBase::PARAM_TYPE], 'limit' );
|
|
$this->assertEquals( $params['limit'][\ApiBase::PARAM_MIN], 1 );
|
|
$this->assertEquals( $params['limit'][\ApiBase::PARAM_MAX], 20 );
|
|
$this->assertEquals( $params['limit'][\ApiBase::PARAM_MAX2], 20 );
|
|
}
|
|
|
|
/**
|
|
* @dataProvider provideFirstSectionsToExtract
|
|
*/
|
|
public function testGetFirstSection( $text, $isPlainText, $expected ) {
|
|
/** @var ApiQueryExtracts $instance */
|
|
$instance = TestingAccessWrapper::newFromObject( $this->newInstance() );
|
|
|
|
$this->assertSame( $expected, $instance->getFirstSection( $text, $isPlainText ) );
|
|
}
|
|
|
|
public function provideFirstSectionsToExtract() {
|
|
return [
|
|
'Plain text match' => [
|
|
"First\nsection \1\2... \1\2...",
|
|
true,
|
|
"First\nsection ",
|
|
],
|
|
'Plain text without a match' => [
|
|
'Example\1\2...',
|
|
true,
|
|
'Example\1\2...',
|
|
],
|
|
|
|
'HTML match' => [
|
|
"First\nsection <h1>...<h2>...",
|
|
false,
|
|
"First\nsection ",
|
|
],
|
|
'HTML without a match' => [
|
|
'Example <h11>...',
|
|
false,
|
|
'Example <h11>...',
|
|
],
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @dataProvider provideSectionsToFormat
|
|
*/
|
|
public function testDoSections( $text, $format, $expected ) {
|
|
/** @var ApiQueryExtracts $instance */
|
|
$instance = TestingAccessWrapper::newFromObject( $this->newInstance() );
|
|
$instance->params = [ 'sectionformat' => $format ];
|
|
|
|
$this->assertSame( $expected, $instance->doSections( $text ) );
|
|
}
|
|
|
|
public function provideSectionsToFormat() {
|
|
$level = 3;
|
|
$marker = "\1\2$level\2\1";
|
|
|
|
return [
|
|
'Raw' => [
|
|
"$marker Headline\t\nNext line",
|
|
'raw',
|
|
"$marker Headline\t\nNext line",
|
|
],
|
|
'Wiki text' => [
|
|
"$marker Headline\t\nNext line",
|
|
'wiki',
|
|
"\n=== Headline ===\nNext line",
|
|
],
|
|
'Plain text' => [
|
|
"$marker Headline\t\nNext line",
|
|
'plain',
|
|
"\nHeadline\nNext line",
|
|
],
|
|
|
|
'Multiple matches' => [
|
|
"${marker}First\n${marker}Second",
|
|
'wiki',
|
|
"\n=== First ===\n\n=== Second ===",
|
|
],
|
|
];
|
|
}
|
|
}
|