mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/TemplateData
synced 2024-11-25 16:25:41 +00:00
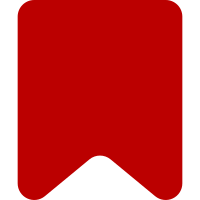
See Iae0e2ce3. Since TemplateData master requires core master, this just depends on the master patch instead of trying to maintain BC. Depends-On: Iae0e2ce3bd42dd4776a9779664086119ac188412 Change-Id: Iebe8557affd5ecf206814e7fdef5d8656417dadb
143 lines
3.5 KiB
PHP
143 lines
3.5 KiB
PHP
<?php
|
|
/**
|
|
* Implement the 'templatedata' query module in the API.
|
|
* Format JSON only.
|
|
*
|
|
* @file
|
|
*/
|
|
|
|
/**
|
|
* @ingroup API
|
|
* @emits error.code templatedata-corrupt
|
|
* @todo Support continuation (see I1a6e51cd)
|
|
*/
|
|
class ApiTemplateData extends ApiBase {
|
|
/**
|
|
* For backwards compatibility, this module needs to output format=json when
|
|
* no format is specified.
|
|
* @return ApiFormatBase|null
|
|
*/
|
|
public function getCustomPrinter() {
|
|
if ( $this->getMain()->getVal( 'format' ) === null ) {
|
|
$this->addDeprecation(
|
|
'apiwarn-templatedata-deprecation-format', 'action=templatedata&!format'
|
|
);
|
|
return $this->getMain()->createPrinterByName( 'json' );
|
|
}
|
|
return null;
|
|
}
|
|
|
|
/**
|
|
* @return ApiPageSet
|
|
*/
|
|
private function getPageSet() {
|
|
if ( !isset( $this->mPageSet ) ) {
|
|
$this->mPageSet = new ApiPageSet( $this );
|
|
}
|
|
return $this->mPageSet;
|
|
}
|
|
|
|
public function execute() {
|
|
$params = $this->extractRequestParams();
|
|
$result = $this->getResult();
|
|
|
|
if ( is_null( $params['lang'] ) ) {
|
|
$langCode = false;
|
|
} elseif ( !Language::isValidCode( $params['lang'] ) ) {
|
|
$this->dieWithError( [ 'apierror-invalidlang', 'lang' ] );
|
|
} else {
|
|
$langCode = $params['lang'];
|
|
}
|
|
|
|
$pageSet = $this->getPageSet();
|
|
$pageSet->execute();
|
|
$titles = $pageSet->getGoodTitles(); // page_id => Title object
|
|
|
|
if ( !count( $titles ) ) {
|
|
$result->addValue( null, 'pages', (object) [] );
|
|
return;
|
|
}
|
|
|
|
$db = $this->getDB();
|
|
$res = $db->select( 'page_props',
|
|
[ 'pp_page', 'pp_value' ], [
|
|
'pp_page' => array_keys( $titles ),
|
|
'pp_propname' => 'templatedata'
|
|
],
|
|
__METHOD__,
|
|
[ 'ORDER BY', 'pp_page' ]
|
|
);
|
|
|
|
$resp = [];
|
|
|
|
foreach ( $res as $row ) {
|
|
$rawData = $row->pp_value;
|
|
$tdb = TemplateDataBlob::newFromDatabase( $rawData );
|
|
$status = $tdb->getStatus();
|
|
|
|
if ( !$status->isOK() ) {
|
|
$this->dieWithError(
|
|
[ 'apierror-templatedata-corrupt', intval( $row->pp_page ), $status->getMessage() ]
|
|
);
|
|
}
|
|
|
|
if ( $langCode ) {
|
|
$data = $tdb->getDataInLanguage( $langCode );
|
|
} else {
|
|
$data = $tdb->getData();
|
|
}
|
|
|
|
// HACK: don't let ApiResult's formatversion=1 compatibility layer mangle our booleans
|
|
// to empty strings / absent properties
|
|
foreach ( $data->params as &$param ) {
|
|
$param->{ApiResult::META_BC_BOOLS} = [ 'required', 'suggested', 'deprecated' ];
|
|
}
|
|
unset( $param );
|
|
|
|
$data->params->{ApiResult::META_TYPE} = 'kvp';
|
|
$data->params->{ApiResult::META_KVP_KEY_NAME} = 'key';
|
|
$data->params->{ApiResult::META_INDEXED_TAG_NAME} = 'param';
|
|
ApiResult::setIndexedTagName( $data->paramOrder, 'p' );
|
|
|
|
$resp[$row->pp_page] = [
|
|
'title' => strval( $titles[$row->pp_page] ),
|
|
] + (array) $data;
|
|
}
|
|
|
|
ApiResult::setArrayType( $resp, 'kvp', 'id' );
|
|
ApiResult::setIndexedTagName( $resp, 'page' );
|
|
|
|
// Set top level element
|
|
$result->addValue( null, 'pages', (object) $resp );
|
|
|
|
$values = $pageSet->getNormalizedTitlesAsResult();
|
|
if ( $values ) {
|
|
$result->addValue( null, 'normalized', $values );
|
|
}
|
|
$redirects = $pageSet->getRedirectTitlesAsResult();
|
|
if ( $redirects ) {
|
|
$result->addValue( null, 'redirects', $redirects );
|
|
}
|
|
}
|
|
|
|
public function getAllowedParams( $flags = 0 ) {
|
|
return $this->getPageSet()->getFinalParams( $flags ) + [
|
|
'lang' => null
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getExamplesMessages()
|
|
*/
|
|
protected function getExamplesMessages() {
|
|
return [
|
|
'action=templatedata&titles=Template:Stub|Template:Example'
|
|
=> 'apihelp-templatedata-example-1',
|
|
];
|
|
}
|
|
|
|
public function getHelpUrls() {
|
|
return 'https://www.mediawiki.org/wiki/Extension:TemplateData';
|
|
}
|
|
}
|