mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/TemplateData
synced 2024-11-24 16:03:45 +00:00
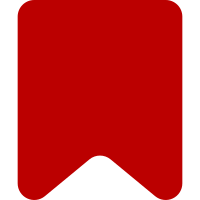
MediaWiki core change I04b1a384 added support for i18n of API module help. This takes advantage of that while still maintaining backwards compatibility with earlier versions of MediaWiki. Once support for MediaWiki before 1.25 is dropped, the methods marked deprecated in this patch may be removed. Change-Id: I67395aff48185f3e09da31b51a08aa2541fe6a17
162 lines
3.8 KiB
PHP
162 lines
3.8 KiB
PHP
<?php
|
|
/**
|
|
* Implement the 'templatedata' query module in the API.
|
|
* Format JSON only.
|
|
*
|
|
* @file
|
|
*/
|
|
|
|
/**
|
|
* @ingroup API
|
|
* @emits error.code templatedata-corrupt
|
|
* @todo Support continuation (see I1a6e51cd)
|
|
*/
|
|
class ApiTemplateData extends ApiBase {
|
|
|
|
/**
|
|
* Override built-in handling of format parameter.
|
|
* Only JSON is supported.
|
|
*
|
|
* @return ApiFormatBase
|
|
*/
|
|
public function getCustomPrinter() {
|
|
$params = $this->extractRequestParams();
|
|
$format = $params['format'];
|
|
$allowed = array( 'json', 'jsonfm' );
|
|
if ( in_array( $format, $allowed ) ) {
|
|
return $this->getMain()->createPrinterByName( $format );
|
|
}
|
|
return $this->getMain()->createPrinterByName( $allowed[0] );
|
|
}
|
|
|
|
/**
|
|
* @return ApiPageSet
|
|
*/
|
|
private function getPageSet() {
|
|
if ( !isset( $this->mPageSet ) ) {
|
|
$this->mPageSet = new ApiPageSet( $this );
|
|
}
|
|
return $this->mPageSet;
|
|
}
|
|
|
|
public function execute() {
|
|
$params = $this->extractRequestParams();
|
|
$result = $this->getResult();
|
|
|
|
if ( is_null( $params['lang'] ) ) {
|
|
$langCode = false;
|
|
} elseif ( !Language::isValidCode( $params['lang'] ) ) {
|
|
$this->dieUsage( 'Invalid language code for parameter lang', 'invalidlang' );
|
|
} else {
|
|
$langCode = $params['lang'];
|
|
}
|
|
|
|
$pageSet = $this->getPageSet();
|
|
$pageSet->execute();
|
|
$titles = $pageSet->getGoodTitles(); // page_id => Title object
|
|
|
|
if ( !count( $titles ) ) {
|
|
$result->addValue( null, 'pages', (object) array() );
|
|
return;
|
|
}
|
|
|
|
$db = $this->getDB();
|
|
$res = $db->select( 'page_props',
|
|
array( 'pp_page', 'pp_value' ), array(
|
|
'pp_page' => array_keys( $titles ),
|
|
'pp_propname' => 'templatedata'
|
|
),
|
|
__METHOD__,
|
|
array( 'ORDER BY', 'pp_page' )
|
|
);
|
|
|
|
$resp = array();
|
|
|
|
foreach ( $res as $row ) {
|
|
$rawData = $row->pp_value;
|
|
$tdb = TemplateDataBlob::newFromDatabase( $rawData );
|
|
$status = $tdb->getStatus();
|
|
|
|
if ( !$status->isOK() ) {
|
|
$this->dieUsage(
|
|
'Page #' . intval( $row->pp_page ) . ' templatedata contains invalid data: '
|
|
. $status->getMessage(), 'templatedata-corrupt'
|
|
);
|
|
}
|
|
|
|
if ( $langCode ) {
|
|
$data = $tdb->getDataInLanguage( $langCode );
|
|
} else {
|
|
$data = $tdb->getData();
|
|
}
|
|
|
|
$resp[$row->pp_page] = array(
|
|
'title' => strval( $titles[$row->pp_page] ),
|
|
) + (array) $data;
|
|
}
|
|
|
|
// Set top level element
|
|
$result->addValue( null, 'pages', (object) $resp );
|
|
|
|
$values = $pageSet->getNormalizedTitlesAsResult();
|
|
if ( $values ) {
|
|
$result->addValue( null, 'normalized', $values );
|
|
}
|
|
$redirects = $pageSet->getRedirectTitlesAsResult();
|
|
if ( $redirects ) {
|
|
$result->addValue( null, 'redirects', $redirects );
|
|
}
|
|
}
|
|
|
|
public function getAllowedParams( $flags = 0 ) {
|
|
return $this->getPageSet()->getFinalParams( $flags ) + array(
|
|
'format' => array(
|
|
ApiBase::PARAM_DFLT => 'json',
|
|
ApiBase::PARAM_TYPE => array( 'json', 'jsonfm' ),
|
|
),
|
|
'lang' => null
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @deprecated since MediaWiki core 1.25
|
|
*/
|
|
public function getParamDescription() {
|
|
return $this->getPageSet()->getParamDescription() + array(
|
|
'format' => 'The format of the output',
|
|
'lang' => 'Return localized values in this language (by default all available' .
|
|
' translations are returned)',
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @deprecated since MediaWiki core 1.25
|
|
*/
|
|
public function getDescription() {
|
|
return 'Data stored by the TemplateData extension';
|
|
}
|
|
|
|
/**
|
|
* @deprecated since MediaWiki core 1.25
|
|
*/
|
|
public function getExamples() {
|
|
return array(
|
|
'api.php?action=templatedata&titles=Template:Stub|Template:Example',
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getExamplesMessages()
|
|
*/
|
|
protected function getExamplesMessages() {
|
|
return array(
|
|
'action=templatedata&titles=Template:Stub|Template:Example'
|
|
=> 'apihelp-templatedata-example-1',
|
|
);
|
|
}
|
|
|
|
public function getHelpUrls() {
|
|
return 'https://www.mediawiki.org/wiki/Extension:TemplateData';
|
|
}
|
|
}
|