mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/TemplateData
synced 2024-11-11 16:59:25 +00:00
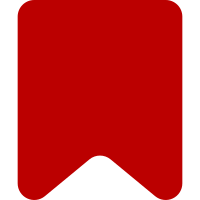
Changes to the use statements done automatically via script Addition of missing use statements done manually Change-Id: I8e049e495a0d0f3a0e1ee02a8252354b12604bf9
54 lines
1.2 KiB
PHP
54 lines
1.2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\TemplateData;
|
|
|
|
use MediaWiki\Status\Status;
|
|
|
|
/**
|
|
* @license GPL-2.0-or-later
|
|
*/
|
|
class TemplateDataStatus {
|
|
|
|
/**
|
|
* @param Status $status
|
|
* @return array contains StatusValue ok and errors fields (does not serialize value)
|
|
*/
|
|
public static function jsonSerialize( Status $status ): array {
|
|
if ( $status->isOK() ) {
|
|
return [ 'ok' => true ];
|
|
}
|
|
|
|
[ $errorsOnlyStatus, $warningsOnlyStatus ] = $status->splitByErrorType();
|
|
// note that non-scalar values are not supported in errors or warnings
|
|
return [
|
|
'ok' => false,
|
|
'errors' => $errorsOnlyStatus->getErrors(),
|
|
'warnings' => $warningsOnlyStatus->getErrors()
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @param Status|array|null $json contains StatusValue ok and errors fields (does not serialize value)
|
|
* @return Status|null
|
|
*/
|
|
public static function newFromJson( $json ): ?Status {
|
|
if ( !is_array( $json ) ) {
|
|
return $json;
|
|
}
|
|
|
|
if ( $json['ok'] ) {
|
|
return Status::newGood();
|
|
}
|
|
|
|
$status = new Status();
|
|
foreach ( $json['errors'] as $error ) {
|
|
$status->fatal( $error['message'], ...$error['params'] );
|
|
}
|
|
foreach ( $json['warnings'] as $warning ) {
|
|
$status->warning( $warning['message'], ...$warning['params'] );
|
|
}
|
|
return $status;
|
|
}
|
|
|
|
}
|