mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Scribunto
synced 2024-11-26 09:15:23 +00:00
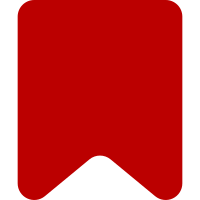
* Implemented the new parser interface based on a frame object, as described in the design document and wikitech-l. * Added parser tests for the new interface. * Removed {{script:}} parser function * Allow named parameters to {{#invoke:}} * Don't trim the return value * If a function invoked by #invoke returns multiple values, concatenate them into a single string. * If there is an error during parse, show the error message as an HTML comment as well as via JavaScript. This makes parser test construction easier, and probably makes debugging easier also. * Rename mw_internal to mw_php to clarify its role. It is now strictly a private Lua -> PHP interface function table. * Protect mw.setup() against multiple invocation. * Fixed a bug in Scribunto_LuaStandaloneInterpreter::receiveMessage(): large packets caused fread() to return with less than the requested amount of data, which previously caused an exception. It's necessary to check for EOF and to repeat the read to get all data. The receive function on the Lua side does not suffer from this problem. * In the standalone engine, fixed a bug in the interpretation of null return values from PHP callbacks. This should return no values to Lua. * Updated the Lua unit tests to account for the fact that functions are now forced to return strings. * Updated the getfenv and setfenv tests to account for the extra stack level introduced by mw.executeFunction(). Change-Id: If8fdecdfc91ebe7bd4b1dae8489ccbdeb6bbf5ce
306 lines
5.1 KiB
Plaintext
306 lines
5.1 KiB
Plaintext
!! article
|
|
Module:Test
|
|
!! text
|
|
local p = {}
|
|
|
|
local isoTestData = ''
|
|
|
|
require('bit')
|
|
|
|
function p.tooFewArgs()
|
|
require()
|
|
end
|
|
|
|
function p.getAllArgs( frame )
|
|
local buf = {}
|
|
local names = {}
|
|
local values = {}
|
|
for name, value in frame:argumentPairs() do
|
|
table.insert(names, name)
|
|
values[name] = value
|
|
end
|
|
table.sort(names, function (a, b) return tostring(a) < tostring(b) end)
|
|
for index, name in ipairs(names) do
|
|
if #buf ~= 0 then
|
|
table.insert( buf, ', ' )
|
|
end
|
|
table.insert( buf, name .. '=' .. values[name] )
|
|
end
|
|
return table.concat( buf )
|
|
end
|
|
|
|
function p.getArg( frame )
|
|
local name = frame.args[1]
|
|
return frame:getArgument( name ):expand()
|
|
end
|
|
|
|
function p.getArgLength( frame )
|
|
local name = frame.args[1]
|
|
return #(frame.args[name])
|
|
end
|
|
|
|
function p.getArgType( frame )
|
|
local name = frame.args[1]
|
|
return type( frame.args[name] )
|
|
end
|
|
|
|
function p.hello()
|
|
return 'hello'
|
|
end
|
|
|
|
function p.emptyTable()
|
|
return {}
|
|
end
|
|
|
|
function p.import()
|
|
return require('Module:Test2').countBeans()
|
|
end
|
|
|
|
function p.bitop()
|
|
return bit.bor(1, bit.bor(2, bit.bor(4, 8)))
|
|
end
|
|
|
|
function p.isolationTestUpvalue( frame )
|
|
isoTestData = isoTestData .. frame.args[1]
|
|
return isoTestData
|
|
end
|
|
|
|
function p.isolationTestGlobal( frame )
|
|
if isoTestDataGlobal == nil then
|
|
isoTestDataGlobal = ''
|
|
end
|
|
isoTestDataGlobal = isoTestDataGlobal .. frame.args[1]
|
|
return isoTestDataGlobal
|
|
end
|
|
|
|
function p.getParentArgs( frame )
|
|
return p.getAllArgs( frame:getParent() )
|
|
end
|
|
|
|
function p.testExpandTemplate( frame )
|
|
return frame:expandTemplate{
|
|
title = 'Scribunto_all_args',
|
|
args = { x = 1, y = 2, z = '|||' }
|
|
}
|
|
end
|
|
|
|
function p.testNewTemplateParserValue( frame )
|
|
return
|
|
frame:newTemplateParserValue{
|
|
title = 'Scribunto_all_args',
|
|
args = { x = 1, y = 2, z = 'blah' }
|
|
} : expand()
|
|
end
|
|
|
|
function p.testPreprocess( frame )
|
|
return frame:preprocess( '{{Scribunto_all_args|{{{1}}}}}|x=y' )
|
|
end
|
|
|
|
function p.testNewParserValue( frame )
|
|
return frame:newParserValue( '{{Scribunto_all_args|{{{1}}}}}|x=y' ):expand()
|
|
end
|
|
|
|
return p
|
|
!! endarticle
|
|
|
|
|
|
!! article
|
|
Module:Test2
|
|
!! text
|
|
return {
|
|
countBeans = function ()
|
|
return 3
|
|
end
|
|
}
|
|
!! endarticle
|
|
|
|
|
|
!! article
|
|
Module:Parse error
|
|
!! text
|
|
{{{{
|
|
!! endarticle
|
|
|
|
!! article
|
|
Template:Scribunto_all_args
|
|
!! text
|
|
{{#invoke:test|getParentArgs}}
|
|
!! endarticle
|
|
|
|
!! test
|
|
Scribunto: no such module
|
|
!! input
|
|
{{#invoke:foo|bar}}
|
|
!! result
|
|
<p><strong class="error"><span class="scribunto-error" id="mw-scribunto-error-0">Script error<!--Script error: No such module--></span></strong>
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: no such function
|
|
!! input
|
|
{{#invoke:test|blah}}
|
|
!! result
|
|
<p><strong class="error"><span class="scribunto-error" id="mw-scribunto-error-0">Script error<!--Script error: The function you specified did not exist.--></span></strong>
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: parse error
|
|
!! input
|
|
{{#invoke:Parse error|blah}}
|
|
!! result
|
|
<p><strong class="error"><span class="scribunto-error" id="mw-scribunto-error-0">Script error<!--Lua error in Module:Parse_error at line 1: unexpected symbol near '{'--></span></strong>
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: hello world
|
|
!! input
|
|
{{#invoke:test|hello}}
|
|
!! result
|
|
<p>hello
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: getAllArgs
|
|
!! input
|
|
{{#invoke:test|getAllArgs|x|y|z|a=1|b=2|c=3}}
|
|
!! result
|
|
<p>1=x, 2=y, 3=z, a=1, b=2, c=3
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: named numeric parameters
|
|
!! input
|
|
{{#invoke:test|getArg|2|a|2=b}}
|
|
{{#invoke:test|getArg|2|2=a|b}}
|
|
!! result
|
|
<p>b
|
|
b
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: template-style argument trimming
|
|
!! input
|
|
{{#invoke:test|getArgLength|2| x }}
|
|
{{#invoke:test|getArgLength|2|2= x }}
|
|
!! result
|
|
<p>3
|
|
1
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: missing argument
|
|
!! input
|
|
{{#invoke:test|getArgType|2}}
|
|
{{#invoke:test|getArgType|blah}}
|
|
!! result
|
|
<p>nil
|
|
nil
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: parent frame access
|
|
!! input
|
|
{{Scribunto_all_args|x|y|z|a = 1|b = 2|c = 3}}
|
|
!! result
|
|
<p>1=x, 2=y, 3=z, a=1, b=2, c=3
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: expandTemplate
|
|
!! input
|
|
{{#invoke:test|testExpandTemplate}}
|
|
!! result
|
|
<p>x=1, y=2, z=|||
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: newTemplateParserValue
|
|
!! input
|
|
{{#invoke:test|testNewTemplateParserValue}}
|
|
!! result
|
|
<p>x=1, y=2, z=blah
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: preprocess
|
|
!! input
|
|
{{#invoke:test|testPreprocess|foo}}
|
|
!! result
|
|
<p>1=foo|x=y
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: newParserValue
|
|
!! input
|
|
{{#invoke:test|testNewParserValue|foo}}
|
|
!! result
|
|
<p>1=foo|x=y
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: table return
|
|
!! input
|
|
{{#invoke:test|emptyTable}}
|
|
!! result
|
|
<p>table
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: require
|
|
!! input
|
|
{{#invoke:test|import}}
|
|
!! result
|
|
<p>3
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: access to a module imported at the chunk level
|
|
!! input
|
|
{{#invoke:test|bitop}}
|
|
!! result
|
|
<p>15
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: invoke instance upvalue isolation
|
|
!! input
|
|
{{#invoke:test|isolationTestUpvalue|1}}
|
|
{{#invoke:test|isolationTestUpvalue|2}}
|
|
{{#invoke:test|isolationTestUpvalue|3}}
|
|
!! result
|
|
<p>1
|
|
2
|
|
3
|
|
</p>
|
|
!! end
|
|
|
|
!! test
|
|
Scribunto: invoke instance global isolation
|
|
!! input
|
|
{{#invoke:test|isolationTestGlobal|1}}
|
|
{{#invoke:test|isolationTestGlobal|2}}
|
|
{{#invoke:test|isolationTestGlobal|3}}
|
|
!! result
|
|
<p>1
|
|
2
|
|
3
|
|
</p>
|
|
!! end
|
|
|